This page explains how to build a homepage for direct messages with your Google Chat app. A homepage, referred to as app home in the Google Chat API, is a customizable card interface that appears in the Home tab of direct message spaces between a user and a Chat app.

You can use app home to share tips for interacting with the Chat app or letting users access and use an external service or tool from Chat.
Use the Card Builder to design and preview messaging and user interfaces for Chat apps:
Open the Card BuilderPrerequisites
Node.js
A Google Chat app that's enabled for interactive features. To create an interactive Chat app using an HTTP service, complete this quickstart.
Python
A Google Chat app that's enabled for interactive features. To create an interactive Chat app using an HTTP service, complete this quickstart.
Java
A Google Chat app that's enabled for interactive features. To create an interactive Chat app using an HTTP service, complete this quickstart.
Apps Script
A Google Chat app that's enabled for interactive features. To create an interactive Chat app in Apps Script, complete this quickstart.
Configure app home for your Chat app
To support app home, you must configure your Chat app
to receive
APP_HOME
interaction events,
Your Chat app receives this event whenever a user
clicks the Home tab from a direct message with the
Chat app.
To update your configuration settings in the Google Cloud console, do the following:
In the Google Cloud console, go to Menu > More products > Google Workspace > Product Library > Google Chat API.
Click Manage, and then click the Configuration tab.
Under Interactive features, go to the Functionality section to configure app home:
- Select the Receive 1:1 messages checkbox.
- Select the Support App Home checkbox.
If your Chat app uses an HTTP service, go to Connection settings and specify an endpoint for the App Home URL field. You can use the same URL that you specified in the HTTP endpoint URL field.
Click Save.
Build an app home card
When a user opens app home, your Chat app must handle
the APP_HOME
interaction event by returning an instance of
RenderActions
with pushCard
navigation and a
Card
. To create an
interactive experience, the card can contain interactive widgets such as buttons
or text inputs that the Chat app can process and
respond to with additional cards, or a dialog.
In the following example, the Chat app displays an initial app home card that displays the time the card was created and a button. When a user clicks the button, the Chat app returns an updated card that displays the time the updated card was created.
Node.js
Python
Java
Apps Script
Implement the onAppHome
function that is called after all APP_HOME
interaction events:
This example sends a card message by returning card JSON. You can also use the Apps Script card service.
Respond to app home interactions
If your initial app home card contains interactive widgets, such as buttons
or selection inputs, your Chat app must handle
the related interaction events by returning an instance of
RenderActions
with updateCard
navigation. To learn more about handling interactive
widgets, see
Process information inputted by users.
In the previous example, the initial app home card included a button. Whenever
a user clicks the button, a CARD_CLICKED
interaction event
triggers the function updateAppHome
to refresh the app home card, as shown
in the following code:
Node.js
Python
Java
Apps Script
This example sends a card message by returning card JSON. You can also use the Apps Script card service.
Open dialogs
Your Chat app can also respond to interactions in app home by opening dialogs.
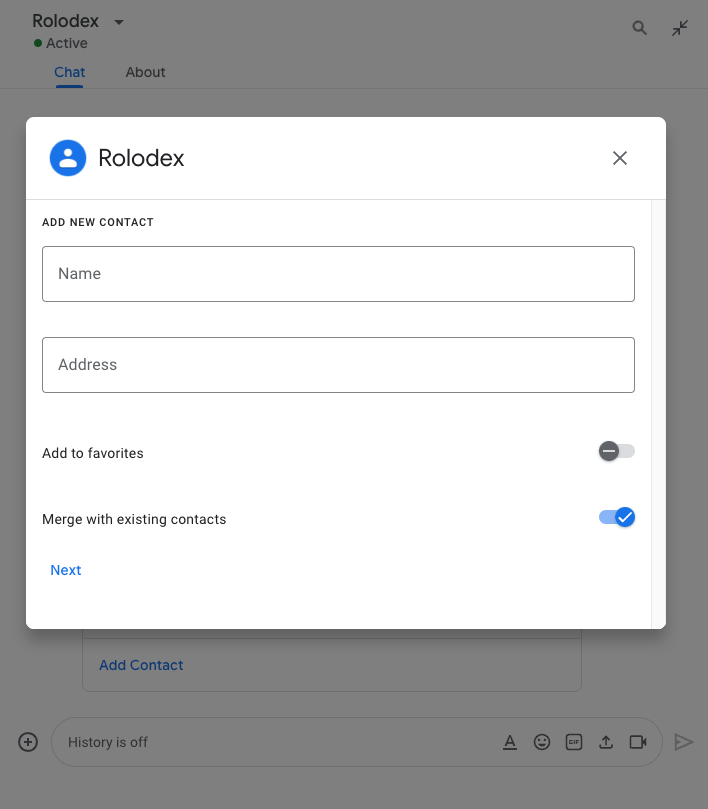
To open a dialog from app home, process the related interaction event by
returning renderActions
with updateCard
navigation that contains a Card
object. In the following example, a Chat app responds
to a button click from an app home card by processing the CARD_CLICKED
interaction event and opening a dialog:
{ renderActions: { action: { navigations: [{ updateCard: { sections: [{
header: "Add new contact",
widgets: [{ "textInput": {
label: "Name",
type: "SINGLE_LINE",
name: "contactName"
}}, { textInput: {
label: "Address",
type: "MULTIPLE_LINE",
name: "address"
}}, { decoratedText: {
text: "Add to favorites",
switchControl: {
controlType: "SWITCH",
name: "saveFavorite"
}
}}, { decoratedText: {
text: "Merge with existing contacts",
switchControl: {
controlType: "SWITCH",
name: "mergeContact",
selected: true
}
}}, { buttonList: { buttons: [{
text: "Next",
onClick: { action: { function: "openSequentialDialog" }}
}]}}]
}]}}]}}}
To close a dialog, process the following interaction events:
CLOSE_DIALOG
: Closes the dialog and returns to the Chat app's initial app home card.CLOSE_DIALOG_AND_EXECUTE
: Closes the dialog and refreshes the app home card.
The following code sample uses CLOSE_DIALOG
to close a dialog and return to
the app home card:
{ renderActions: { action: {
navigations: [{ endNavigation: { action: "CLOSE_DIALOG" }}]
}}}
To collect information from users, you can also build sequential dialogs. To learn how to build sequential dialogs, see Open and respond to dialogs.
Related topics
- View Chat app samples that use app home.
- Open and respond to dialogs.