高度なマーカーでは、次の 2 つのクラスを使用してマーカーを定義します。
GMSAdvancedMarker
クラスがデフォルトのマーカーを提供
、GMSPinImageOptions
にはオプションが含まれます。
カスタマイズすることもできます。このページでは、
できます。
- 背景色を変更する
- 輪郭線の色を変更する
- グリフの色を変更する
- グリフテキストを変更する
- iconView プロパティを使用してカスタムビューとアニメーションをサポートする
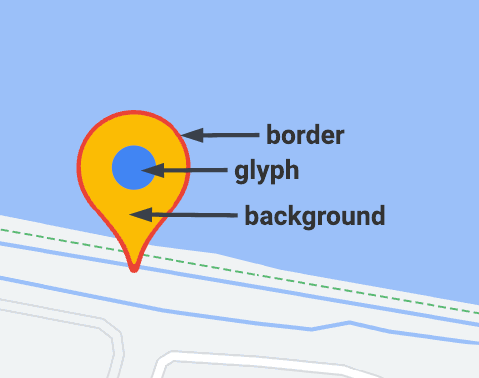
背景色を変更する
GMSPinImageOptions.backgroundColor
オプションを使用して、以下を行います。
マーカーの背景色を変更する。
Swift
//... let options = GMSPinImageOptions() options.backgroundColor = .blue let pinImage = GMSPinImage(options: options) advancedMarker.icon = pinImage advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.backgroundColor = [UIColor blueColor]; GMSPinImage *pin = [GMSPinImage pinImageWithOptions:options]; customTextMarker.icon = pin; customTextMarker.map = mapView;
輪郭線の色を変更する
GMSPinImageOptions.borderColor
オプションを使用して変更する
マーカーの背景色。
Swift
//... let options = GMSPinImageOptions() options.borderColor = .blue let pinImage = GMSPinImage(options: options) advancedMarker.icon = pinImage advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.backgroundColor = [UIColor blueColor]; GMSPinImage *pin = [GMSPinImage pinImageWithOptions:options]; advancedMarker.icon = pin; advancedMarker.map = mapView;
グリフの色を変更する
GMSPinImageGlyph.glyphColor
を使用して背景を変更する
マーカーの色。
Swift
//... let options = GMSPinImageOptions() let glyph = GMSPinImageGlyph(glyphColor: .yellow) options.glyph = glyph let glyphColor = GMSPinImage(options: options) advancedMarker.icon = glyphColor advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.glyph = [[GMSPinImageGlyph alloc] initWithGlyphColor:[UIColor yellowColor]]; GMSPinImage *glyphColor = [GMSPinImage pinImageWithOptions:options]; advancedMarker.icon = glyphColor; advancedMarker.map = mapView;
グリフテキストを変更する
マーカーのグリフテキストを変更するには、GMSPinImageGlyph
を使用します。
Swift
//... let options = GMSPinImageOptions() let glyph = GMSPinImageGlyph(text: "ABC", textColor: .white) options.glyph = glyph let pinImage = GMSPinImage(options: options) advancedMarker.icon = pinImage advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.glyph = [[GMSPinImageGlyph alloc] initWithText:@"ABC" textColor:[UIColor whiteColor]]; GMSPinImage *pin = [GMSPinImage pinImageWithOptions:options]; customTextMarker.icon = pin; customTextMarker.map = mapView;
iconView
プロパティを使用してカスタムビューとアニメーションをサポートする
GMSMarker
と同様、GMSAdvancedMarker
では、
iconView
。
iconView
プロパティは、アニメーション化可能なすべてのプロパティのアニメーションをサポートします。
フレームと中央を除く UIView
。iconViews
を含むマーカーはサポートされていません。
と icons
が同じ地図に表示されます。
Swift
//... let advancedMarker = GMSAdvancedMarker(position: coordinate) advancedMarker.iconView = customView() advancedMarker.map = mapView func customView() -> UIView { // return your custom UIView. }
Objective-C
//... GMSAdvancedMarker *advancedMarker = [GMSAdvancedMarker markerWithPosition:kCoordinate]; advancedMarker.iconView = [self customView]; advancedMarker.map = self.mapView; - (UIView *)customView { // return custom view }
レイアウトの制約
GMSAdvancedMarker
はレイアウトを直接サポートしていません
その iconView
の制約です。ただし、ユーザーにレイアウトの制約を
iconView
内のインターフェース要素です。ビューを作成すると、オブジェクトは
frame
または size
をマーカーに渡す必要があります。
Swift
//do not set advancedMarker.iconView.translatesAutoresizingMaskIntoConstraints = false let advancedMarker = GMSAdvancedMarker(position: coordinate) let customView = customView() //set frame customView.frame = CGRect(0, 0, width, height) advancedMarker.iconView = customView
Objective-C
//do not set advancedMarker.iconView.translatesAutoresizingMaskIntoConstraints = NO; GMSAdvancedMarker *advancedMarker = [GMSAdvancedMarker markerWithPosition:kCoordinate]; CustomView *customView = [self customView]; //set frame customView.frame = CGRectMake(0, 0, width, height); advancedMarker.iconView = customView;