The autocomplete service in the Places SDK for Android returns place predictions in response to user search queries. As the user types, the autocomplete service returns suggestions for places such as businesses, addresses, plus codes, and points of interest.
You can add autocomplete to your app in the following ways:
- Add an autocomplete widget to save development time and ensure a consistent user experience.
- Get place predictions programmatically to create a customized user experience.
Add an autocomplete widget
The autocomplete widget is a search dialog with built-in autocomplete
functionality. As a user enters search terms, the widget presents a list of
predicted places to choose from. When the user makes a selection, a
Place
instance is returned, which your app can then use to get details about the
selected place.
There are two options for adding the autocomplete widget to your app:
- Option 1: Embed an
AutocompleteSupportFragment
. - Option 2: Use an intent to launch the autocomplete activity.
Option 1: Embed an AutocompleteSupportFragment
To add an AutocompleteSupportFragment
to your app, take the following steps:
- Add a fragment to your activity's XML layout.
- Add a listener to your activity or fragment.
Add AutocompleteSupportFragment to an activity
To add AutocompleteSupportFragment
to an activity, add a new fragment to an
XML layout. For example:
<fragment android:id="@+id/autocomplete_fragment"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:name="com.google.android.libraries.places.widget.AutocompleteSupportFragment"
/>
- By default, the fragment has no border or background. To provide a consistent visual appearance, nest the fragment within another layout element such as a CardView.
- If you are using the Autocomplete fragment, and need to override
onActivityResult
, you must callsuper.onActivityResult
, otherwise the fragment will not function properly.
Add a PlaceSelectionListener to an activity
The PlaceSelectionListener
handles returning a place in response to the user's
selection. The following code shows creating a reference to the fragment and
adding a listener to your AutocompleteSupportFragment
:
Kotlin
// Initialize the AutocompleteSupportFragment. val autocompleteFragment = supportFragmentManager.findFragmentById(R.id.autocomplete_fragment) as AutocompleteSupportFragment // Specify the types of place data to return. autocompleteFragment.setPlaceFields(listOf(Place.Field.ID, Place.Field.NAME)) // Set up a PlaceSelectionListener to handle the response. autocompleteFragment.setOnPlaceSelectedListener(object : PlaceSelectionListener { override fun onPlaceSelected(place: Place) { // TODO: Get info about the selected place. Log.i(TAG, "Place: ${place.name}, ${place.id}") } override fun onError(status: Status) { // TODO: Handle the error. Log.i(TAG, "An error occurred: $status") } })
Java
// Initialize the AutocompleteSupportFragment. AutocompleteSupportFragment autocompleteFragment = (AutocompleteSupportFragment) getSupportFragmentManager().findFragmentById(R.id.autocomplete_fragment); // Specify the types of place data to return. autocompleteFragment.setPlaceFields(Arrays.asList(Place.Field.ID, Place.Field.NAME)); // Set up a PlaceSelectionListener to handle the response. autocompleteFragment.setOnPlaceSelectedListener(new PlaceSelectionListener() { @Override public void onPlaceSelected(@NonNull Place place) { // TODO: Get info about the selected place. Log.i(TAG, "Place: " + place.getName() + ", " + place.getId()); } @Override public void onError(@NonNull Status status) { // TODO: Handle the error. Log.i(TAG, "An error occurred: " + status); } });
Option 2: Use an intent to launch the autocomplete activity
If you want your app to use a different navigational flow (for example, to trigger the autocomplete experience from an icon rather than a search field), your app can launch autocomplete by using an intent.
To launch the autocomplete widget using an intent, follow these steps:
- Use
Autocomplete.IntentBuilder
to create an intent, passing the desiredAutocomplete
mode. - Define an activity result launcher
registerForActivityResult
that can be used to launch the intent and handle the user's selected place prediction in the result.
Create an autocomplete intent
The example below uses
Autocomplete.IntentBuilder
to create an intent to launch the autocomplete widget as an intent:
Kotlin
// Set the fields to specify which types of place data to // return after the user has made a selection. val fields = listOf(Place.Field.ID, Place.Field.NAME) // Start the autocomplete intent. val intent = Autocomplete.IntentBuilder(AutocompleteActivityMode.FULLSCREEN, fields) .build(this) startAutocomplete.launch(intent)
Java
// Set the fields to specify which types of place data to // return after the user has made a selection. List<Place.Field> fields = Arrays.asList(Place.Field.ID, Place.Field.NAME); // Start the autocomplete intent. Intent intent = new Autocomplete.IntentBuilder(AutocompleteActivityMode.FULLSCREEN, fields) .build(this); startAutocomplete.launch(intent);
When using an intent to launch the autocomplete widget, you can choose from overlay or full-screen display modes. The following screenshots show each display mode respectively:
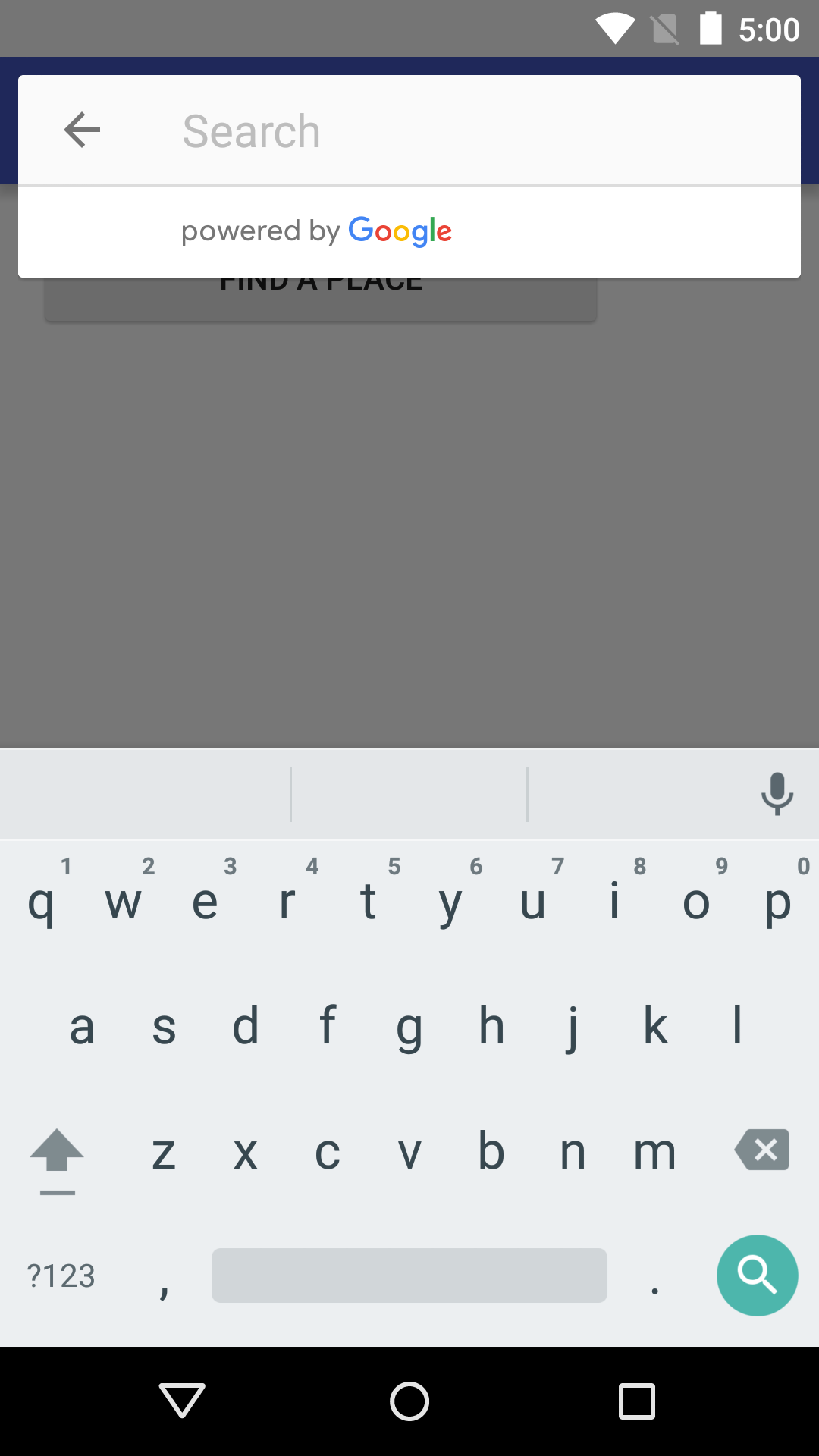
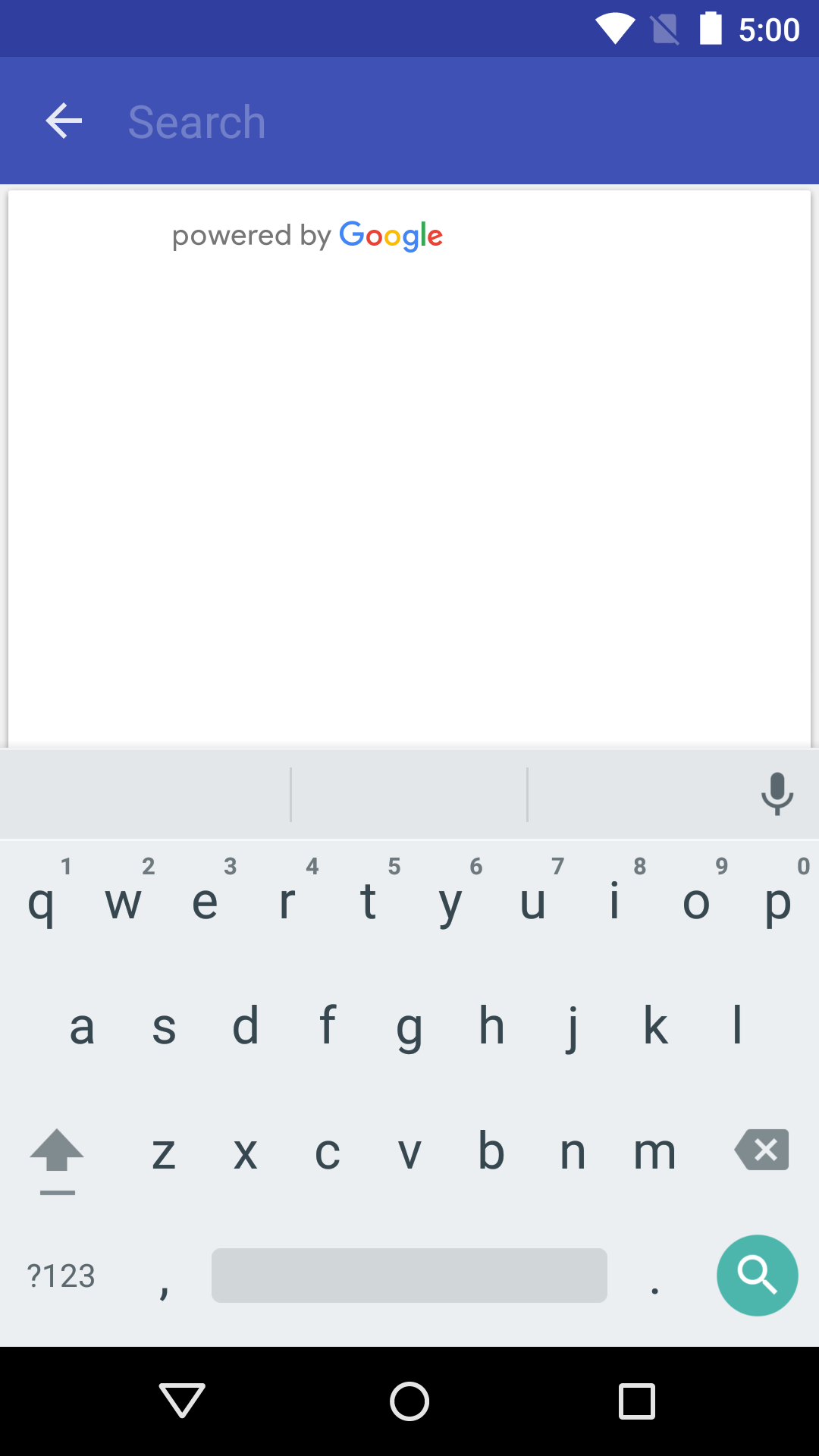
Register a callback for the intent result
To receive a notification when the user has selected a place, define a
registerForActivityResult()
launcher, which launches the activity and also handles the
result as shown in the following example. If the user selected a prediction, it
will be delivered in the intent contained in the result object. Since the intent
was built by Autocomplete.IntentBuilder
, the method
Autocomplete.getPlaceFromIntent()
can extract the Place object from it.
Kotlin
private val startAutocomplete = registerForActivityResult(ActivityResultContracts.StartActivityForResult()) { result: ActivityResult -> if (result.resultCode == Activity.RESULT_OK) { val intent = result.data if (intent != null) { val place = Autocomplete.getPlaceFromIntent(intent) Log.i( TAG, "Place: ${place.name}, ${place.id}" ) } } else if (result.resultCode == Activity.RESULT_CANCELED) { // The user canceled the operation. Log.i(TAG, "User canceled autocomplete") } }
Java
private final ActivityResultLauncher<Intent> startAutocomplete = registerForActivityResult( new ActivityResultContracts.StartActivityForResult(), result -> { if (result.getResultCode() == Activity.RESULT_OK) { Intent intent = result.getData(); if (intent != null) { Place place = Autocomplete.getPlaceFromIntent(intent); Log.i(TAG, "Place: ${place.getName()}, ${place.getId()}"); } } else if (result.getResultCode() == Activity.RESULT_CANCELED) { // The user canceled the operation. Log.i(TAG, "User canceled autocomplete"); } });
Getting place predictions programmatically
You can create a custom search UI as an alternative to the UI provided by the
autocomplete widget. To do this, your app must get place predictions
programmatically. Your app can get a list of predicted place names and/or
addresses from the autocomplete API by calling
PlacesClient.findAutocompletePredictions()
,
passing a
FindAutocompletePredictionsRequest
object with the following parameters:
- Required: A
query
string containing the text typed by the user. - Recommended: A
AutocompleteSessionToken
, which groups the query and selection phases of a user search into a discrete session for billing purposes. The session begins when the user starts typing a query, and concludes when they select a place. - Recommended: A
RectangularBounds
object, which specifies latitude and longitude bounds to constrain results to the specified region. - Optional: One or more two-letter country codes (ISO 3166-1 Alpha-2), indicating the country or countries to which results should be restricted.
Optional: A
TypeFilter
, which you can use to restrict the results to the specified place type. The following place types are supported:TypeFilter.GEOCODE
– Returns only geocoding results, rather than businesses. Use this request to disambiguate results where the specified location may be indeterminate.TypeFilter.ADDRESS
– Returns only autocomplete results with a precise address. Use this type when you know the user is looking for a fully specified address.TypeFilter.ESTABLISHMENT
– Returns only places that are businesses.TypeFilter.REGIONS
– Returns only places that match one of the following types:LOCALITY
SUBLOCALITY
POSTAL_CODE
COUNTRY
ADMINISTRATIVE_AREA_LEVEL_1
ADMINISTRATIVE_AREA_LEVEL_2
TypeFilter.CITIES
– Returns only results matchingLOCALITY
orADMINISTRATIVE_AREA_LEVEL_3
.
Optional: A
LatLng
specifying the location of origin for the request. When you callsetOrigin()
, the service returns distance in meters (distanceMeters
) from the specified origin, for each autocomplete prediction in the response.
For information about place types, see the guide to place types.
The example below shows a complete call to
PlacesClient.findAutocompletePredictions()
.
Kotlin
// Create a new token for the autocomplete session. Pass this to FindAutocompletePredictionsRequest, // and once again when the user makes a selection (for example when calling fetchPlace()). val token = AutocompleteSessionToken.newInstance() // Create a RectangularBounds object. val bounds = RectangularBounds.newInstance( LatLng(-33.880490, 151.184363), LatLng(-33.858754, 151.229596) ) // Use the builder to create a FindAutocompletePredictionsRequest. val request = FindAutocompletePredictionsRequest.builder() // Call either setLocationBias() OR setLocationRestriction(). .setLocationBias(bounds) //.setLocationRestriction(bounds) .setOrigin(LatLng(-33.8749937, 151.2041382)) .setCountries("AU", "NZ") .setTypesFilter(listOf(PlaceTypes.ADDRESS)) .setSessionToken(token) .setQuery(query) .build() placesClient.findAutocompletePredictions(request) .addOnSuccessListener { response: FindAutocompletePredictionsResponse -> for (prediction in response.autocompletePredictions) { Log.i(TAG, prediction.placeId) Log.i(TAG, prediction.getPrimaryText(null).toString()) } }.addOnFailureListener { exception: Exception? -> if (exception is ApiException) { Log.e(TAG, "Place not found: ${exception.statusCode}") } }
Java
// Create a new token for the autocomplete session. Pass this to FindAutocompletePredictionsRequest, // and once again when the user makes a selection (for example when calling fetchPlace()). AutocompleteSessionToken token = AutocompleteSessionToken.newInstance(); // Create a RectangularBounds object. RectangularBounds bounds = RectangularBounds.newInstance( new LatLng(-33.880490, 151.184363), new LatLng(-33.858754, 151.229596)); // Use the builder to create a FindAutocompletePredictionsRequest. FindAutocompletePredictionsRequest request = FindAutocompletePredictionsRequest.builder() // Call either setLocationBias() OR setLocationRestriction(). .setLocationBias(bounds) //.setLocationRestriction(bounds) .setOrigin(new LatLng(-33.8749937, 151.2041382)) .setCountries("AU", "NZ") .setTypesFilter(Arrays.asList(PlaceTypes.ADDRESS)) .setSessionToken(token) .setQuery(query) .build(); placesClient.findAutocompletePredictions(request).addOnSuccessListener((response) -> { for (AutocompletePrediction prediction : response.getAutocompletePredictions()) { Log.i(TAG, prediction.getPlaceId()); Log.i(TAG, prediction.getPrimaryText(null).toString()); } }).addOnFailureListener((exception) -> { if (exception instanceof ApiException) { ApiException apiException = (ApiException) exception; Log.e(TAG, "Place not found: " + apiException.getStatusCode()); } });
The API returns an
FindAutocompletePredictionsResponse
in a
Task
. The FindAutocompletePredictionsResponse
contains a list of
AutocompletePrediction
objects representing predicted places. The list may be empty, if there is no
known place corresponding to the query and the filter criteria.
For each predicted place, you can call the following methods to retrieve place details:
getFullText(CharacterStyle)
returns the full text of a place description. This is a combination of the primary and secondary text. Example: "Eiffel Tower, Avenue Anatole France, Paris, France". In addition, this method lets you highlight the sections of the description that match the search with a style of your choice, usingCharacterStyle
. TheCharacterStyle
parameter is optional. Set it to null if you don't need any highlighting.getPrimaryText(CharacterStyle)
returns the main text describing a place. This is usually the name of the place. Examples: "Eiffel Tower", and "123 Pitt Street".getSecondaryText(CharacterStyle)
returns the subsidiary text of a place description. This is useful, for example, as a second line when showing autocomplete predictions. Examples: "Avenue Anatole France, Paris, France", and "Sydney, New South Wales".getPlaceId()
returns the place ID of the predicted place. A place ID is a textual identifier that uniquely identifies a place, which you can use to retrieve thePlace
object again later. For more information about place IDs in Places SDK for Android, see the Place Details. For general information about place IDs, see the Place ID overview.getPlaceTypes()
returns the list of place types associated with this place.getDistanceMeters()
returns the straight-line distance in meters between this place and the origin specified in the request.
Session tokens
Session tokens group the query and selection phases of a user autocomplete search into a discrete session for billing purposes. The session begins when the user starts typing a query, and concludes when they select a place. Each session can have multiple queries, followed by one place selection. Once a session has concluded, the token is no longer valid; your app must generate a fresh token for each session. We recommend using session tokens for all programmatic autocomplete sessions (when you embed a fragment, or launch autocomplete using an intent, the API takes care of this automatically).
The Places SDK for Android uses a
AutocompleteSessionToken
to identify each session. Your app should pass a new session token upon
beginning each new session, then pass that same token, along with a Place ID, in
the subsequent call to
fetchPlace()
to retrieve Place Details for the place that was selected by the user.
Learn more about session tokens.
Constrain autocomplete results
You can constrain autocomplete results to a specific geographic region, and/or
filter the results to one or more place types, or to up to five countries. You
can apply these constraints to the autocomplete activity,
AutocompleteSupportFragment
, and programmatic autocomplete APIs.
To constrain results, do the following:
- To prefer results within the defined region, call
setLocationBias()
(some results from outside the defined region may still be returned). - To only show results within the defined region, call
setLocationRestriction()
(only results within the defined region will be returned). - To return only results that conform to a particular place type, call
setTypesFilter()
(for example, specifyingTypeFilter.ADDRESS
will return only results with a precise address). - To return only results within up to five specified countries, call
setCountries()
. Countries must be passed as a two-character, ISO 3166-1 Alpha-2 compatible country code.
Bias results to a specific region
To bias autocomplete results to a specific geographic region, call
setLocationBias()
, passing a
RectangularBounds
.
The following code example shows calling setLocationBias()
on a fragment
instance to bias its autocomplete suggestions to a region of Sydney, Australia.
Kotlin
autocompleteFragment.setLocationBias( RectangularBounds.newInstance( LatLng(-33.880490, 151.184363), LatLng(-33.858754, 151.229596) ) )
Java
autocompleteFragment.setLocationBias(RectangularBounds.newInstance( new LatLng(-33.880490, 151.184363), new LatLng(-33.858754, 151.229596)));
Restrict results to a specific region
To restrict autocomplete results to a specific geographic region, call
setLocationRestriction()
, passing a
RectangularBounds
.
The following code example shows calling setLocationRestriction()
on a
fragment instance to bias its autocomplete suggestions to a region of Sydney,
Australia.
Kotlin
autocompleteFragment.setLocationRestriction( RectangularBounds.newInstance( LatLng(-33.880490, 151.184363), LatLng(-33.858754, 151.229596) ) )
Java
autocompleteFragment.setLocationRestriction(RectangularBounds.newInstance( new LatLng(-33.880490, 151.184363), new LatLng(-33.858754, 151.229596)));
Note: This restriction is only applied to entire routes, synthetic results located outside the rectangular bounds may be returned based on a route that overlaps with the location restrict.
Filter results by place types or type collection
You can restrict results from an autocomplete request so they only return a certain place type. Specify a filter using the place types or a type collection listed in Tables 1, 2, and 3 on Place Types. If nothing is specified, all types are returned.
To filter autocomplete results, call
setTypesFilter()
to set the filter.
To specify a type or type collection filter:
Call
setTypesFilter()
and specify up to five type values from Table 1 and Table 2 shown on Place Types. The type values are defined by the constants in PlaceTypes.Call
setTypesFilter()
and specify a type collection from Table 3 shown on Place Types. The collection values are defined by the constants in PlaceTypes.Only a single type from Table 3 is allowed in the request. If you specify a value from Table 3, you cannot specify a value from Table 1 or Table 2. If you do, then an error occurs.
The following code example calls setTypesFilter()
on an
AutocompleteSupportFragment
and specifies multiple type values.
Kotlin
autocompleteFragment.setTypesFilter(listOf("landmark", "restaurant", "store"))
Java
autocompleteFragment.setTypesFilter(Arrays.asList("landmark", "restaurant", "store"));
The following code example shows calling setTypesFilter()
on an
AutocompleteSupportFragment
to set a filter returning only results with a
precise address by specifying a type collection.
Kotlin
autocompleteFragment.setTypesFilter(listOf(PlaceTypes.ADDRESS))
Java
autocompleteFragment.setTypesFilter(Arrays.asList(PlaceTypes.ADDRESS, PlaceTypes.ESTABLISHMENT));
The following code example shows calling setTypesFilter()
on an
IntentBuilder
to set a filter returning only results with a precise address by
specifying a type collection.
Kotlin
val intent = Autocomplete.IntentBuilder(AutocompleteActivityMode.FULLSCREEN, fields) .setTypesFilter(listOf(PlaceTypes.ADDRESS)) .build(this)
Java
Intent intent = new Autocomplete.IntentBuilder( AutocompleteActivityMode.FULLSCREEN, fields) .setTypesFilter(Arrays.asList(PlaceTypes.ADDRESS)) .build(this);
Filter results by country
To filter autocomplete results to up to five countries, call
setCountries()
to set the country code.
Then, pass the filter to a fragment or intent. Countries must be passed as a
two-character, ISO 3166-1 Alpha-2 compatible country
code.
The following code example shows calling setCountries()
on an
AutocompleteSupportFragment
, to set a filter returning only results within the
specified countries.
Kotlin
autocompleteFragment.setCountries("AU", "NZ")
Java
autocompleteFragment.setCountries("AU", "NZ");
Usage limits
Your usage of the Places API, including the Places SDK for Android, is no longer limited to a maximum number of requests per day (QPD). However, the following usage limits still apply:
- Rate limit is 6,000 QPM (requests per minute). It is calculated as the sum of client-side and server-side requests for all applications using the credentials of the same project.
Display attributions in your app
- If your app uses the autocomplete service programmatically, your UI must either display a 'Powered by Google' attribution, or appear within a Google-branded map.
- If your app uses the autocomplete widget no additional action is required (the required attribution is displayed by default).
- If you retrieve and display additional place information after getting a place by ID, you must display third-party attributions too.
For more details, see the documentation on attributions.
Place Autocomplete optimization
This section describes best practices to help you make the most of the Place Autocomplete service.
Here are some general guidelines:
- The quickest way to develop a working user interface is to use the Maps JavaScript API Autocomplete widget, Places SDK for Android Autocomplete widget, or Places SDK for iOS Autocomplete UI control
- Develop an understanding of essential Place Autocomplete data fields from the start.
- Location biasing and location restriction fields are optional but can have a significant impact on autocomplete performance.
- Use error handling to make sure your app degrades gracefully if the API returns an error.
- Make sure your app handles when there is no selection and offers users a way to continue.
Cost optimization best practices
Basic cost optimization
To optimize the cost of using the Place Autocomplete service, use field masks in Place Details and Place Autocomplete widgets to return only the place data fields you need.
Advanced cost optimization
Consider programmatic implementation of Place Autocomplete in order to access Per Request pricing and request Geocoding API results about the selected place instead of Place Details. Per Request pricing paired with Geocoding API is more cost-effective than Per Session (session-based) pricing if both of the following conditions are met:
- If you only need the latitude/longitude or address of the user's selected place, the Geocoding API delivers this information for less than a Place Details call.
- If users select an autocomplete prediction within an average of four Autocomplete predictions requests or fewer, Per Request pricing may be more cost-effective than Per Session pricing.
Does your application require any information other than the address and latitude/longitude of the selected prediction?
Yes, needs more details
Use session-based Place Autocomplete with Place Details.
Since your application requires Place Details such as the place name, business status, or opening hours, your implementation of Place Autocomplete should use a session token (programmatically or built into the JavaScript, Android, or iOS widgets) for a total cost of $0.017 per session plus applicable Places Data SKUs depending on which place data fields you request.1
Widget implementation
Session management is automatically built into the JavaScript, Android, or iOS widgets. This includes both the Place Autocomplete requests and the Place Details request on the selected prediction. Be sure to specify the fields
parameter in order to ensure you are only requesting the
place data fields you need.
Programmatic implementation
Use a session token with your Place Autocomplete requests. When requesting Place Details about the selected prediction, include the following parameters:
- The place ID from the Place Autocomplete response
- The session token used in the Place Autocomplete request
- The
fields
parameter specifying the place data fields you need
No, needs only address and location
Geocoding API could be a more cost-effective option than Place Details for your application, depending on the performance of your Place Autocomplete usage. Every application's Autocomplete efficiency varies depending on what users are entering, where the application is being used, and whether performance optimization best practices have been implemented.
In order to answer the following question, analyze how many characters a user types on average before selecting a Place Autocomplete prediction in your application.
Do your users select a Place Autocomplete prediction in four or fewer requests, on average?
Yes
Implement Place Autocomplete programmatically without session tokens and call Geocoding API on the selected place prediction.
Geocoding API delivers addresses and latitude/longitude coordinates for $0.005 per request. Making four Place Autocomplete - Per Request requests costs $0.01132 so the total cost of four requests plus a Geocoding API call about the selected place prediction would be $0.01632 which is less than the Per Session Autocomplete price of $0.017 per session.1
Consider employing performance best practices to help your users get the prediction they're looking for in even fewer characters.
No
Use session-based Place Autocomplete with Place Details.
Since the average number of requests you expect to make before a user selects a Place Autocomplete prediction exceeds the cost of Per Session pricing, your implementation of Place Autocomplete should use a session token for both the Place Autocomplete requests and the associated Place Details request for a total cost of $0.017 per session.1
Widget implementation
Session management is automatically built into the JavaScript, Android, or iOS widgets. This includes both the Place Autocomplete requests and the Place Details request on the selected prediction. Be sure to specify the fields
parameter in order to ensure you are only requesting Basic Data fields.
Programmatic implementation
Use a session token with your Place Autocomplete requests. When requesting Place Details about the selected prediction, include the following parameters:
- The place ID from the Place Autocomplete response
- The session token used in the Place Autocomplete request
- The
fields
parameter specifying Basic Data fields such as address and geometry
Consider delaying Place Autocomplete requests
You can employ strategies such as delaying a Place Autocomplete request until the user has typed in the first three or four characters so that your application makes fewer requests. For example, making Place Autocomplete requests for each character after the user has typed the third character means that if the user types seven characters then selects a prediction for which you make one Geocoding API request, the total cost would be $0.01632 (4 * $0.00283 Autocomplete Per Request + $0.005 Geocoding).1
If delaying requests can get your average programmatic request below four, you can follow the guidance for performant Place Autocomplete with Geocoding API implementation. Note that delaying requests can be perceived as latency by the user who might be expecting to see predictions with every new keystroke.
Consider employing performance best practices to help your users get the prediction they're looking for in fewer characters.
-
Costs listed here are in USD. Please refer to the Google Maps Platform Billing page for full pricing information.
Performance best practices
The following guidelines describe ways to optimize Place Autocomplete performance:
- Add country restrictions, location biasing, and (for programmatic implementations) language preference to your Place Autocomplete implementation. Language preference is not needed with widgets since they pick language preferences from the user's browser or mobile device.
- If Place Autocomplete is accompanied by a map, you can bias location by map viewport.
- In situations when a user does not choose one of the Autocomplete predictions, generally
because none of those predictions are the desired result-address, you can reuse the original
user input to attempt to get more relevant results:
- If you expect the user to enter only address information, reuse the original user input in a call to the Geocoding API.
- If you expect the user to enter queries for a specific place by name or address, use a Find Place request. If results are only expected in a specific region, use location biasing.
- Users inputting subpremise addresses, such as addresses for specific units or apartments within a building. For example, the Czech address "Stroupežnického 3191/17, Praha" yields a partial prediction in Place Autocomplete.
- Users inputting addresses with road-segment prefixes like "23-30 29th St, Queens" in New York City or "47-380 Kamehameha Hwy, Kaneohe" on the island of Kauai in Hawai'i.
Troubleshooting
Although a wide variety of errors can occur, the majority of the errors your app is likely to experience are usually caused by configuration errors (for example, the wrong API key was used, or the API key was configured incorrectly), or quota errors (your app has exceeded its quota). See Usage Limits for more information about quotas.
Errors that occur in the use of the autocomplete controls are returned in the
onActivityResult()
callback. Call Autocomplete.getStatus()
to get the status
message for the result.