This page shows you how to render geographic data in the KML
format, using GMUKMLParser
in
conjunction with GMUGeometryRenderer
. KML is a popular
format for rendering geographic data such as points, lines, and polygons.
The following screenshot shows some example KML data rendered on a map:
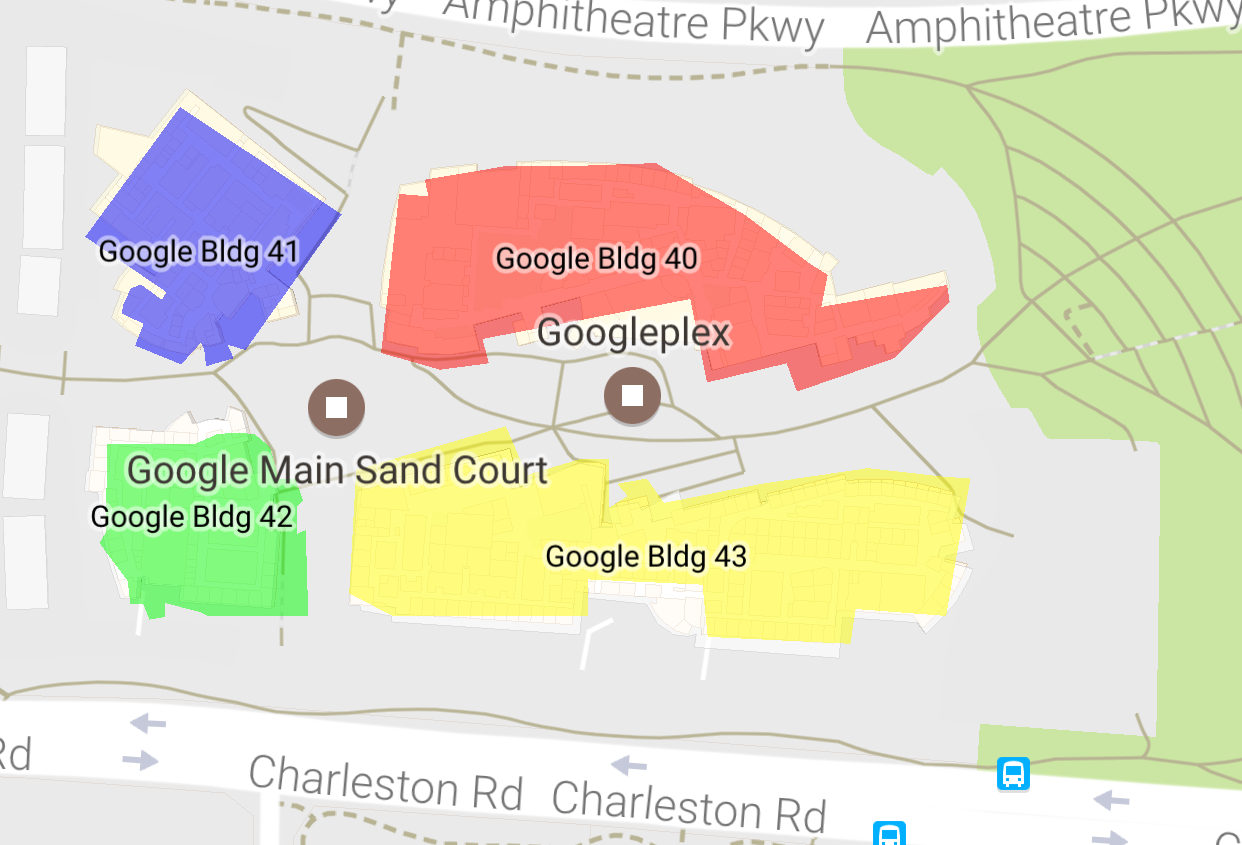
Prerequisites and notes
GMUKMLParser
is part of
the Maps SDK for iOS Utility Library. If you haven't yet set up
the library, follow the setup guide before reading the rest of this page.
For the full code sample, see the sample apps on GitHub.
Rendering KML data
To render KML data on a map, create a GMUKMLParser
with the
path to a KML resource (KML_Sample.kml
in this example). Then,
create a GMUGeometryRenderer
passing the GMUKMLParser
instance. Finally, call GMUGeometryRenderer.render()
. The
following code example shows rendering KML data on a map:
Swift
import GoogleMapsUtils class KML: NSObject { private var mapView: GMSMapView! func renderKml() { guard let path = Bundle.main.path(forResource: "KML_Sample", ofType: "kml") else { print("Invalid path") return } let url = URL(fileURLWithPath: path) let kmlParser = GMUKMLParser(url: url) kmlParser.parse() let renderer = GMUGeometryRenderer( map: mapView, geometries: kmlParser.placemarks, styles: kmlParser.styles ) renderer.render() } }
Objective-C
@import GoogleMapsUtils; @implementation KML { GMSMapView *_mapView; } - (void)renderKml { NSString *path = [[NSBundle mainBundle] pathForResource:@"KML_Sample" ofType:@"kml"]; NSURL *url = [NSURL fileURLWithPath:path]; GMUKMLParser *parser = [[GMUKMLParser alloc] initWithURL:url]; [parser parse]; GMUGeometryRenderer *renderer = [[GMUGeometryRenderer alloc] initWithMap:_mapView geometries:parser.placemarks styles:parser.styles]; [renderer render]; } @end