進階標記使用兩個類別來定義標記:
AdvancedMarkerElement
類別提供基本參數 (position
、
title
和 map
),而 PinElement
類別則包含進一步的選項
。下列程式碼片段顯示建立新 PinElement
的程式碼。
然後套用至標記
// Create a pin element.
const pin = new PinElement({
scale: 1.5,
});
// Create a marker and apply the element.
const marker = new AdvancedMarkerElement({
map,
position: { lat: 37.419, lng: -122.02 },
content: pin.element,
});
在使用 HTML 建立的地圖中,標記的基本參數是使用 宣告。
gmp-advanced-marker
HTML 元素;使用的任何自訂選項
PinElement
類別必須透過程式輔助方式套用。如要這麼做,您的程式碼必須
從 HTML 網頁擷取 gmp-advanced-marker
元素。下列
顯示程式碼片段,可用於查詢 gmp-advanced-marker
元素集合
然後反覆查看結果,以套用
PinElement
。
// Return an array of markers.
const advancedMarkers = [...document.querySelectorAll('gmp-advanced-marker')];
// Loop through the markers
for (let i = 0; i < advancedMarkers.length; i++) {
const pin = new PinElement({
scale: 2.0,
});
marker.appendChild(pin.element);
}
本頁說明如何透過下列方式自訂標記:
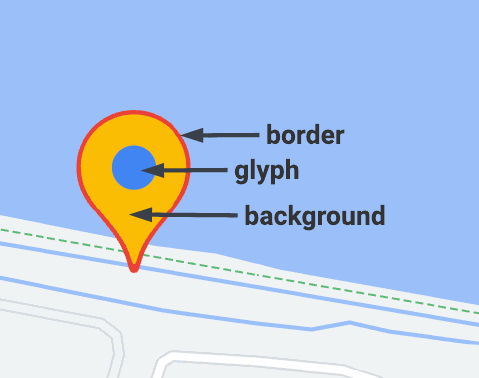
加入標題文字
標題文字會在滑鼠游標懸停在標記上時出現。標題文字清晰易讀 由螢幕閱讀器朗讀
如要透過程式輔助方式新增標題文字,請使用 AdvancedMarkerElement.title
選項:
// Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: "Title text for the marker at lat: 37.419, lng: -122.03", });
如要在使用 HTML 建立的標記中加入標題文字,請使用 title
屬性:
<gmp-map center="43.4142989,-124.2301242" zoom="4" map-id="DEMO_MAP_ID" style="height: 400px" > <gmp-advanced-marker position="37.4220656,-122.0840897" title="Mountain View, CA" ></gmp-advanced-marker> <gmp-advanced-marker position="47.648994,-122.3503845" title="Seattle, WA" ></gmp-advanced-marker> </gmp-map>
縮放標記
如要縮放標記,請使用 scale
選項。
TypeScript
// Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, });
JavaScript
// Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, });
變更背景顏色
使用 PinElement.background
選項即可變更標記的背景顏色:
TypeScript
// Change the background color. const pinBackground = new PinElement({ background: '#FBBC04', }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, });
JavaScript
// Change the background color. const pinBackground = new PinElement({ background: "#FBBC04", }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, });
變更邊框顏色
使用 PinElement.borderColor
選項即可變更標記的邊框顏色:
TypeScript
// Change the border color. const pinBorder = new PinElement({ borderColor: '#137333', }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.03 }, content: pinBorder.element, });
JavaScript
// Change the border color. const pinBorder = new PinElement({ borderColor: "#137333", }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.03 }, content: pinBorder.element, });
變更字符顏色
使用 PinElement.glyphColor
選項即可變更標記的字符顏色:
TypeScript
// Change the glyph color. const pinGlyph = new PinElement({ glyphColor: 'white', }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.02 }, content: pinGlyph.element, });
JavaScript
// Change the glyph color. const pinGlyph = new PinElement({ glyphColor: "white", }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.02 }, content: pinGlyph.element, });
隱藏字符
將 PinElement.glyph
選項設為空白字串,即可隱藏標記字符:
TypeScript
// Hide the glyph. const pinNoGlyph = new PinElement({ glyph: '', }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.01 }, content: pinNoGlyph.element, });
JavaScript
// Hide the glyph. const pinNoGlyph = new PinElement({ glyph: "", }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.01 }, content: pinNoGlyph.element, });
或者,您也可以將 PinElement.glyphColor
設為使用 PinElement.background
的值。
這在視覺上有隱藏字符的效果。