Giriş
İşaretçi, harita üzerinde bir konumu tanımlar. Varsayılan olarak, bir işaretçi
standart bir resimdir. İşaretçiler özel resimler gösterebilir. Bu durumda
bunlara genellikle "simgeler" denir. İşaretçiler ve simgeler belirli tür nesnelerdir
Marker
. İşaretçinin oluşturucusunda veya
işaretçide setIcon()
aranıyor. hakkında daha fazla bilgi
işaretçi resmini özelleştirme.
İşaretçiler, genel anlamda bir tür yer paylaşımıdır. Diğer bilgiler için daha fazla bilgi görmek için Haritada çizim.
İşaretçiler etkileşimli olacak şekilde tasarlanmıştır. Örneğin, varsayılan olarak
'click'
etkinlik alır, böylece şuna bir etkinlik işleyici ekleyebilirsiniz:
şunu getir
bilgi penceresi
özel bilgiler görüntüler. Kullanıcıların, bir işaretçiyi
draggable
özelliğini
true
. Sürüklenebilir işaretçiler hakkında daha fazla bilgi için bkz.
burada bulabilirsiniz.
İşaretçi ekleyin
İlgili içeriği oluşturmak için kullanılan
google.maps.Marker
oluşturucu tek bir Marker options
nesnesi alır
değişmez, işaretçinin başlangıç özelliklerini belirtir.
Aşağıdaki alanlar özellikle önemlidir ve genellikle bir işaretçi oluşturun:
-
position
(zorunlu), birLatLng
, işaretçinin ilk konumunu tanımlıyor. Bir birLatLng
almanın yolu Coğrafi kodlama hizmeti. -
map
(isteğe bağlı), hangiMap
öğesinin uygulanacağını belirtir işaretçiyi yerleştirin. İnşaat sırasında haritayı belirtmezseniz veya üzerinde görüntülenmeyen bir işaretleyici olduğu için tıklayın. İşaretçiyi daha sonrasetMap()
yöntemini çağırın.
Aşağıdaki örnekte, Uluru'daki bir haritaya basit bir işaretçi ekler. merkezi:
TypeScript
function initMap(): void { const myLatLng = { lat: -25.363, lng: 131.044 }; const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 4, center: myLatLng, } ); new google.maps.Marker({ position: myLatLng, map, title: "Hello World!", }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
function initMap() { const myLatLng = { lat: -25.363, lng: 131.044 }; const map = new google.maps.Map(document.getElementById("map"), { zoom: 4, center: myLatLng, }); new google.maps.Marker({ position: myLatLng, map, title: "Hello World!", }); } window.initMap = initMap;
Örneği Deneyin
Yukarıdaki örnekte, işaretçi harita üzerinde
İşaretçi seçeneklerinde map
özelliğini kullanarak.
Alternatif olarak,
aşağıdaki örnekte gösterildiği gibi, işaretçinin setMap()
yöntemi:
var myLatlng = new google.maps.LatLng(-25.363882,131.044922); var mapOptions = { zoom: 4, center: myLatlng } var map = new google.maps.Map(document.getElementById("map"), mapOptions); var marker = new google.maps.Marker({ position: myLatlng, title:"Hello World!" }); // To add the marker to the map, call setMap(); marker.setMap(map);
İşaretçinin title
bir ipucu olarak görünür.
Herhangi bir Marker options
testini geçmek istemiyorsanız
işaretçisinin oluşturucusu yerine boş bir nesneyi {}
kurucunun son bağımsız değişkenidir.
İşaretçiyi kaldırma
Bir işaretçiyi haritadan kaldırmak için setMap()
yöntemini çağırın
bağımsız değişken olarak null
iletiliyor.
marker.setMap(null);
Yukarıdaki yöntemin işaretçiyi silmediğini unutmayın. Araç,
haritadan kaldırın. Bunun yerine işaretçiyi silmek isterseniz
önce haritadan kaldırıp işaretçinin kendisini
null
Bir dizi işaretçiyi yönetmek istiyorsanız işaretlenecek bir dizi oluşturmanız gerekir
tıklayın. Bu diziyi kullanarak setMap()
öğesini çağırabilirsiniz.
işaretleyicileri kaldırmanız gerektiğinde dizideki her bir işaretçiye sırayla ekleyin. Siz
işaretçileri haritadan kaldırıp
dizide, tüm öğeleri kaldıran length
parametresinden 0
değerine
başvurulara da göz atmanızı öneririm.
İşaretçi resmini özelleştirme
İşaretçilerin görünümünü özelleştirmek için image file veya varsayılan yerine görüntülenecek vektör tabanlı simge Google Haritalar raptiye simgesi. Metinleri şununla ekleyebilirsiniz: işaretçi etiketini kullanın ve tıklanabilir bölgeleri tanımlamak için karmaşık simgeler ve işaretçilerin yığın sırasını ayarlayın.
Resim simgeleri içeren işaretçiler
En temel durumda, simge
varsayılan Google Haritalar raptiye simgesi. Böyle bir simgeyi belirtmek için,
işaretçinin icon
özelliğini bir resmin URL'sine ekleyin. Maps JavaScript API, simgeyi otomatik olarak boyutlandırır.
TypeScript
// This example adds a marker to indicate the position of Bondi Beach in Sydney, // Australia. function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 4, center: { lat: -33, lng: 151 }, } ); const image = "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png"; const beachMarker = new google.maps.Marker({ position: { lat: -33.89, lng: 151.274 }, map, icon: image, }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// This example adds a marker to indicate the position of Bondi Beach in Sydney, // Australia. function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 4, center: { lat: -33, lng: 151 }, }); const image = "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png"; const beachMarker = new google.maps.Marker({ position: { lat: -33.89, lng: 151.274 }, map, icon: image, }); } window.initMap = initMap;
Örneği Deneyin
Vektör tabanlı simgeler içeren işaretçiler
Şunun görsel görünümünü tanımlamak için özel SVG vektör yollarını kullanabilirsiniz:
kullanabilirsiniz. Bunu yapmak için bir Symbol
nesnesi değişmez değerini (şununla birlikte) iletin:
işaretçinin icon
özelliğine gitmek için istediğiniz yolu seçin. Hedeflerinize göre bir
kullanılan özel yol
SVG yol gösterimini veya
google.maps.SymbolPath olarak değiştirin. İşaretçinin şunları yapması için anchor
özelliği gereklidir:
yakınlaştırma seviyesi değiştiğinde doğru bir şekilde oluşturulmasını sağlayın. Daha fazla bilgi:
vektör tabanlı simgeler oluşturmak için sembolleri kullanma
kullanın.
TypeScript
// This example uses SVG path notation to add a vector-based symbol // as the icon for a marker. The resulting icon is a marker-shaped // symbol with a blue fill and no border. function initMap(): void { const center = new google.maps.LatLng(-33.712451, 150.311823); const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 9, center: center, } ); const svgMarker = { path: "M-1.547 12l6.563-6.609-1.406-1.406-5.156 5.203-2.063-2.109-1.406 1.406zM0 0q2.906 0 4.945 2.039t2.039 4.945q0 1.453-0.727 3.328t-1.758 3.516-2.039 3.070-1.711 2.273l-0.75 0.797q-0.281-0.328-0.75-0.867t-1.688-2.156-2.133-3.141-1.664-3.445-0.75-3.375q0-2.906 2.039-4.945t4.945-2.039z", fillColor: "blue", fillOpacity: 0.6, strokeWeight: 0, rotation: 0, scale: 2, anchor: new google.maps.Point(0, 20), }; new google.maps.Marker({ position: map.getCenter(), icon: svgMarker, map: map, }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// This example uses SVG path notation to add a vector-based symbol // as the icon for a marker. The resulting icon is a marker-shaped // symbol with a blue fill and no border. function initMap() { const center = new google.maps.LatLng(-33.712451, 150.311823); const map = new google.maps.Map(document.getElementById("map"), { zoom: 9, center: center, }); const svgMarker = { path: "M-1.547 12l6.563-6.609-1.406-1.406-5.156 5.203-2.063-2.109-1.406 1.406zM0 0q2.906 0 4.945 2.039t2.039 4.945q0 1.453-0.727 3.328t-1.758 3.516-2.039 3.070-1.711 2.273l-0.75 0.797q-0.281-0.328-0.75-0.867t-1.688-2.156-2.133-3.141-1.664-3.445-0.75-3.375q0-2.906 2.039-4.945t4.945-2.039z", fillColor: "blue", fillOpacity: 0.6, strokeWeight: 0, rotation: 0, scale: 2, anchor: new google.maps.Point(0, 20), }; new google.maps.Marker({ position: map.getCenter(), icon: svgMarker, map: map, }); } window.initMap = initMap;
Örneği Deneyin
İşaretçi etiketleri
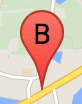
İşaretçi etiketi, işaretçi içinde görünen bir harf veya rakamdır. İlgili içeriği oluşturmak için kullanılan
Bu bölümde, "B" harfini içeren bir işaretçi etiketi görüntülenir
tıklayın. Bir işaretçi etiketini dize veya
MarkerLabel
dize ve diğer etiket özelliklerini içeren nesnedir.
İşaretçi oluştururken label
özelliğini
"the"
MarkerOptions
nesnesini tanımlayın. Alternatif olarak, şu numaradan setLabel()
numaralı telefonu arayabilirsiniz:
İşaretçi
nesnesini de kullanabilirsiniz.
Aşağıdaki örnekte, kullanıcı harita:
TypeScript
// In the following example, markers appear when the user clicks on the map. // Each marker is labeled with a single alphabetical character. const labels = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; let labelIndex = 0; function initMap(): void { const bangalore = { lat: 12.97, lng: 77.59 }; const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 12, center: bangalore, } ); // This event listener calls addMarker() when the map is clicked. google.maps.event.addListener(map, "click", (event) => { addMarker(event.latLng, map); }); // Add a marker at the center of the map. addMarker(bangalore, map); } // Adds a marker to the map. function addMarker(location: google.maps.LatLngLiteral, map: google.maps.Map) { // Add the marker at the clicked location, and add the next-available label // from the array of alphabetical characters. new google.maps.Marker({ position: location, label: labels[labelIndex++ % labels.length], map: map, }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// In the following example, markers appear when the user clicks on the map. // Each marker is labeled with a single alphabetical character. const labels = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; let labelIndex = 0; function initMap() { const bangalore = { lat: 12.97, lng: 77.59 }; const map = new google.maps.Map(document.getElementById("map"), { zoom: 12, center: bangalore, }); // This event listener calls addMarker() when the map is clicked. google.maps.event.addListener(map, "click", (event) => { addMarker(event.latLng, map); }); // Add a marker at the center of the map. addMarker(bangalore, map); } // Adds a marker to the map. function addMarker(location, map) { // Add the marker at the clicked location, and add the next-available label // from the array of alphabetical characters. new google.maps.Marker({ position: location, label: labels[labelIndex++ % labels.length], map: map, }); } window.initMap = initMap;
Örneği Deneyin
Karmaşık simgeler
Tıklanabilir bölgeleri belirtmek için karmaşık şekiller belirtebilirsiniz ve
simgelerin diğer yer paylaşımlarına göre nasıl görüneceğini belirtmek
"yığın sırası"). Bu şekilde belirtilen simgeler,
icon
.
özellikleri
Icon
Icon
nesneler bir görüntüyü tanımlar. Ayrıca, anahtar/değer çiftlerinin size
simgesini, origin
(istediğiniz resim reklam
büyük bir resmin çizimi) ve
Simgenin hotspot'unun bulunduğu yerde anchor
(kaynağa göre) gösterir.
Özel etiket içeren bir etiket kullanıyorsanız
kullanıyorsanız etiketi
labelOrigin
mülk
Icon
nesnesini tanımlayın.
TypeScript
// The following example creates complex markers to indicate beaches near // Sydney, NSW, Australia. Note that the anchor is set to (0,32) to correspond // to the base of the flagpole. function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 10, center: { lat: -33.9, lng: 151.2 }, } ); setMarkers(map); } // Data for the markers consisting of a name, a LatLng and a zIndex for the // order in which these markers should display on top of each other. const beaches: [string, number, number, number][] = [ ["Bondi Beach", -33.890542, 151.274856, 4], ["Coogee Beach", -33.923036, 151.259052, 5], ["Cronulla Beach", -34.028249, 151.157507, 3], ["Manly Beach", -33.80010128657071, 151.28747820854187, 2], ["Maroubra Beach", -33.950198, 151.259302, 1], ]; function setMarkers(map: google.maps.Map) { // Adds markers to the map. // Marker sizes are expressed as a Size of X,Y where the origin of the image // (0,0) is located in the top left of the image. // Origins, anchor positions and coordinates of the marker increase in the X // direction to the right and in the Y direction down. const image = { url: "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png", // This marker is 20 pixels wide by 32 pixels high. size: new google.maps.Size(20, 32), // The origin for this image is (0, 0). origin: new google.maps.Point(0, 0), // The anchor for this image is the base of the flagpole at (0, 32). anchor: new google.maps.Point(0, 32), }; // Shapes define the clickable region of the icon. The type defines an HTML // <area> element 'poly' which traces out a polygon as a series of X,Y points. // The final coordinate closes the poly by connecting to the first coordinate. const shape = { coords: [1, 1, 1, 20, 18, 20, 18, 1], type: "poly", }; for (let i = 0; i < beaches.length; i++) { const beach = beaches[i]; new google.maps.Marker({ position: { lat: beach[1], lng: beach[2] }, map, icon: image, shape: shape, title: beach[0], zIndex: beach[3], }); } } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// The following example creates complex markers to indicate beaches near // Sydney, NSW, Australia. Note that the anchor is set to (0,32) to correspond // to the base of the flagpole. function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 10, center: { lat: -33.9, lng: 151.2 }, }); setMarkers(map); } // Data for the markers consisting of a name, a LatLng and a zIndex for the // order in which these markers should display on top of each other. const beaches = [ ["Bondi Beach", -33.890542, 151.274856, 4], ["Coogee Beach", -33.923036, 151.259052, 5], ["Cronulla Beach", -34.028249, 151.157507, 3], ["Manly Beach", -33.80010128657071, 151.28747820854187, 2], ["Maroubra Beach", -33.950198, 151.259302, 1], ]; function setMarkers(map) { // Adds markers to the map. // Marker sizes are expressed as a Size of X,Y where the origin of the image // (0,0) is located in the top left of the image. // Origins, anchor positions and coordinates of the marker increase in the X // direction to the right and in the Y direction down. const image = { url: "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png", // This marker is 20 pixels wide by 32 pixels high. size: new google.maps.Size(20, 32), // The origin for this image is (0, 0). origin: new google.maps.Point(0, 0), // The anchor for this image is the base of the flagpole at (0, 32). anchor: new google.maps.Point(0, 32), }; // Shapes define the clickable region of the icon. The type defines an HTML // <area> element 'poly' which traces out a polygon as a series of X,Y points. // The final coordinate closes the poly by connecting to the first coordinate. const shape = { coords: [1, 1, 1, 20, 18, 20, 18, 1], type: "poly", }; for (let i = 0; i < beaches.length; i++) { const beach = beaches[i]; new google.maps.Marker({ position: { lat: beach[1], lng: beach[2] }, map, icon: image, shape: shape, title: beach[0], zIndex: beach[3], }); } } window.initMap = initMap;
Örneği Deneyin
MarkerImage
nesne Icon
türüne dönüştürülüyor
Maps JavaScript API'nin 3.10 sürümüne kadar, karmaşık simgeler
MarkerImage
nesne olarak tanımlandı. İlgili içeriği oluşturmak için kullanılan
Icon
nesne değişmez değeri sürüm 3.10'da eklendi ve nesne değişmez değeri
3.11'den itibaren MarkerImage
.
Icon
nesne değişmez değeri,
MarkerImage
, bir dosyayı kolayca dönüştürmenizi sağlar.
Bir Icon
cihazına MarkerImage
oluşturucusu, önceki parametreleri {}
içine alır ve
her parametrenin adını ekleyin. Örneğin:
var image = new google.maps.MarkerImage( place.icon, new google.maps.Size(71, 71), new google.maps.Point(0, 0), new google.maps.Point(17, 34), new google.maps.Size(25, 25));
şu olur:
var image = { url: place.icon, size: new google.maps.Size(71, 71), origin: new google.maps.Point(0, 0), anchor: new google.maps.Point(17, 34), scaledSize: new google.maps.Size(25, 25) };
Optimize işaretçileri
Optimizasyon, çok sayıda işaretçiyi tek bir işaretçi olarak oluşturarak performansı artırır. statik öğedir. Bu, çok sayıda işaretçinin bulunduğu durumlarda gereklidir. Varsayılan olarak, Google Haritalar JavaScript API'si bir işaretçinin optimize edilip edilmeyeceğini belirtir. Projede çok sayıda Maps JavaScript API, istediğiniz her yerde işaretçileri otomatik olarak kullanır. İşaretçilerin hepsi optimize edilemez; bazı bazı durumlarda, Maps JavaScript API'nin Optimizasyonu olmayan işaretçiler. Animasyonlar için optimize edilmiş oluşturmayı devre dışı bırak GIF'ler veya PNG'ler ya da her bir işaretçinin ayrı bir DOM olarak oluşturulması gerektiğinde öğesine dokunun. Aşağıdaki örnekte, optimize edilmiş bir işaretçinin oluşturulması gösterilmektedir:
var marker = new google.maps.Marker({ position: myLatlng, title:"Hello World!", optimized: true });
İşaretçileri erişilebilir hale getirme
Bir tıklama işleyici etkinliği ekleyerek bir işaretçiyi erişilebilir hale getirebilirsiniz.
optimized
, false
olarak ayarlanıyor. Tıklama işleyici
Bu, işaretçiye
klavyeyle gezinme, ekran okuyucular gibi pek çok özelliği kullanabilirsiniz. Şunu kullanın:
title
seçeneği.
Aşağıdaki örnekte, sekme açıldığında ilk işaretçi basıldı; ardından, işaretçiler arasında geçiş yapmak için ok tuşlarını kullanabilirsiniz. Bas sekmesini tekrar tıklayın. bilgi penceresi varsa, işaretçiyi tıklayarak veya İşaretçi seçildiğinde Enter tuşuna veya boşluk çubuğuna basılması. bilgi penceresi kapanırsa odak ilgili işaretçiye geri döner.
TypeScript
// The following example creates five accessible and // focusable markers. function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 12, center: { lat: 34.84555, lng: -111.8035 }, } ); // Set LatLng and title text for the markers. The first marker (Boynton Pass) // receives the initial focus when tab is pressed. Use arrow keys to // move between markers; press tab again to cycle through the map controls. const tourStops: [google.maps.LatLngLiteral, string][] = [ [{ lat: 34.8791806, lng: -111.8265049 }, "Boynton Pass"], [{ lat: 34.8559195, lng: -111.7988186 }, "Airport Mesa"], [{ lat: 34.832149, lng: -111.7695277 }, "Chapel of the Holy Cross"], [{ lat: 34.823736, lng: -111.8001857 }, "Red Rock Crossing"], [{ lat: 34.800326, lng: -111.7665047 }, "Bell Rock"], ]; // Create an info window to share between markers. const infoWindow = new google.maps.InfoWindow(); // Create the markers. tourStops.forEach(([position, title], i) => { const marker = new google.maps.Marker({ position, map, title: `${i + 1}. ${title}`, label: `${i + 1}`, optimized: false, }); // Add a click listener for each marker, and set up the info window. marker.addListener("click", () => { infoWindow.close(); infoWindow.setContent(marker.getTitle()); infoWindow.open(marker.getMap(), marker); }); }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// The following example creates five accessible and // focusable markers. function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 12, center: { lat: 34.84555, lng: -111.8035 }, }); // Set LatLng and title text for the markers. The first marker (Boynton Pass) // receives the initial focus when tab is pressed. Use arrow keys to // move between markers; press tab again to cycle through the map controls. const tourStops = [ [{ lat: 34.8791806, lng: -111.8265049 }, "Boynton Pass"], [{ lat: 34.8559195, lng: -111.7988186 }, "Airport Mesa"], [{ lat: 34.832149, lng: -111.7695277 }, "Chapel of the Holy Cross"], [{ lat: 34.823736, lng: -111.8001857 }, "Red Rock Crossing"], [{ lat: 34.800326, lng: -111.7665047 }, "Bell Rock"], ]; // Create an info window to share between markers. const infoWindow = new google.maps.InfoWindow(); // Create the markers. tourStops.forEach(([position, title], i) => { const marker = new google.maps.Marker({ position, map, title: `${i + 1}. ${title}`, label: `${i + 1}`, optimized: false, }); // Add a click listener for each marker, and set up the info window. marker.addListener("click", () => { infoWindow.close(); infoWindow.setContent(marker.getTitle()); infoWindow.open(marker.getMap(), marker); }); }); } window.initMap = initMap;
Örneği Deneyin
İşaretçiyi canlandırma
İşaretçileri animasyonla gösterip çeşitli öğelerde dinamik hareket gösterebilirsiniz
farklı koşullar. Bir işaretçinin animasyon yöntemini belirlemek için
İşaretçinin animation
özelliği, türü
google.maps.Animation
. Aşağıdakiler
Animation
değerleri desteklenir:
-
DROP
, işaretçinin ilk kez yerleştirildiğinde haritayı son konumuna getirir. Animasyon işaretçi durduğunda durur veanimation
,null
öğesine geri dön. Bu animasyon türü genellikleMarker
öğesi oluşturuldu. -
BOUNCE
, işaretçinin yerinde atlaması gerektiğini belirtir. CEVAP geri dönen işaretçi, kendisi sona erene kadaranimation
özelliği açıkçanull
olarak ayarlandı.
Şunu tıklayarak mevcut bir işaretçide bir animasyon başlatabilirsiniz:
Marker
nesnesinde setAnimation()
.
TypeScript
// The following example creates a marker in Stockholm, Sweden using a DROP // animation. Clicking on the marker will toggle the animation between a BOUNCE // animation and no animation. let marker: google.maps.Marker; function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 13, center: { lat: 59.325, lng: 18.07 }, } ); marker = new google.maps.Marker({ map, draggable: true, animation: google.maps.Animation.DROP, position: { lat: 59.327, lng: 18.067 }, }); marker.addListener("click", toggleBounce); } function toggleBounce() { if (marker.getAnimation() !== null) { marker.setAnimation(null); } else { marker.setAnimation(google.maps.Animation.BOUNCE); } } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// The following example creates a marker in Stockholm, Sweden using a DROP // animation. Clicking on the marker will toggle the animation between a BOUNCE // animation and no animation. let marker; function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 13, center: { lat: 59.325, lng: 18.07 }, }); marker = new google.maps.Marker({ map, draggable: true, animation: google.maps.Animation.DROP, position: { lat: 59.327, lng: 18.067 }, }); marker.addListener("click", toggleBounce); } function toggleBounce() { if (marker.getAnimation() !== null) { marker.setAnimation(null); } else { marker.setAnimation(google.maps.Animation.BOUNCE); } } window.initMap = initMap;
Örneği Deneyin
Çok sayıda işaretçiniz varsa bunların tümünü haritaya bırakmak istemeyebilirsiniz
sahip olabilirsiniz. Depolama alanınızı yerleştirmek için setTimeout()
işaretçiler animasyonları aşağıda gösterilene benzer bir kalıp kullanarak oluşturabilirsiniz:
function drop() { for (var i =0; i < markerArray.length; i++) { setTimeout(function() { addMarkerMethod(); }, i * 200); } }
İşaretçiyi sürüklenebilir hale getirme
Kullanıcıların bir işaretçiyi haritada farklı bir konuma sürüklemesine izin vermek için
İşaretçi seçeneklerinde draggable
- true
arası.
var myLatlng = new google.maps.LatLng(-25.363882,131.044922); var mapOptions = { zoom: 4, center: myLatlng } var map = new google.maps.Map(document.getElementById("map"), mapOptions); // Place a draggable marker on the map var marker = new google.maps.Marker({ position: myLatlng, map: map, draggable:true, title:"Drag me!" });
Daha Fazla İşaretçi Özelleştirme
Tamamen özelleştirilmiş bir işaretçi için özelleştirilmiş pop-up örneği.
İşaretçi sınıfı, işaretçi kümeleme ve yönetimi ile yer paylaşımı özelleştirmesiyle ilgili daha fazla uzantı için bkz. açık kaynak kitaplıklar.