На этой странице описано, как ваше приложение чата может открывать диалоговые окна для ответа пользователям.
Диалоги представляют собой оконные интерфейсы на основе карточек, которые открываются из чата или сообщения. Диалог и его содержимое видны только пользователю, который его открыл.
Приложения чата могут использовать диалоговые окна для запроса и сбора информации от пользователей чата, включая многоэтапные формы. Дополнительные сведения о создании входных данных формы см. в разделе Сбор и обработка информации от пользователей .
Предварительные условия
Открыть диалог
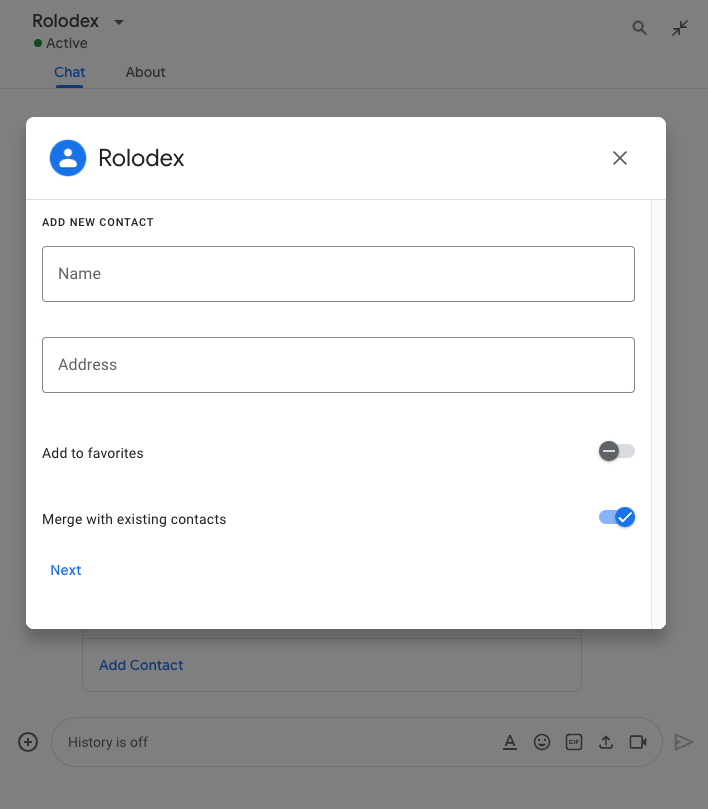
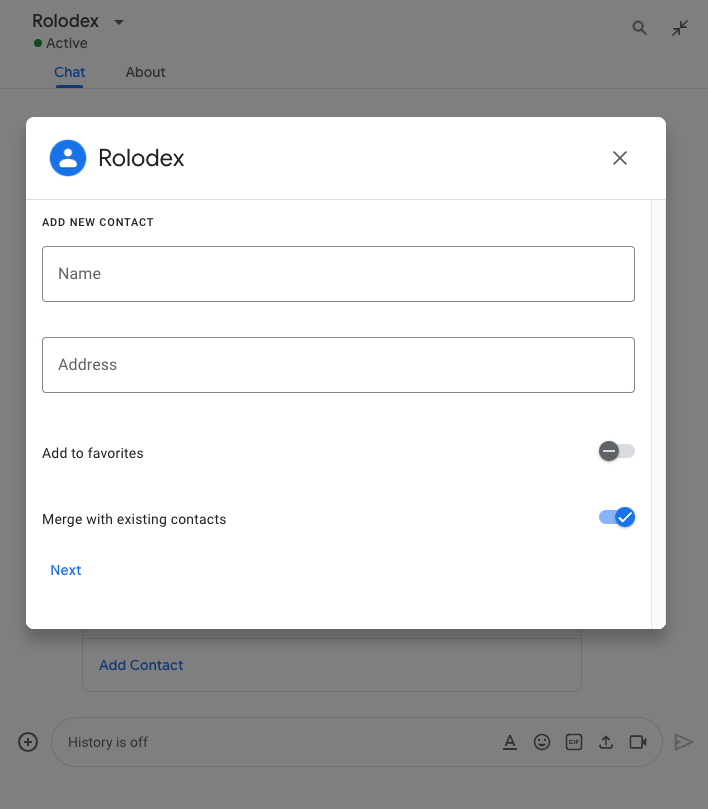
В этом разделе объясняется, как ответить и настроить диалог, выполнив следующие действия:
- Запускайте запрос диалога при взаимодействии с пользователем.
- Обработайте запрос, вернувшись и открыв диалоговое окно.
- После того как пользователи отправят информацию, обработайте отправку, закрыв диалоговое окно или вернув другое диалоговое окно.
Запустить запрос диалога
Приложение чата может открывать диалоговые окна только для ответа на взаимодействие с пользователем, например на команду или нажатие кнопки в сообщении на карточке.
Чтобы ответить пользователям с помощью диалогового окна, приложение Chat должно создать взаимодействие, которое запускает запрос диалогового окна, например следующее:
- Ответить на команду. Чтобы инициировать запрос от команды, необходимо установить флажок Открывает диалог при настройке команды .
- Ответьте на нажатие кнопки в сообщении , либо в карточке, либо в нижней части сообщения. Чтобы инициировать запрос от кнопки в сообщении, вы настраиваете действие кнопки
onClick
, устанавливая для нееinteraction
OPEN_DIALOG
. - Ответьте на нажатие кнопки на главной странице приложения Chat . Подробнее об открытии диалоговых окон с домашних страниц см. в разделе Создание домашней страницы для приложения Google Chat .
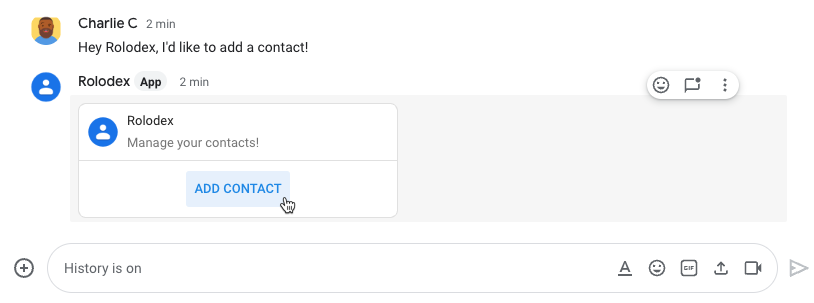
/addContact
.Сообщение также включает кнопку, которую пользователи могут нажать для запуска команды.
В следующем примере кода показано, как инициировать запрос диалогового окна с помощью кнопки в сообщении с карточкой. Чтобы открыть диалоговое окно, в поле button.interaction
установлено значение OPEN_DIALOG
:
В этом примере отправляется карточное сообщение, возвращая card JSON . Вы также можете использовать службу карточек Apps Script .
Открыть начальный диалог
Когда пользователь запускает запрос диалога, ваше приложение Chat получает событие взаимодействия, представленное как тип event
в Chat API. Если взаимодействие запускает запрос диалога, поле dialogEventType
события устанавливается в REQUEST_DIALOG
.
Чтобы открыть диалоговое окно, ваше приложение Chat может ответить на запрос, вернув объект actionResponse
с type
, установленным в DIALOG
и объект Message
. Чтобы указать содержимое диалога, вы включаете следующие объекты:
- Объект
actionResponse
сtype
DIALOG
. - Объект
dialogAction
. Полеbody
содержит элементы пользовательского интерфейса (UI), которые будут отображаться на карточке, включая один или несколькоsections
виджетов. Для сбора информации от пользователей вы можете указать виджеты ввода формы и виджет кнопки. Дополнительные сведения о разработке входных данных для форм см. в разделе Сбор и обработка информации от пользователей .
В следующем примере кода показано, как приложение Chat возвращает ответ, открывающий диалоговое окно:
В этом примере отправляется карточное сообщение, возвращая card JSON . Вы также можете использовать службу карточек Apps Script .
Обработка отправки диалога
Когда пользователи нажимают кнопку, которая отправляет диалоговое окно, ваше приложение Chat получает событие взаимодействия CARD_CLICKED
, где dialogEventType
установлено значение SUBMIT_DIALOG
.
Ваше приложение чата должно обрабатывать событие взаимодействия, выполнив одно из следующих действий:
- Верните другое диалоговое окно , чтобы заполнить другую карточку или форму.
- Закройте диалоговое окно после проверки данных, отправленных пользователем, и, при необходимости, отправьте сообщение с подтверждением.
Необязательно: вернуть другое диалоговое окно.
После того как пользователи отправят исходное диалоговое окно, приложения чата могут вернуть одно или несколько дополнительных диалоговых окон, которые помогут пользователям просмотреть информацию перед отправкой, заполнить многоэтапные формы или динамически заполнить содержимое формы.
Для обработки данных, вводимых пользователями, приложение Chat использует объект event.common.formInputs
. Дополнительные сведения о получении значений из виджетов ввода см. в разделе Сбор и обработка информации от пользователей .
Чтобы отслеживать любые данные, которые пользователи вводят из начального диалогового окна, необходимо добавить параметры к кнопке, открывающей следующее диалоговое окно. Подробности см. в разделе Перенос данных на другую карту .
В этом примере приложение чата открывает начальное диалоговое окно, которое ведет ко второму диалоговому окну для подтверждения перед отправкой:
В этом примере отправляется карточное сообщение, возвращая card JSON . Вы также можете использовать службу карточек Apps Script .
Закрыть диалог
Когда пользователи нажимают кнопку в диалоговом окне, ваше приложение Chat выполняет соответствующее действие и предоставляет объекту события следующую информацию:
-
eventType
—CARD_CLICKED
. -
dialogEventType
—SUBMIT_DIALOG
.
Приложение Chat должно возвращать объект ActionResponse
с type
DIALOG
и dialogAction
.
Необязательно: отображение уведомления
При закрытии диалогового окна вы также можете отобразить текстовое уведомление.
Приложение Chat может ответить уведомлением об успехе или ошибке, вернув ActionResponse
с установленным actionStatus
.
В следующем примере проверяется корректность параметров и закрывается диалоговое окно с текстовым уведомлением в зависимости от результата:
В этом примере отправляется карточное сообщение, возвращая card JSON . Вы также можете использовать службу карточек Apps Script .
Подробности о передаче параметров между диалогами см. в разделе Перенос данных на другую карту .
Необязательно: отправьте сообщение с подтверждением.
Закрывая диалоговое окно, вы также можете отправить новое сообщение или обновить существующее.
Чтобы отправить новое сообщение, верните объект ActionResponse
с type
NEW_MESSAGE
. В следующем примере диалоговое окно закрывается с текстовым уведомлением и текстовым сообщением с подтверждением:
В этом примере отправляется карточное сообщение, возвращая card JSON . Вы также можете использовать службу карточек Apps Script .
Чтобы обновить сообщение, верните объект actionResponse
, который содержит обновленное сообщение и задает один из следующих type
:
-
UPDATE_MESSAGE
: Обновляет сообщение, вызвавшее запрос диалога . -
UPDATE_USER_MESSAGE_CARDS
: обновляет карточку из предварительного просмотра ссылки .
Устранение неполадок
Когда приложение или карточка Google Chat возвращает ошибку, в интерфейсе Chat отображается сообщение «Что-то пошло не так». или «Невозможно обработать ваш запрос». Иногда в пользовательском интерфейсе чата не отображается сообщение об ошибке, но приложение или карточка чата выдает неожиданный результат; например, сообщение с карточкой может не появиться.
Хотя сообщение об ошибке может не отображаться в пользовательском интерфейсе чата, доступны описательные сообщения об ошибках и данные журнала, которые помогут вам исправить ошибки, если включено ведение журнала ошибок для приложений чата. Информацию о просмотре, отладке и исправлении ошибок см. в разделе «Устранение неполадок и исправление ошибок Google Chat» .
Связанные темы
- Ознакомьтесь с примером диспетчера контактов — приложения чата, использующего диалоговые окна для сбора контактной информации.
- Открывайте диалоги на главной странице приложения Google Chat .
- Отвечать на команды приложения Google Chat
- Информация о процессе, введенная пользователями