Google Street View provides panoramic 360-degree views from designated roads throughout its coverage area.
This video shows how you can use the Street View service to give your users a real-world experience of an address on the map, providing them with meaningful context about their destination or any place they're interested in.
The coverage available through the Google Maps Android API v2 is the same as that for the Google Maps app on your Android device. You can read more about Street View and see the supported areas on an interactive map, at About Street View.
The StreetViewPanorama
class models the Street
View panorama in your application. Within your UI, a panorama is represented
by a StreetViewPanoramaFragment
or
StreetViewPanoramaView
object.
Code samples
The ApiDemos repository on GitHub includes samples that demonstrate the use of Street View.
Kotlin samples:
- StreetViewPanoramaBasicDemoActivity: The basics of using Street View
- StreetViewPanoramaEventsDemoActivity: Listening to events
- StreetViewPanoramaNavigationDemoActivity: Controlling Street View panoramas programmatically
- StreetViewPanoramaOptionsDemoActivity: Changing UI and gesture options
- StreetViewPanoramaViewDemoActivity: Using the
StreetViewPanoramaView
(instead of a Fragment) - SplitStreetViewPanoramaAndMapDemoActivity: Using an activity that displays a street view and a map
Java samples:
- StreetViewPanoramaBasicDemoActivity: The basics of using Street View
- StreetViewPanoramaEventsDemoActivity: Listening to events
- StreetViewPanoramaNavigationDemoActivity: Controlling Street View panoramas programmatically
- StreetViewPanoramaOptionsDemoActivity: Changing UI and gesture options
- StreetViewPanoramaViewDemoActivity: Using the
StreetViewPanoramaView
(instead of a Fragment) - SplitStreetViewPanoramaAndMapDemoActivity: Creating an activity that displays a street view and a map
Overview of Street View in the Maps SDK for Android
The Maps SDK for Android provides a Street View service for obtaining and manipulating the imagery used in Google Street View. Images are returned as panoramas.
Each Street View panorama is an image, or set of images, that provides a full 360-degree view from a single location. Images conform to the equirectangular (Plate Carrée) projection, which contains 360 degrees of horizontal view (a full wrap-around) and 180 degrees of vertical view (from straight up to straight down). The resulting 360-degree panorama defines a projection on a sphere with the image wrapped to the two-dimensional surface of that sphere.
StreetViewPanorama
provides a viewer that renders
the panorama as a sphere with a camera at its center. You can manipulate the
StreetViewPanoramaCamera
to control the zoom and the orientation (tilt and bearing) of the camera.
Get started
Set up a project
Follow the getting-started guide to set up a Maps SDK for Android project.
Check Street View panorama availability before adding a panorama
The Google Play services SDK client library includes a few Street View samples which you can import into your project and use as a basis for development. See the introduction for guidelines on importing the samples.
The Maps SDK for Android Utility Library
is an open source library of classes that are useful for a range of
applications. Included in the GitHub repository is the
Street View metadata utility.
This utility checks whether a location is supported by
Street View. You can avoid errors when adding a
Street View panorama to an Android app by calling this
metadata utility and only adding a Street View panorama
if the response is OK
.
Use the API
Follow the instructions below to add a Street View panorama to an Android fragment. That's the simplest way to add Street View to your application. Then read more about fragments, views, and customizing the panorama.
Add a Street View panorama
Follow the steps below to add a Street View panorama like this one:
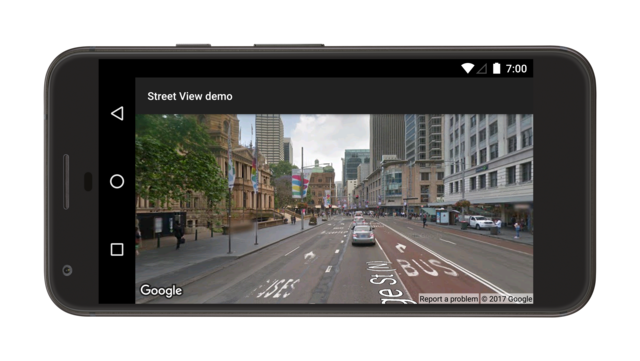
In summary:
- Add a Fragment object to the Activity
that will handle the Street View panorama. The easiest way to do this is to
add a
<fragment>
element to the layout file for theActivity
. - Implement the
OnStreetViewPanoramaReadyCallback
interface and use theonStreetViewPanoramaReady(StreetViewPanorama)
callback method to get a handle to theStreetViewPanorama
object. - Call
getStreetViewPanoramaAsync()
on the fragment to register the callback.
Below is more detail about each step.
Add a fragment
Add a <fragment>
element to the activity's layout file to define a
Fragment object. In this element, set the class
attribute to com.google.android.gms.maps.StreetViewPanoramaFragment
(or
SupportStreetViewPanoramaFragment
).
Here is an example of a fragment in a layout file:
<fragment android:name="com.google.android.gms.maps.StreetViewPanoramaFragment" android:id="@+id/streetviewpanorama" android:layout_width="match_parent" android:layout_height="match_parent"/>
Add Street View code
To work with the Street View panorama inside your app, you'll need to implement
the OnStreetViewPanoramaReadyCallback
interface and set an instance of the callback on a
StreetViewPanoramaFragment
or
StreetViewPanoramaView
object. This tutorial uses a
StreetViewPanoramaFragment
, because that's the simplest way to add Street View
to your app. The first step is to implement the callback interface:
Kotlin
class StreetViewActivity : AppCompatActivity(), OnStreetViewPanoramaReadyCallback { // ... }
Java
class StreetViewActivity extends AppCompatActivity implements OnStreetViewPanoramaReadyCallback { // ... }
In your Activity
's onCreate()
method, set the layout file as the content view. For example, if the layout file
has the name main.xml
, use this code:
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_street_view) val streetViewPanoramaFragment = supportFragmentManager .findFragmentById(R.id.street_view_panorama) as SupportStreetViewPanoramaFragment streetViewPanoramaFragment.getStreetViewPanoramaAsync(this) }
Java
@Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_street_view); SupportStreetViewPanoramaFragment streetViewPanoramaFragment = (SupportStreetViewPanoramaFragment) getSupportFragmentManager() .findFragmentById(R.id.street_view_panorama); streetViewPanoramaFragment.getStreetViewPanoramaAsync(this); }
Get a handle to the fragment by calling
FragmentManager.findFragmentById()
,
passing it the resource ID of your <fragment>
element.
Notice that the resource ID R.id.streetviewpanorama
is added automatically to
the Android project when you build the layout file.
Then use getStreetViewPanoramaAsync()
to set the callback on the fragment.
Kotlin
val streetViewPanoramaFragment = supportFragmentManager .findFragmentById(R.id.street_view_panorama) as SupportStreetViewPanoramaFragment streetViewPanoramaFragment.getStreetViewPanoramaAsync(this)
Java
SupportStreetViewPanoramaFragment streetViewPanoramaFragment = (SupportStreetViewPanoramaFragment) getSupportFragmentManager() .findFragmentById(R.id.street_view_panorama); streetViewPanoramaFragment.getStreetViewPanoramaAsync(this);
Use the
onStreetViewPanoramaReady(StreetViewPanorama)
callback method to to retrieve a non-null instance of
StreetViewPanorama
, ready to be used.
Kotlin
override fun onStreetViewPanoramaReady(streetViewPanorama: StreetViewPanorama) { val sanFrancisco = LatLng(37.754130, -122.447129) streetViewPanorama.setPosition(sanFrancisco) }
Java
@Override public void onStreetViewPanoramaReady(StreetViewPanorama streetViewPanorama) { LatLng sanFrancisco = new LatLng(37.754130, -122.447129); streetViewPanorama.setPosition(sanFrancisco); }
More about configuring initial state
Unlike with a map, it's not possible to configure the initial state of the
Street View panorama via XML. However, you can configure
the panorama programmatically by passing in a
StreetViewPanoramaOptions
object
containing your specified options.
- If you are using a
StreetViewPanoramaFragment
, use theStreetViewPanoramaFragment.newInstance(StreetViewPanoramaOptions options)
static factory method to construct the fragment and pass in your custom configured options. - If you are using a
StreetViewPanoramaView
, use theStreetViewPanoramaView(Context, StreetViewPanoramaOptions)
constructor and pass in your custom configured options.
Kotlin
val sanFrancisco = LatLng(37.754130, -122.447129) val view = StreetViewPanoramaView( this, StreetViewPanoramaOptions().position(sanFrancisco) )
Java
LatLng sanFrancisco = new LatLng(37.754130, -122.447129); StreetViewPanoramaView view = new StreetViewPanoramaView(this, new StreetViewPanoramaOptions().position(sanFrancisco));
More about StreetViewPanoramaFragment
StreetViewPanoramaFragment
is a subclass of the
Android Fragment class, and allows you to place a Street View
panorama in an Android fragment. StreetViewPanoramaFragment
objects act as
containers for the panorama, and provide access to the StreetViewPanorama
object.
StreetViewPanoramaView
StreetViewPanoramaView
, a subclass of the Android
View
class, allows you to place a Street View
panorama in an Android View
. A View
represents a rectangular region of the
screen, and is a fundamental building block for Android applications and widgets.
Much like a StreetViewPanoramaFragment
, the StreetViewPanoramaView
acts as
a container for the panorama, exposing core functionality through the
StreetViewPanorama
object. Users of this class must forward all the activity
life cycle methods (such as onCreate()
, onDestroy()
, onResume()
, and
onPause())
to the corresponding methods in the StreetViewPanoramaView
class.
Customize the user-controlled functionality
By default, the following functionality is available to the user when viewing
the Street View panorama: panning, zooming, and traveling
to adjacent panoramas. You can enable and disable user-controlled gestures
through methods on StreetViewPanorama
. Programmatic
changes are still possible when the gesture is disabled.
Set the location of the panorama
To set the location of the Street View panorama, call
StreetViewPanorama.setPosition()
, passing a LatLng
.
You can also pass radius
and source
as optional parameters.
A radius is useful if you want to widen or
narrow the area in which Street View will look for a matching panorama. A radius
of 0 means that the panorama must be linked to exactly the specified LatLng
.
The default radius is 50 meters. If there is more than one panorama in the
matching area, the API will return the best match.
A source is useful if you want to restrict Street View to only look for panoramas which are outdoors. By default, Street View panoramas could be inside places such as museums, public buildings, cafes and businesses. Note that outdoor panoramas may not exist for the specified location.
Kotlin
val sanFrancisco = LatLng(37.754130, -122.447129) // Set position with LatLng only. streetViewPanorama.setPosition(sanFrancisco) // Set position with LatLng and radius. streetViewPanorama.setPosition(sanFrancisco, 20) // Set position with LatLng and source. streetViewPanorama.setPosition(sanFrancisco, StreetViewSource.OUTDOOR) // Set position with LaLng, radius and source. streetViewPanorama.setPosition(sanFrancisco, 20, StreetViewSource.OUTDOOR)
Java
LatLng sanFrancisco = new LatLng(37.754130, -122.447129); // Set position with LatLng only. streetViewPanorama.setPosition(sanFrancisco); // Set position with LatLng and radius. streetViewPanorama.setPosition(sanFrancisco, 20); // Set position with LatLng and source. streetViewPanorama.setPosition(sanFrancisco, StreetViewSource.OUTDOOR); // Set position with LaLng, radius and source. streetViewPanorama.setPosition(sanFrancisco, 20, StreetViewSource.OUTDOOR);
Alternatively, you can set the location based upon a panorama ID by passing a
panoId
to StreetViewPanorama.setPosition()
.
To retrieve the panorama ID for adjacent panoramas, first use
getLocation()
to retrieve a StreetViewPanoramaLocation
.
This object contains the ID of the
current panorama and an array of StreetViewPanoramaLink
objects, each
of which contains the ID of a panorama connected to the current one.
Kotlin
streetViewPanorama.location.links.firstOrNull()?.let { link: StreetViewPanoramaLink -> streetViewPanorama.setPosition(link.panoId) }
Java
StreetViewPanoramaLocation location = streetViewPanorama.getLocation(); if (location != null && location.links != null) { streetViewPanorama.setPosition(location.links[0].panoId); }
Zoom in and out
You can change the zoom level programmatically by setting
StreetViewPanoramaCamera.zoom
.
Setting the zoom to 1.0 will magnify the image
by a factor of 2.
The following snippet uses StreetViewPanoramaCamera.Builder()
to construct a
new camera with the tilt and bearing of the existing camera, while increasing
the zoom by fifty percent.
Kotlin
val zoomBy = 0.5f val camera = StreetViewPanoramaCamera.Builder() .zoom(streetViewPanorama.panoramaCamera.zoom + zoomBy) .tilt(streetViewPanorama.panoramaCamera.tilt) .bearing(streetViewPanorama.panoramaCamera.bearing) .build()
Java
float zoomBy = 0.5f; StreetViewPanoramaCamera camera = new StreetViewPanoramaCamera.Builder() .zoom(streetViewPanorama.getPanoramaCamera().zoom + zoomBy) .tilt(streetViewPanorama.getPanoramaCamera().tilt) .bearing(streetViewPanorama.getPanoramaCamera().bearing) .build();
Set the camera orientation (point of view)
You can determine the Street View camera's orientation by setting the
bearing and tilt on StreetViewPanoramaCamera
.
- bearing
- The direction in which the camera is pointing, specified in degrees clockwise from true north, around the camera locus. True north is 0, east is 90, south is 180, west is 270.
- tilt
- The Y-axis tilt up or down. The range is -90 through 0 to 90, with -90 looking straight down, 0 centered on the horizon, and 90 looking straight up. The variance is measured from the camera's initial default pitch, which is often (but not always) flat horizontal. For example, an image taken on a hill will probably have a default pitch that is not horizontal.
The following snippet uses StreetViewPanoramaCamera.Builder()
to construct a
new camera with the zoom and tilt of the existing camera, while changing the
bearing by 30 degrees to the left.
Kotlin
val panBy = 30f val camera = StreetViewPanoramaCamera.Builder() .zoom(streetViewPanorama.panoramaCamera.zoom) .tilt(streetViewPanorama.panoramaCamera.tilt) .bearing(streetViewPanorama.panoramaCamera.bearing - panBy) .build()
Java
float panBy = 30; StreetViewPanoramaCamera camera = new StreetViewPanoramaCamera.Builder() .zoom(streetViewPanorama.getPanoramaCamera().zoom) .tilt(streetViewPanorama.getPanoramaCamera().tilt) .bearing(streetViewPanorama.getPanoramaCamera().bearing - panBy) .build();
The following snippet tilts the camera upward by 30 degrees.
Kotlin
var tilt = streetViewPanorama.panoramaCamera.tilt + 30 tilt = if (tilt > 90) 90f else tilt val previous = streetViewPanorama.panoramaCamera val camera = StreetViewPanoramaCamera.Builder(previous) .tilt(tilt) .build()
Java
float tilt = streetViewPanorama.getPanoramaCamera().tilt + 30; tilt = (tilt > 90) ? 90 : tilt; StreetViewPanoramaCamera previous = streetViewPanorama.getPanoramaCamera(); StreetViewPanoramaCamera camera = new StreetViewPanoramaCamera.Builder(previous) .tilt(tilt) .build();
Animate the camera movements
To animate the camera movements, call
StreetViewPanorama.animateTo()
.
The animation interpolates between the current camera attributes and the new
camera attributes. If you want to jump directly to the camera without animation,
you can set the duration to 0.
Kotlin
// Keeping the zoom and tilt. Animate bearing by 60 degrees in 1000 milliseconds. val duration: Long = 1000 val camera = StreetViewPanoramaCamera.Builder() .zoom(streetViewPanorama.panoramaCamera.zoom) .tilt(streetViewPanorama.panoramaCamera.tilt) .bearing(streetViewPanorama.panoramaCamera.bearing - 60) .build() streetViewPanorama.animateTo(camera, duration)
Java
// Keeping the zoom and tilt. Animate bearing by 60 degrees in 1000 milliseconds. long duration = 1000; StreetViewPanoramaCamera camera = new StreetViewPanoramaCamera.Builder() .zoom(streetViewPanorama.getPanoramaCamera().zoom) .tilt(streetViewPanorama.getPanoramaCamera().tilt) .bearing(streetViewPanorama.getPanoramaCamera().bearing - 60) .build(); streetViewPanorama.animateTo(camera, duration);
The following image shows the result when you schedule the above animation to run
every 2000 milliseconds, using Handler.postDelayed()
:
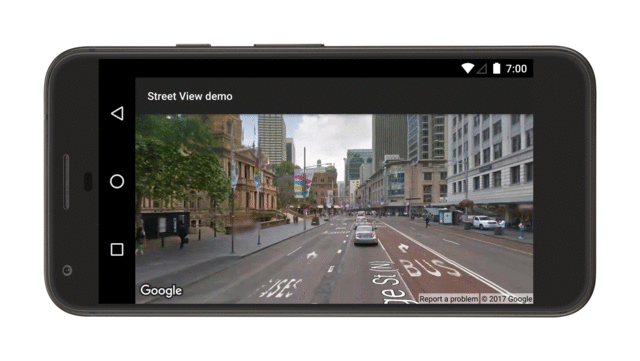