مقدمة
تحدد العلامة موقعًا على الخريطة. بشكل افتراضي، تستخدم العلامة
وصورة قياسية. يمكن للعلامات عرض صور مخصصة، وفي هذه الحالة تكون
يُشار إليها عادةً باسم "الأيقونات". العلامات والرموز هي كائنات من النوع
Marker
ويمكنك تعيين رمز مخصص داخل الدالة الإنشائية للعلامة أو من خلال
جارٍ طلب setIcon()
على محدّد الموقع. الاطّلاع على مزيد من المعلومات حول
تخصيص صورة العلامة.
بشكل عام، تعد العلامات نوعًا من التراكب. للحصول على معلومات عن أنواع التراكب، راجع الرسم على الخريطة:
تم تصميم العلامات لتكون تفاعلية. على سبيل المثال، بشكل افتراضي
تلقّي أحداث "'click'
"، وبالتالي يمكنك إضافة أداة معالجة الأحداث إلى
إحضار
نافذة المعلومات
عرض المعلومات المخصصة. يمكنك السماح للمستخدمين بنقل إحدى العلامات على
من خلال ضبط سمة draggable
للعلامة على
true
لمزيد من المعلومات عن العلامات القابلة للسحب، راجع
أدناه.
إضافة علامة
تشير رسالة الأشكال البيانية
google.maps.Marker
تأخذ الدالة الإنشائية كائن Marker options
واحدًا
حرفي، من خلال تحديد الخصائص الأولية للعلامة.
تُعد الحقول التالية مهمة بشكل خاص ويتم تعيينها بشكل شائع عند إنشاء علامة:
-
يحدّد
position
(مطلوب)LatLng
يحدد الموقع الأولي للعلامة. وَاحِدْ لاسترجاعLatLng
، هي باستخدام خدمة ترميز المواقع الجغرافية: -
تحدّد السمة
map
(اختيارية) السمةMap
التي يتم ضع العلامة. إذا لم يتم تحديد الخريطة عند إنشاء محدّد الموقع، يتمّ إنشاء العلامة ولكن لم يتمّ إرفاقها بـ (أو عرضها) الخريطة. يمكنك إضافة محدد الموقع لاحقًا عن طريق استدعاء علامة طريقةsetMap()
.
يضيف المثال التالي علامة بسيطة إلى خريطة في أولورو، في وسط أستراليا:
TypeScript
function initMap(): void { const myLatLng = { lat: -25.363, lng: 131.044 }; const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 4, center: myLatLng, } ); new google.maps.Marker({ position: myLatLng, map, title: "Hello World!", }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
function initMap() { const myLatLng = { lat: -25.363, lng: 131.044 }; const map = new google.maps.Map(document.getElementById("map"), { zoom: 4, center: myLatLng, }); new google.maps.Marker({ position: myLatLng, map, title: "Hello World!", }); } window.initMap = initMap;
تجربة "عيّنة"
في المثال أعلاه، يتم وضع العلامة على الخريطة عند إنشاء
العلامة التي تستخدم السمة map
في خيارات العلامة.
أو يمكنك إضافة المُحدِّد إلى الخريطة مباشرةً باستخدام
للعلامة setMap()
، كما هو موضح في المثال أدناه:
var myLatlng = new google.maps.LatLng(-25.363882,131.044922); var mapOptions = { zoom: 4, center: myLatlng } var map = new google.maps.Map(document.getElementById("map"), mapOptions); var marker = new google.maps.Marker({ position: myLatlng, title:"Hello World!" }); // To add the marker to the map, call setMap(); marker.setMap(map);
سيظهر title
للعلامة كتلميح.
إذا كنت لا تريد تمرير أي Marker options
في
الدالة الإنشائية للعلامة، ولكن يمكنك بدلاً من ذلك تمرير كائن فارغ {}
في
الوسيطة الأخيرة للدالة الإنشائية.
إزالة علامة
لإزالة محدِّد من الخريطة، يمكنك استدعاء طريقة setMap()
تمرير null
كوسيطة.
marker.setMap(null);
لاحظ أن الطريقة المذكورة أعلاه لا تحذف العلامة. حيث تزيل
محدد من الخريطة. إذا كنت تريد حذف محدد الموقع بدلاً من ذلك، فيجب
إزالته من الخريطة، ثم تعيين محدّد الموقع نفسه على
null
إذا كنت ترغب في إدارة مجموعة من العلامات، فينبغي عليك إنشاء صفيف للاحتفاظ
العلامات. باستخدام هذه الصفيفة، يمكنك بعد ذلك استدعاء setMap()
على
كل علامة في الصفيفة بدورها عندما تحتاج إلى إزالة العلامات. إِنْتَ
حذف محدّدات المواقع بإزالتها من الخريطة ثم إعداد
من length
إلى 0
للصفيف، ما يؤدي إلى إزالة جميع
المراجع إلى العلامات.
تخصيص صورة محدّد موقع
يمكنك تخصيص المظهر المرئي للعلامات من خلال تحديد image file أو رمز قائم على المتّجهات المطلوب عرضه بدلاً من الرمز التلقائي رمز الدبوس في "خرائط Google" يمكنك إضافة نص باستخدام علامة التصنيف، واستخدام الرموز المعقّدة لتحديد المناطق القابلة للنقر وتعيين ترتيب تسلسل استدعاء الدوال البرمجية للعلامات.
علامات مع رموز صور
في الحالة الأساسية، يمكن للأيقونة تحديد صورة لاستخدامها بدلاً من
رمز الدبوس التلقائي في "خرائط Google". لتحديد مثل هذا الرمز، اضبط
السمة icon
الخاصة بالعلامة التجارية على عنوان URL الخاص بصورة. تعمل واجهة برمجة تطبيقات JavaScript للخرائط على تحديد حجم الرمز تلقائيًا.
TypeScript
// This example adds a marker to indicate the position of Bondi Beach in Sydney, // Australia. function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 4, center: { lat: -33, lng: 151 }, } ); const image = "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png"; const beachMarker = new google.maps.Marker({ position: { lat: -33.89, lng: 151.274 }, map, icon: image, }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// This example adds a marker to indicate the position of Bondi Beach in Sydney, // Australia. function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 4, center: { lat: -33, lng: 151 }, }); const image = "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png"; const beachMarker = new google.maps.Marker({ position: { lat: -33.89, lng: 151.274 }, map, icon: image, }); } window.initMap = initMap;
تجربة "عيّنة"
علامات برموز متجهات
يمكنك استخدام مسارات متجهات SVG مخصصة لتحديد المظهر المرئي
. للقيام بذلك، عليك تمرير كائن Symbol
حرفيًا مع
المسار المطلوب إلى السمة icon
الخاصة بالعلامة التجارية. يمكنك تحديد
مسار مخصص باستخدام
تدوين مسار SVG، أو استخدِم أحد المسارات المحدَّدة مسبقًا في
google.maps.SymbolPath. يجب إدخال السمة anchor
لكي يتم استخدام العلامة
العرض بشكل صحيح عندما يتغير مستوى التكبير/التصغير. مزيد من المعلومات حول
استخدام الرموز لإنشاء رموز متجهات
للعلامات (والخطوط المتعددة).
TypeScript
// This example uses SVG path notation to add a vector-based symbol // as the icon for a marker. The resulting icon is a marker-shaped // symbol with a blue fill and no border. function initMap(): void { const center = new google.maps.LatLng(-33.712451, 150.311823); const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 9, center: center, } ); const svgMarker = { path: "M-1.547 12l6.563-6.609-1.406-1.406-5.156 5.203-2.063-2.109-1.406 1.406zM0 0q2.906 0 4.945 2.039t2.039 4.945q0 1.453-0.727 3.328t-1.758 3.516-2.039 3.070-1.711 2.273l-0.75 0.797q-0.281-0.328-0.75-0.867t-1.688-2.156-2.133-3.141-1.664-3.445-0.75-3.375q0-2.906 2.039-4.945t4.945-2.039z", fillColor: "blue", fillOpacity: 0.6, strokeWeight: 0, rotation: 0, scale: 2, anchor: new google.maps.Point(0, 20), }; new google.maps.Marker({ position: map.getCenter(), icon: svgMarker, map: map, }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// This example uses SVG path notation to add a vector-based symbol // as the icon for a marker. The resulting icon is a marker-shaped // symbol with a blue fill and no border. function initMap() { const center = new google.maps.LatLng(-33.712451, 150.311823); const map = new google.maps.Map(document.getElementById("map"), { zoom: 9, center: center, }); const svgMarker = { path: "M-1.547 12l6.563-6.609-1.406-1.406-5.156 5.203-2.063-2.109-1.406 1.406zM0 0q2.906 0 4.945 2.039t2.039 4.945q0 1.453-0.727 3.328t-1.758 3.516-2.039 3.070-1.711 2.273l-0.75 0.797q-0.281-0.328-0.75-0.867t-1.688-2.156-2.133-3.141-1.664-3.445-0.75-3.375q0-2.906 2.039-4.945t4.945-2.039z", fillColor: "blue", fillOpacity: 0.6, strokeWeight: 0, rotation: 0, scale: 2, anchor: new google.maps.Point(0, 20), }; new google.maps.Marker({ position: map.getCenter(), icon: svgMarker, map: map, }); } window.initMap = initMap;
تجربة "عيّنة"
تصنيفات العلامات
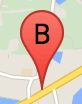
علامة العلامة هي حرف أو رقم يظهر داخل العلامة. تشير رسالة الأشكال البيانية
تعرض صورة العلامة في هذا القسم تصنيف علامة بالحرف "B"
عليها. يمكنك تحديد تصنيف علامة كسلسلة أو
MarkerLabel
يتضمن سلسلة وخصائص التصنيف الأخرى.
عند إنشاء علامة، يمكنك تحديد السمة label
في
الـ
MarkerOptions
الخاص بك. يمكنك بدلاً من ذلك الاتصال بـ setLabel()
على الرقم
محدِّد
لتعيين التصنيف على علامة حالية.
يعرض المثال التالي علامات مصنَّفة عندما ينقر المستخدم على الخريطة:
TypeScript
// In the following example, markers appear when the user clicks on the map. // Each marker is labeled with a single alphabetical character. const labels = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; let labelIndex = 0; function initMap(): void { const bangalore = { lat: 12.97, lng: 77.59 }; const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 12, center: bangalore, } ); // This event listener calls addMarker() when the map is clicked. google.maps.event.addListener(map, "click", (event) => { addMarker(event.latLng, map); }); // Add a marker at the center of the map. addMarker(bangalore, map); } // Adds a marker to the map. function addMarker(location: google.maps.LatLngLiteral, map: google.maps.Map) { // Add the marker at the clicked location, and add the next-available label // from the array of alphabetical characters. new google.maps.Marker({ position: location, label: labels[labelIndex++ % labels.length], map: map, }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// In the following example, markers appear when the user clicks on the map. // Each marker is labeled with a single alphabetical character. const labels = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; let labelIndex = 0; function initMap() { const bangalore = { lat: 12.97, lng: 77.59 }; const map = new google.maps.Map(document.getElementById("map"), { zoom: 12, center: bangalore, }); // This event listener calls addMarker() when the map is clicked. google.maps.event.addListener(map, "click", (event) => { addMarker(event.latLng, map); }); // Add a marker at the center of the map. addMarker(bangalore, map); } // Adds a marker to the map. function addMarker(location, map) { // Add the marker at the clicked location, and add the next-available label // from the array of alphabetical characters. new google.maps.Marker({ position: location, label: labels[labelIndex++ % labels.length], map: map, }); } window.initMap = initMap;
تجربة "عيّنة"
الأيقونات المعقدة
يمكنك تحديد أشكال معقدة للإشارة إلى المناطق القابلة للنقر،
تحديد كيفية ظهور الرموز بالنسبة للتراكبات الأخرى (
"ترتيب تسلسل استدعاء الدوال البرمجية"). يجب أن تحدد الرموز المحددة بهذه الطريقة
icon
الخصائص لكائن من النوع
Icon
Icon
الكائنات تحدد الصورة. وتحدّد أيضًا size
الرمز، origin
للرمز (إذا كانت الصورة التي تريدها جزءًا
صورة أكبر في الرموز المتحركة، على سبيل المثال)
anchor
الذي ينبغي أن تقع فيه نقطة اتصال الرمز (وهي
استنادًا إلى الأصل).
في حال استخدام تصنيف مع تصنيف مخصّص
يمكنك وضع التصنيف مع
الخاصية "labelOrigin
" في
Icon
الخاص بك.
TypeScript
// The following example creates complex markers to indicate beaches near // Sydney, NSW, Australia. Note that the anchor is set to (0,32) to correspond // to the base of the flagpole. function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 10, center: { lat: -33.9, lng: 151.2 }, } ); setMarkers(map); } // Data for the markers consisting of a name, a LatLng and a zIndex for the // order in which these markers should display on top of each other. const beaches: [string, number, number, number][] = [ ["Bondi Beach", -33.890542, 151.274856, 4], ["Coogee Beach", -33.923036, 151.259052, 5], ["Cronulla Beach", -34.028249, 151.157507, 3], ["Manly Beach", -33.80010128657071, 151.28747820854187, 2], ["Maroubra Beach", -33.950198, 151.259302, 1], ]; function setMarkers(map: google.maps.Map) { // Adds markers to the map. // Marker sizes are expressed as a Size of X,Y where the origin of the image // (0,0) is located in the top left of the image. // Origins, anchor positions and coordinates of the marker increase in the X // direction to the right and in the Y direction down. const image = { url: "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png", // This marker is 20 pixels wide by 32 pixels high. size: new google.maps.Size(20, 32), // The origin for this image is (0, 0). origin: new google.maps.Point(0, 0), // The anchor for this image is the base of the flagpole at (0, 32). anchor: new google.maps.Point(0, 32), }; // Shapes define the clickable region of the icon. The type defines an HTML // <area> element 'poly' which traces out a polygon as a series of X,Y points. // The final coordinate closes the poly by connecting to the first coordinate. const shape = { coords: [1, 1, 1, 20, 18, 20, 18, 1], type: "poly", }; for (let i = 0; i < beaches.length; i++) { const beach = beaches[i]; new google.maps.Marker({ position: { lat: beach[1], lng: beach[2] }, map, icon: image, shape: shape, title: beach[0], zIndex: beach[3], }); } } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// The following example creates complex markers to indicate beaches near // Sydney, NSW, Australia. Note that the anchor is set to (0,32) to correspond // to the base of the flagpole. function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 10, center: { lat: -33.9, lng: 151.2 }, }); setMarkers(map); } // Data for the markers consisting of a name, a LatLng and a zIndex for the // order in which these markers should display on top of each other. const beaches = [ ["Bondi Beach", -33.890542, 151.274856, 4], ["Coogee Beach", -33.923036, 151.259052, 5], ["Cronulla Beach", -34.028249, 151.157507, 3], ["Manly Beach", -33.80010128657071, 151.28747820854187, 2], ["Maroubra Beach", -33.950198, 151.259302, 1], ]; function setMarkers(map) { // Adds markers to the map. // Marker sizes are expressed as a Size of X,Y where the origin of the image // (0,0) is located in the top left of the image. // Origins, anchor positions and coordinates of the marker increase in the X // direction to the right and in the Y direction down. const image = { url: "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png", // This marker is 20 pixels wide by 32 pixels high. size: new google.maps.Size(20, 32), // The origin for this image is (0, 0). origin: new google.maps.Point(0, 0), // The anchor for this image is the base of the flagpole at (0, 32). anchor: new google.maps.Point(0, 32), }; // Shapes define the clickable region of the icon. The type defines an HTML // <area> element 'poly' which traces out a polygon as a series of X,Y points. // The final coordinate closes the poly by connecting to the first coordinate. const shape = { coords: [1, 1, 1, 20, 18, 20, 18, 1], type: "poly", }; for (let i = 0; i < beaches.length; i++) { const beach = beaches[i]; new google.maps.Marker({ position: { lat: beach[1], lng: beach[2] }, map, icon: image, shape: shape, title: beach[0], zIndex: beach[3], }); } } window.initMap = initMap;
تجربة "عيّنة"
جارٍ تحويل MarkerImage
عناصر إلى النوع Icon
حتى الإصدار 3.10 من واجهة برمجة تطبيقات JavaScript للخرائط، كانت الرموز المعقدة
تم تحديدها على أنّها عناصر MarkerImage
. تشير رسالة الأشكال البيانية
تمت إضافة قيمة حرفية لعنصر Icon
في الإصدار 3.10 واستبدالها.
MarkerImage
من الإصدار 3.11 والإصدارات الأحدث.
تتوافق القيم الحرفية لكائنات Icon
مع المعلَمات نفسها مثل
MarkerImage
، ما يتيح لك تحويل
MarkerImage
إلى Icon
عن طريق إزالة
الدالة الإنشائية، مع إحاطة المعاملات السابقة بـ {}
،
وإضافة أسماء كل معلمة. على سبيل المثال:
var image = new google.maps.MarkerImage( place.icon, new google.maps.Size(71, 71), new google.maps.Point(0, 0), new google.maps.Point(17, 34), new google.maps.Size(25, 25));
يصبح
var image = { url: place.icon, size: new google.maps.Size(71, 71), origin: new google.maps.Point(0, 0), anchor: new google.maps.Point(17, 34), scaledSize: new google.maps.Size(25, 25) };
علامات التحسين
يؤدي التحسين إلى تحسين الأداء من خلال عرض العديد من العلامات كعلامة واحدة عنصر ثابت. يفيد ذلك في الحالات التي يتم فيها نشر عدد كبير من العلامات مطلوبة. بشكل افتراضي، ستحدد واجهة برمجة تطبيقات JavaScript للخرائط ما إذا كان سيتم تحسين علامة أم لا. عندما يكون هناك عدد كبير من المستخدم، ستحاول واجهة برمجة تطبيقات JavaScript للخرائط عرض مع التحسين. لا يمكن تحسين كل العلامات؛ في بعض قد تحتاج واجهة برمجة تطبيقات JavaScript للخرائط إلى عرض محددات بدون تحسين. إيقاف العرض المحسَّن للصور المتحركة ملفات GIF أو PNG، أو عندما يجب عرض كل علامة كنموذج DOM منفصل العنصر. يوضح المثال التالي إنشاء علامة محسَّنة:
var marker = new google.maps.Marker({ position: myLatlng, title:"Hello World!", optimized: true });
إتاحة الوصول إلى علامة
يمكنك إتاحة الوصول إلى محدّد الموقع من خلال إضافة حدث استماع نقر،
ضبط optimized
على false
أداة معالجة النقرات
إلى اشتمال العلامة على دلالات الزر، والتي يمكن الوصول إليها باستخدام
التنقل بلوحة المفاتيح، وقارئات الشاشة، وما إلى ذلك. يمكنك استخدام
خيار title
لمشاركة نص يمكن الوصول إليه لعلامة معيّنة.
في المثال التالي، يتم التركيز على العلامة الأولى عندما تكون علامة التبويب مضغوطة يمكنك بعد ذلك استخدام مفاتيح الأسهم للتنقل بين العلامات. اضغط على انقر مرة أخرى لمتابعة التنقل من خلال بقية عناصر التحكم في الخريطة. إذا علامة الموقع في نافذة المعلومات، يمكنك فتحها بالنقر على العلامة أو من خلال الضغط على مفتاح Enter أو مفتاح المسافة عند تحديد العلامة. عندما تم إغلاق نافذة المعلومات، سيعود التركيز إلى العلامة المرتبطة.
TypeScript
// The following example creates five accessible and // focusable markers. function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 12, center: { lat: 34.84555, lng: -111.8035 }, } ); // Set LatLng and title text for the markers. The first marker (Boynton Pass) // receives the initial focus when tab is pressed. Use arrow keys to // move between markers; press tab again to cycle through the map controls. const tourStops: [google.maps.LatLngLiteral, string][] = [ [{ lat: 34.8791806, lng: -111.8265049 }, "Boynton Pass"], [{ lat: 34.8559195, lng: -111.7988186 }, "Airport Mesa"], [{ lat: 34.832149, lng: -111.7695277 }, "Chapel of the Holy Cross"], [{ lat: 34.823736, lng: -111.8001857 }, "Red Rock Crossing"], [{ lat: 34.800326, lng: -111.7665047 }, "Bell Rock"], ]; // Create an info window to share between markers. const infoWindow = new google.maps.InfoWindow(); // Create the markers. tourStops.forEach(([position, title], i) => { const marker = new google.maps.Marker({ position, map, title: `${i + 1}. ${title}`, label: `${i + 1}`, optimized: false, }); // Add a click listener for each marker, and set up the info window. marker.addListener("click", () => { infoWindow.close(); infoWindow.setContent(marker.getTitle()); infoWindow.open(marker.getMap(), marker); }); }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// The following example creates five accessible and // focusable markers. function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 12, center: { lat: 34.84555, lng: -111.8035 }, }); // Set LatLng and title text for the markers. The first marker (Boynton Pass) // receives the initial focus when tab is pressed. Use arrow keys to // move between markers; press tab again to cycle through the map controls. const tourStops = [ [{ lat: 34.8791806, lng: -111.8265049 }, "Boynton Pass"], [{ lat: 34.8559195, lng: -111.7988186 }, "Airport Mesa"], [{ lat: 34.832149, lng: -111.7695277 }, "Chapel of the Holy Cross"], [{ lat: 34.823736, lng: -111.8001857 }, "Red Rock Crossing"], [{ lat: 34.800326, lng: -111.7665047 }, "Bell Rock"], ]; // Create an info window to share between markers. const infoWindow = new google.maps.InfoWindow(); // Create the markers. tourStops.forEach(([position, title], i) => { const marker = new google.maps.Marker({ position, map, title: `${i + 1}. ${title}`, label: `${i + 1}`, optimized: false, }); // Add a click listener for each marker, and set up the info window. marker.addListener("click", () => { infoWindow.close(); infoWindow.setContent(marker.getTitle()); infoWindow.open(marker.getMap(), marker); }); }); } window.initMap = initMap;
تجربة "عيّنة"
تحريك علامة
يمكنك تحريك العلامات بحيث تظهر حركة ديناميكية في مجموعة متنوعة
من الظروف المختلفة. لتحديد الطريقة التي تتحرك بها العلامة، استخدم
السمة animation
الخاصة بالعلامة التجارية، من النوع
google.maps.Animation
ما يلي:
قيم Animation
مسموح بها:
-
يشير
DROP
إلى أنه يجب إفلات العلامة من أعلى الخريطة إلى موقعها النهائي عند وضعها لأول مرة على الخريطة. صورة متحركة سيتوقّف عند وضع العلامة وسيعملanimation
العودة إلىnull
يتم عادةً تحديد هذا النوع من الرسوم المتحركة أثناء إنشاءMarker
. -
تشير القيمة
BOUNCE
إلى أنّ العلامة يجب أن ترتد في مكانها. حاسمة ستستمر علامة الارتداد في الارتداد حتى تم ضبط السمةanimation
صراحةً علىnull
.
يمكنك بدء رسم متحرك على علامة حالية من خلال استدعاء
setAnimation()
في الكائن Marker
.
TypeScript
// The following example creates a marker in Stockholm, Sweden using a DROP // animation. Clicking on the marker will toggle the animation between a BOUNCE // animation and no animation. let marker: google.maps.Marker; function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 13, center: { lat: 59.325, lng: 18.07 }, } ); marker = new google.maps.Marker({ map, draggable: true, animation: google.maps.Animation.DROP, position: { lat: 59.327, lng: 18.067 }, }); marker.addListener("click", toggleBounce); } function toggleBounce() { if (marker.getAnimation() !== null) { marker.setAnimation(null); } else { marker.setAnimation(google.maps.Animation.BOUNCE); } } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// The following example creates a marker in Stockholm, Sweden using a DROP // animation. Clicking on the marker will toggle the animation between a BOUNCE // animation and no animation. let marker; function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 13, center: { lat: 59.325, lng: 18.07 }, }); marker = new google.maps.Marker({ map, draggable: true, animation: google.maps.Animation.DROP, position: { lat: 59.327, lng: 18.067 }, }); marker.addListener("click", toggleBounce); } function toggleBounce() { if (marker.getAnimation() !== null) { marker.setAnimation(null); } else { marker.setAnimation(google.maps.Animation.BOUNCE); } } window.initMap = initMap;
تجربة "عيّنة"
إذا كان لديك العديد من العلامات، فقد لا ترغب في إسقاطها على الخريطة جميعًا
دفعة واحدة. يمكنك الاستفادة من setTimeout()
في إنشاء مسافة
أقلام تحديد والرسوم المتحركة باستخدام نمط مثل ما هو موضح أدناه:
function drop() { for (var i =0; i < markerArray.length; i++) { setTimeout(function() { addMarkerMethod(); }, i * 200); } }
جعل محدّد الموقع قابلاً للسحب
للسماح للمستخدمين بسحب علامة إلى موقع مختلف على الخريطة، قم بتعيين
من draggable
إلى true
في خيارات محدّد الموقع.
var myLatlng = new google.maps.LatLng(-25.363882,131.044922); var mapOptions = { zoom: 4, center: myLatlng } var map = new google.maps.Map(document.getElementById("map"), mapOptions); // Place a draggable marker on the map var marker = new google.maps.Marker({ position: myLatlng, map: map, draggable:true, title:"Drag me!" });
تخصيص علامات إضافية
للحصول على علامة مخصصة بالكامل، راجع مثال على نافذة منبثقة مخصّصة
للحصول على مزيد من الإضافات في فئة محدّد الموقع، وتجميع العلامات وإدارتها، وتخصيص التراكب، يمكنك الاطلاع على المكتبات المفتوحة المصدر.