เครื่องหมายขั้นสูงใช้คลาส 2 คลาสในการกําหนดเครื่องหมาย ได้แก่ คลาส GMSAdvancedMarker
ซึ่งให้ความสามารถเริ่มต้นของเครื่องหมาย และคลาส GMSPinImageOptions
ซึ่งมีตัวเลือกสําหรับการปรับแต่งเพิ่มเติม หน้านี้จะแสดงวิธีปรับแต่งเครื่องหมายดังต่อไปนี้
- เปลี่ยนสีพื้นหลัง
- เปลี่ยนสีเส้นขอบ
- เปลี่ยนสีสัญลักษณ์
- เปลี่ยนข้อความแบบอักขระ
- รองรับมุมมองและภาพเคลื่อนไหวที่กําหนดเองด้วยพร็อพเพอร์ตี้ iconView
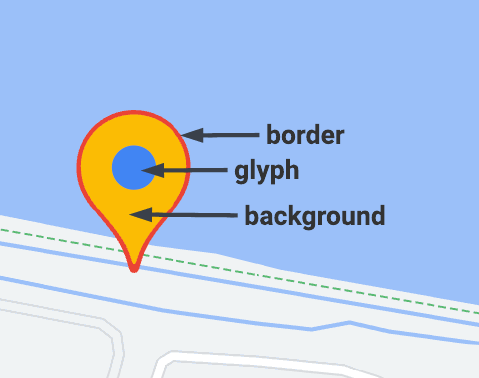
เปลี่ยนสีพื้นหลัง
ใช้ตัวเลือก GMSPinImageOptions.backgroundColor
เพื่อเปลี่ยนสีพื้นหลังของเครื่องหมาย
Swift
//... let options = GMSPinImageOptions() options.backgroundColor = .blue let pinImage = GMSPinImage(options: options) advancedMarker.icon = pinImage advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.backgroundColor = [UIColor blueColor]; GMSPinImage *pin = [GMSPinImage pinImageWithOptions:options]; customTextMarker.icon = pin; customTextMarker.map = mapView;
เปลี่ยนสีเส้นขอบ
ใช้ตัวเลือก
GMSPinImageOptions.borderColor
เพื่อเปลี่ยนสีพื้นหลังของตัวทำเครื่องหมาย
Swift
//... let options = GMSPinImageOptions() options.borderColor = .blue let pinImage = GMSPinImage(options: options) advancedMarker.icon = pinImage advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.backgroundColor = [UIColor blueColor]; GMSPinImage *pin = [GMSPinImage pinImageWithOptions:options]; advancedMarker.icon = pin; advancedMarker.map = mapView;
เปลี่ยนสีสัญลักษณ์
ใช้ GMSPinImageGlyph.glyphColor
เพื่อเปลี่ยนสีพื้นหลังของเครื่องหมาย
Swift
//... let options = GMSPinImageOptions() let glyph = GMSPinImageGlyph(glyphColor: .yellow) options.glyph = glyph let glyphColor = GMSPinImage(options: options) advancedMarker.icon = glyphColor advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.glyph = [[GMSPinImageGlyph alloc] initWithGlyphColor:[UIColor yellowColor]]; GMSPinImage *glyphColor = [GMSPinImage pinImageWithOptions:options]; advancedMarker.icon = glyphColor; advancedMarker.map = mapView;
เปลี่ยนข้อความแบบอักขระ
ใช้ GMSPinImageGlyph
เพื่อเปลี่ยนข้อความสัญลักษณ์ของหมุด
Swift
//... let options = GMSPinImageOptions() let glyph = GMSPinImageGlyph(text: "ABC", textColor: .white) options.glyph = glyph let pinImage = GMSPinImage(options: options) advancedMarker.icon = pinImage advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.glyph = [[GMSPinImageGlyph alloc] initWithText:@"ABC" textColor:[UIColor whiteColor]]; GMSPinImage *pin = [GMSPinImage pinImageWithOptions:options]; customTextMarker.icon = pin; customTextMarker.map = mapView;
รองรับมุมมองและภาพเคลื่อนไหวที่กําหนดเองด้วยพร็อพเพอร์ตี้ iconView
GMSAdvancedMarker
รองรับเครื่องหมายที่มี iconView
เช่นเดียวกับ GMSMarker
พร็อพเพอร์ตี้ iconView
รองรับภาพเคลื่อนไหวของพร็อพเพอร์ตี้ที่เคลื่อนไหวได้ทั้งหมดของ UIView
ยกเว้นเฟรมและศูนย์ และไม่รองรับเครื่องหมายที่มี iconViews
และ icons
แสดงบนแผนที่เดียวกัน
Swift
//... let advancedMarker = GMSAdvancedMarker(position: coordinate) advancedMarker.iconView = customView() advancedMarker.map = mapView func customView() -> UIView { // return your custom UIView. }
Objective-C
//... GMSAdvancedMarker *advancedMarker = [GMSAdvancedMarker markerWithPosition:kCoordinate]; advancedMarker.iconView = [self customView]; advancedMarker.map = self.mapView; - (UIView *)customView { // return custom view }
ข้อจำกัดของเลย์เอาต์
GMSAdvancedMarker
ไม่รองรับข้อจำกัดของเลย์เอาต์สำหรับ iconView
โดยตรง อย่างไรก็ตาม คุณสามารถกำหนดข้อจำกัดของเลย์เอาต์สำหรับองค์ประกอบอินเทอร์เฟซผู้ใช้ภายใน iconView
ได้ เมื่อสร้างมุมมองแล้ว คุณควรส่งออบเจ็กต์ frame
หรือ size
ไปยังเครื่องหมาย
Swift
//do not set advancedMarker.iconView.translatesAutoresizingMaskIntoConstraints = false let advancedMarker = GMSAdvancedMarker(position: coordinate) let customView = customView() //set frame customView.frame = CGRect(0, 0, width, height) advancedMarker.iconView = customView
Objective-C
//do not set advancedMarker.iconView.translatesAutoresizingMaskIntoConstraints = NO; GMSAdvancedMarker *advancedMarker = [GMSAdvancedMarker markerWithPosition:kCoordinate]; CustomView *customView = [self customView]; //set frame customView.frame = CGRectMake(0, 0, width, height); advancedMarker.iconView = customView;