En esta página, se describe cómo tu app de Chat puede abrir diálogos para responder a los usuarios.
Los diálogos son interfaces con ventanas y basadas en tarjetas que se abren desde un espacio o mensaje de Chat. El diálogo y su contenido solo son visibles para el usuario que lo abrió.
Las apps de chat pueden usar diálogos para solicitar y recopilar información de los usuarios de Chat, incluidos los formularios de varios pasos. Para obtener más detalles sobre la creación de entradas de formularios, consulta Cómo recopilar y procesar información de los usuarios.
Requisitos previos
Node.js
Una app de Google Chat habilitada para funciones interactivas. Si quieres crear una app de chat interactiva con un servicio HTTP, completa esta guía de inicio rápido.
Python
Una app de Google Chat habilitada para funciones interactivas. Si quieres crear una app de chat interactiva con un servicio HTTP, completa esta guía de inicio rápido.
Java
Una app de Google Chat habilitada para funciones interactivas Para crear una app de Chat interactiva con un servicio HTTP, completa esta guía de inicio rápido.
Apps Script
Una app de Google Chat habilitada para funciones interactivas Para crear una app de Chat interactiva en Apps Script, completa esta guía de inicio rápido.
Cómo abrir un diálogo
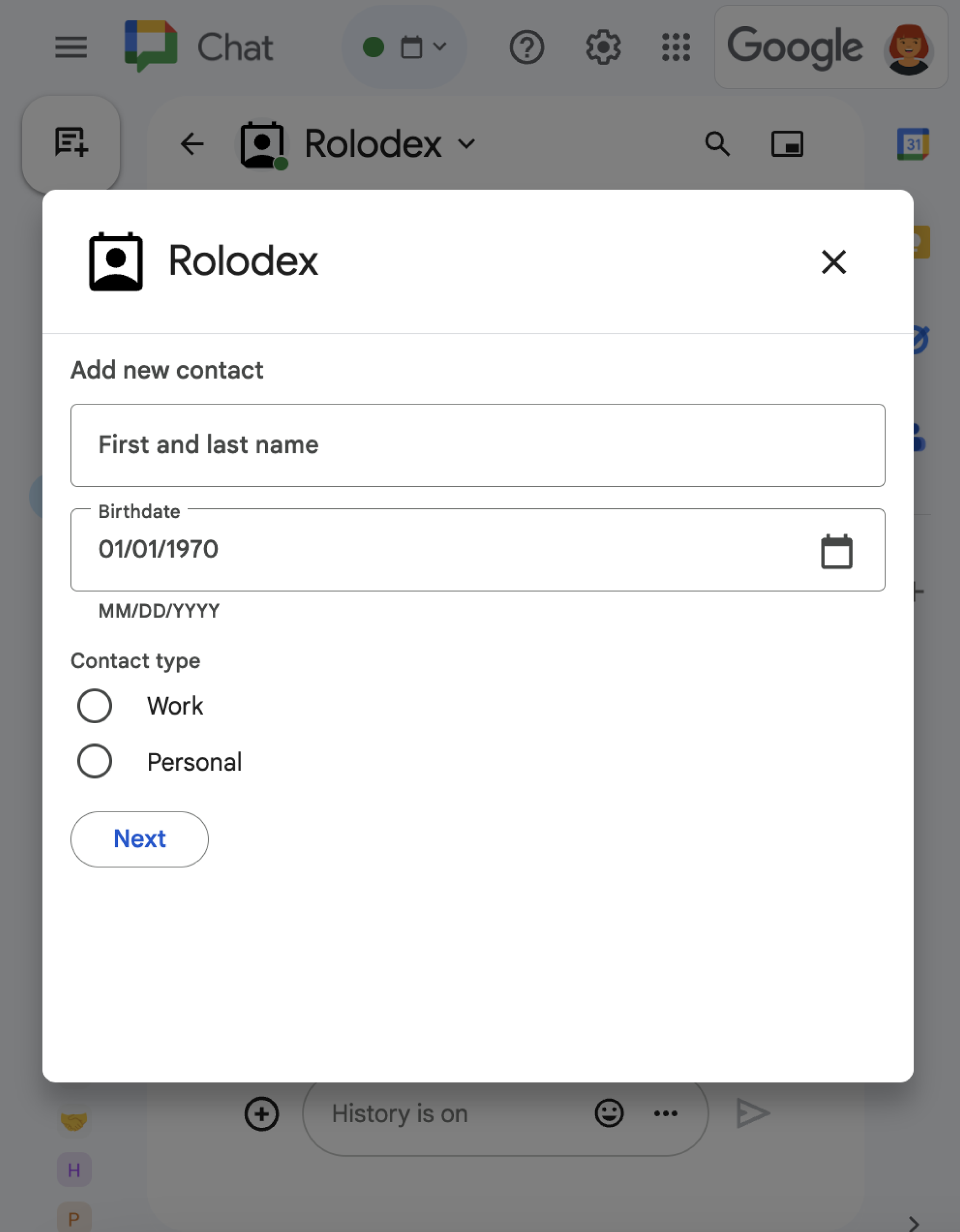
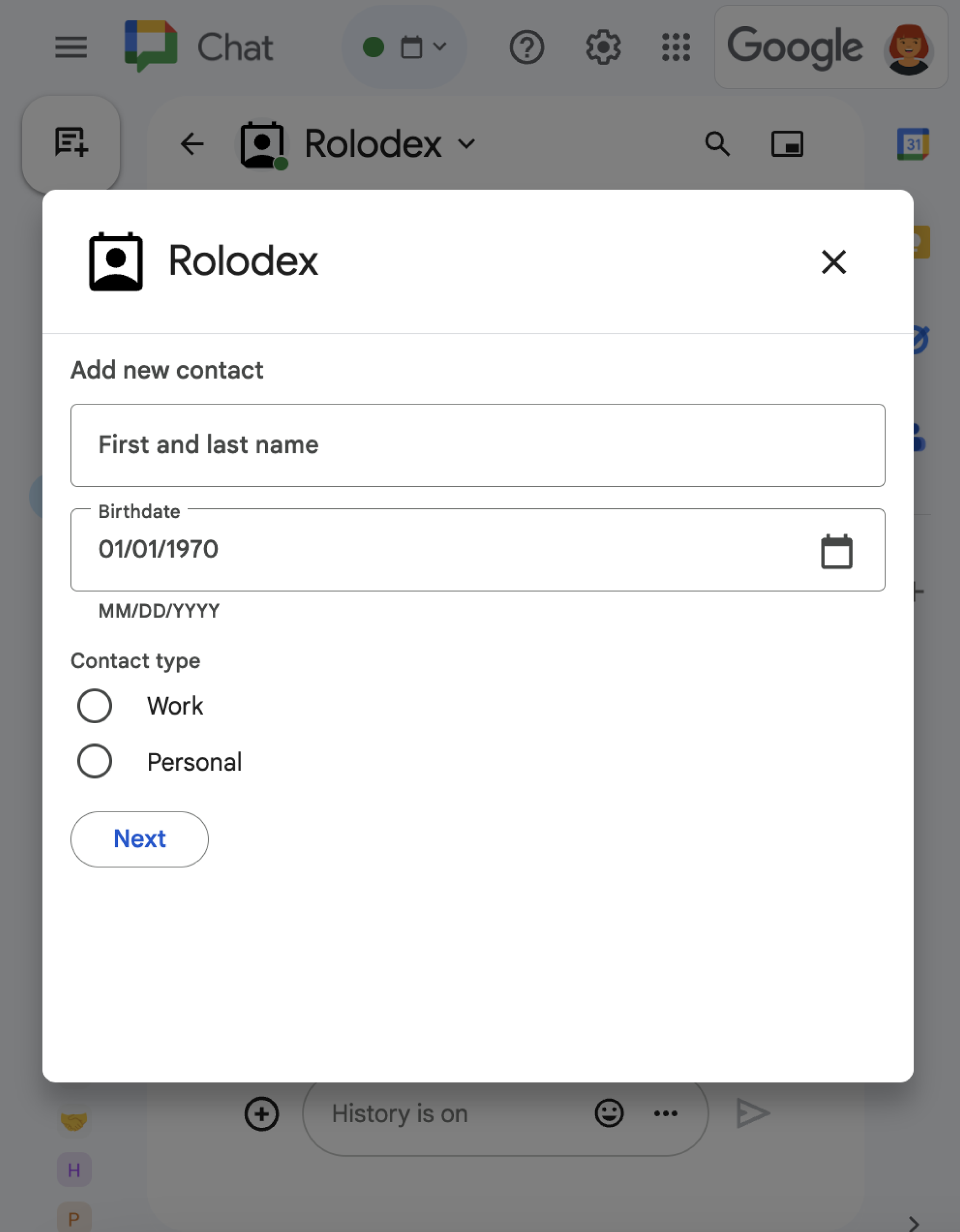
En esta sección, se explica cómo responder y configurar un diálogo de la siguiente manera:
- Activa la solicitud de diálogo a partir de una interacción del usuario.
- Para controlar la solicitud, muestra y abre un diálogo.
- Después de que los usuarios envíen información, cierra el diálogo o muestra otro para procesar el envío.
Activa una solicitud de diálogo
Una app de chat solo puede abrir diálogos para responder a una interacción del usuario, como un comando de barra o un clic en un botón de un mensaje en una tarjeta.
Para responder a los usuarios con un diálogo, una app de Chat debe compilar una interacción que active la solicitud de diálogo, como la siguiente:
- Responde a un comando de barra. Para activar la solicitud desde un comando de barra, debes marcar la casilla de verificación Abre un diálogo cuando configures el comando.
- Responde a un clic en un botón de un
mensaje,
como parte de una tarjeta o en la parte inferior del mensaje. Para activar la
solicitud desde un botón en un mensaje, configura la
acción
onClick
del botón configurando suinteraction
enOPEN_DIALOG
. - Responde a un clic en un botón en la página principal de la app de Chat. Para obtener información sobre cómo abrir diálogos desde páginas principales, consulta Cómo compilar una página principal para tu app de Google Chat.
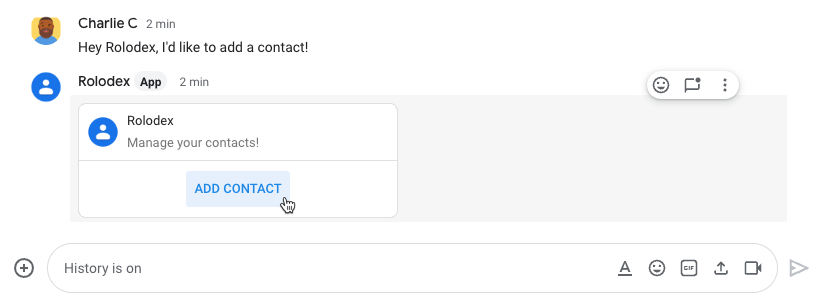
/addContact
. El mensaje también incluye un botón en el que los usuarios pueden hacer clic para activar el comando.
En la siguiente muestra de código, se muestra cómo activar una solicitud de diálogo desde un botón en un mensaje de tarjeta. Para abrir el diálogo, el campo button.interaction
se establece en OPEN_DIALOG
:
Node.js
Python
Java
Apps Script
En este ejemplo, se muestra un mensaje de tarjeta mediante la devolución de un JSON de tarjeta. También puedes usar el servicio de tarjetas de Apps Script.
Abre el diálogo inicial
Cuando un usuario activa una solicitud de diálogo, tu app de Chat recibe un evento de interacción, representado como un tipo event
en la API de Chat. Si la interacción activa una solicitud de diálogo, el campo dialogEventType
del evento se establece en REQUEST_DIALOG
.
Para abrir un diálogo, tu app de Chat puede responder a la solicitud mostrando un objeto actionResponse
con el type
establecido en DIALOG
y el objeto Message
. Para especificar el contenido del diálogo, incluye los siguientes objetos:
- Un objeto
actionResponse
, con sutype
configurado comoDIALOG
- Un objeto
dialogAction
. El campobody
contiene los elementos de la interfaz de usuario (IU) que se mostrarán en la tarjeta, incluidos uno o mássections
de widgets. Para recopilar información de los usuarios, puedes especificar widgets de entrada de formulario y un widget de botón. Para obtener más información sobre el diseño de entradas de formularios, consulta Cómo recopilar y procesar información de los usuarios.
En la siguiente muestra de código, se muestra cómo una app de Chat muestra una respuesta que abre un diálogo:
Node.js
Python
Java
Apps Script
En este ejemplo, se muestra un mensaje de tarjeta mediante la devolución de un JSON de tarjeta. También puedes usar el servicio de tarjetas de Apps Script.
Controla el envío del diálogo
Cuando los usuarios hacen clic en un botón que envía un diálogo, la app de Chat recibe un evento de interacción CARD_CLICKED
en el que dialogEventType
es SUBMIT_DIALOG
.
Tu app de Chat debe controlar el evento de interacción haciendo una de las siguientes acciones:
- Devuelve otro diálogo para propagar otra tarjeta o formulario.
- Cierra el diálogo después de validar los datos que envió el usuario y, de manera opcional, envía un mensaje de confirmación.
Opcional: Devuelve otro diálogo
Después de que los usuarios envían el diálogo inicial, las apps de Chat pueden mostrar uno o más diálogos adicionales para ayudarlos a revisar la información antes de enviarla, completar formularios de varios pasos o propagar el contenido de los formularios de forma dinámica.
Para procesar los datos que ingresan los usuarios, la app de Chat usa el objeto event.common.formInputs
. Para obtener más información sobre cómo recuperar valores de widgets de entrada, consulta
Cómo recopilar y procesar información de los usuarios.
Para realizar un seguimiento de los datos que los usuarios ingresan desde el diálogo inicial, debes agregar parámetros al botón que abre el siguiente diálogo. Para obtener más información, consulta Transfiere datos a otra tarjeta.
En este ejemplo, una app de Chat abre un diálogo inicial que lleva a un segundo diálogo para confirmar antes de enviar:
Node.js
Python
Java
Apps Script
En este ejemplo, se muestra el JSON de la tarjeta para enviar un mensaje de tarjeta. También puedes usar el servicio de tarjetas de Apps Script.
Cerrar el diálogo
Cuando los usuarios hacen clic en un botón de un diálogo, tu app de chat ejecuta la acción asociada y le proporciona al objeto del evento la siguiente información:
eventType
esCARD_CLICKED
.dialogEventType
esSUBMIT_DIALOG
.
La app de Chat debería mostrar un objeto ActionResponse
con su type
establecido en DIALOG
y dialogAction
.
Opcional: Cómo mostrar una notificación
Cuando cierres el diálogo, también puedes mostrar una notificación de texto.
La app de Chat puede responder con una notificación de éxito o error mostrando una ActionResponse
con actionStatus
configurado.
En el siguiente ejemplo, se verifica que los parámetros sean válidos y se cierra el diálogo con una notificación de texto según el resultado:
Node.js
Python
Java
Apps Script
En este ejemplo, se muestra un mensaje de tarjeta mediante la devolución de un JSON de tarjeta. También puedes usar el servicio de tarjetas de Apps Script.
Para obtener información sobre cómo pasar parámetros entre diálogos, consulta Transfiere datos a otra tarjeta.
Opcional: Envía un mensaje de confirmación
Cuando cierres el diálogo, también podrás enviar un mensaje nuevo o actualizar uno existente.
Para enviar un mensaje nuevo, muestra un objeto ActionResponse
con type
establecido en NEW_MESSAGE
. En el siguiente ejemplo, se cierra el diálogo con una notificación de texto y un mensaje de texto de confirmación:
Node.js
Python
Java
Apps Script
En este ejemplo, se muestra un mensaje de tarjeta mediante la devolución de un JSON de tarjeta. También puedes usar el servicio de tarjetas de Apps Script.
Para actualizar un mensaje, muestra un objeto actionResponse
que contenga el mensaje actualizado y establece type
en uno de los siguientes valores:
UPDATE_MESSAGE
: Actualiza el mensaje que activó la solicitud de diálogo.UPDATE_USER_MESSAGE_CARDS
: Actualiza la tarjeta desde una vista previa del vínculo.
Solucionar problemas
Cuando una app de Google Chat o una tarjeta muestran un error, la interfaz de Chat muestra un mensaje que dice "Se produjo un error". o "No se puede procesar tu solicitud". A veces, la IU de Chat no muestra ningún mensaje de error, pero la app o la tarjeta de Chat producen un resultado inesperado. Por ejemplo, es posible que no aparezca un mensaje de la tarjeta.
Aunque es posible que no se muestre un mensaje de error en la IU de Chat, los mensajes de error descriptivos y los datos de registro están disponibles para ayudarte a corregir errores cuando se activa el registro de errores de las apps de Chat. Si necesitas ayuda para ver, depurar y corregir errores, consulta Cómo solucionar problemas y corregir errores de Google Chat.
Temas relacionados
- Consulta la muestra de Administrador de contactos, que es una app de chat que usa diálogos para recopilar información de contacto.
- Abrir diálogos desde la página principal de la app de Google Chat
- Cómo configurar y responder comandos de barra
- Procesa la información que ingresan los usuarios