Auf dieser Seite wird beschrieben, wie Ihre Chat-App Dialogfelder öffnen kann, um auf Nutzer zu reagieren.
Dialogfelder sind kartenbasierte Benutzeroberflächen, die über einen Chatbereich oder eine Nachricht geöffnet werden. Das Dialogfeld und sein Inhalt sind nur für den Nutzer sichtbar, der es geöffnet hat.
Chat-Apps können Dialogfelder verwenden, um Informationen von Google Chat-Nutzern anzufordern und zu erheben, einschließlich mehrstufiger Formulare. Weitere Informationen zum Erstellen von Formulareingaben finden Sie unter Nutzerinformationen erfassen und verarbeiten.
Vorbereitung
Node.js
Eine Google Chat App mit aktivierten interaktiven Funktionen. Wenn Sie eine interaktive Chat-App mit einem HTTP-Dienst erstellen möchten, folgen Sie dieser Kurzanleitung.
Python
Eine Google Chat-App, für die interaktive Funktionen aktiviert sind. Wenn Sie eine interaktive Chat-App mit einem HTTP-Dienst erstellen möchten, folgen Sie dieser Kurzanleitung.
Java
Eine Google Chat App mit aktivierten interaktiven Funktionen. Führen Sie diese Kurzanleitung aus, um eine interaktive Chat-App mit einem HTTP-Dienst zu erstellen.
Apps Script
Eine Google Chat-App, für die interaktive Funktionen aktiviert sind. Wenn Sie eine interaktive Chat-App in Apps Script erstellen möchten, folgen Sie dieser Kurzanleitung.
Dialogfeld öffnen
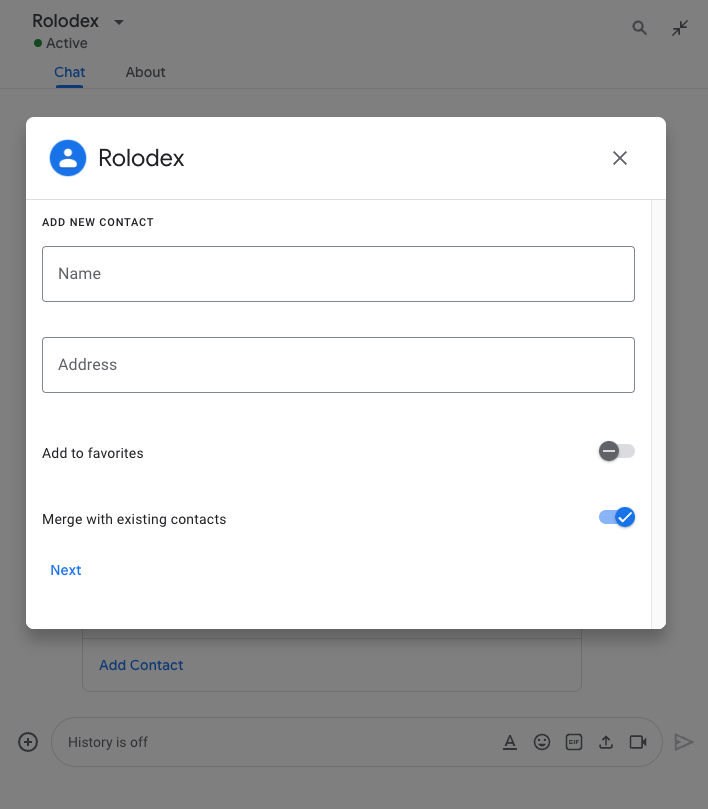
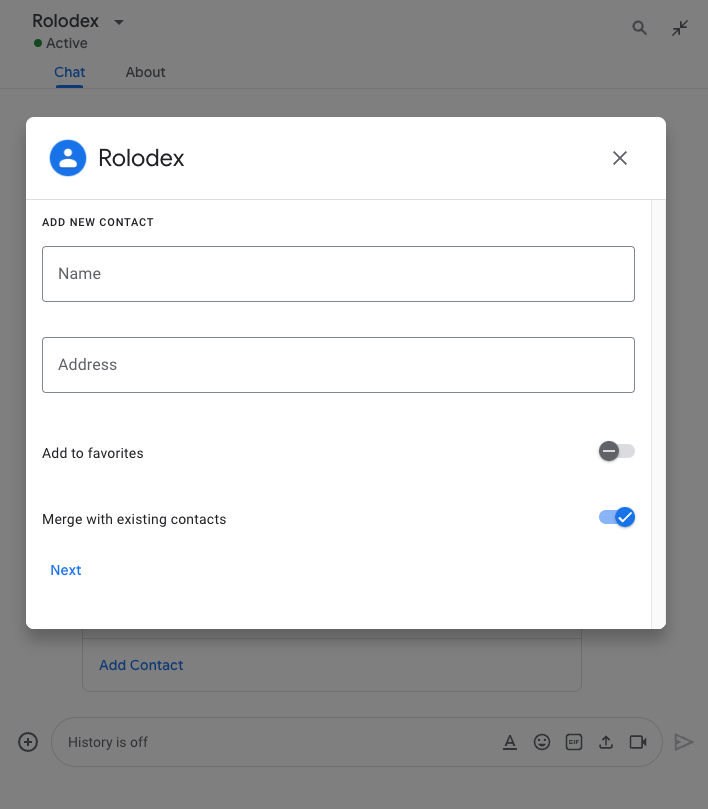
In diesem Abschnitt wird beschrieben, wie Sie antworten und einen Dialog einrichten:
- Auslösen der Dialoganfrage durch eine Nutzerinteraktion
- Verarbeiten Sie die Anfrage, indem Sie ein Dialogfeld zurückgeben und öffnen.
- Nachdem Nutzer Informationen gesendet haben, verarbeiten Sie die Einreichung, indem Sie entweder das Dialogfeld schließen oder ein anderes Dialogfeld zurückgeben.
Dialoganfrage auslösen
Eine Chat-App kann nur Dialogfelder öffnen, um auf eine Nutzerinteraktion zu reagieren, z. B. einen Schrägstrichbefehl oder einen Klick auf eine Schaltfläche in einer Nachricht auf einer Karte.
Damit eine Chat-App Nutzern mit einem Dialog antworten kann, muss sie eine Interaktion erstellen, die die Dialoganfrage auslöst. Beispiele:
- Auf einen Slash-Befehl reagieren Wenn du die Anfrage über einen Befehl mit einem Schrägstrich auslösen möchtest, musst du beim Konfigurieren des Befehls das Kästchen Öffnet ein Dialogfeld aktivieren.
- Sie können auf einen Schaltflächenklick in einer Nachricht reagieren, entweder als Teil einer Karte oder unten in der Nachricht. Wenn Sie die Anfrage über eine Schaltfläche in einer Nachricht auslösen möchten, konfigurieren Sie die
onClick
-Aktion der Schaltfläche, indem Sie ihreinteraction
aufOPEN_DIALOG
festlegen. - Auf einen Klick auf eine Schaltfläche auf der Startseite der Google Chat App reagieren Informationen zum Öffnen von Dialogfeldern über Startseiten finden Sie unter Startseite für die Google Chat App erstellen.
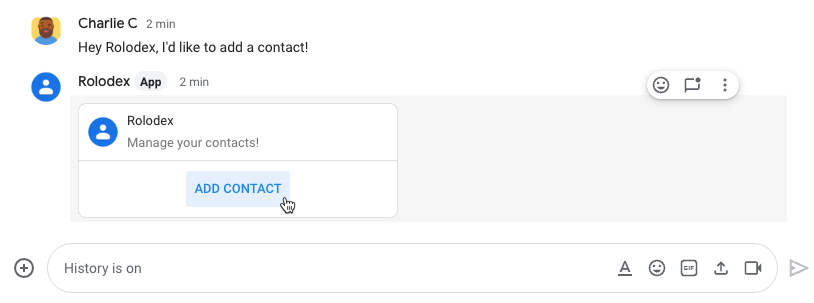
/addContact
zu verwenden. Die Meldung enthält auch eine Schaltfläche, auf die Nutzer klicken können, um den Befehl auszulösen.
Im folgenden Codebeispiel wird gezeigt, wie eine Dialoganfrage über eine Schaltfläche in einer Kartennachricht ausgelöst wird. Um das Dialogfeld zu öffnen, muss das Feld button.interaction
auf OPEN_DIALOG
gesetzt sein:
Node.js
Python
Java
Apps Script
In diesem Beispiel wird eine Kartennachricht gesendet, indem Karten-JSON zurückgegeben wird. Sie können auch den Apps Script-Kartendienst verwenden.
Erstes Dialogfeld öffnen
Wenn ein Nutzer eine Dialoganfrage auslöst, empfängt Ihre Chat-App ein Interaktionsereignis, das in der Chat API als Typ event
dargestellt wird. Wenn die Interaktion eine Dialoganfrage auslöst, wird das Feld dialogEventType
des Ereignisses auf REQUEST_DIALOG
gesetzt.
Zum Öffnen eines Dialogfelds kann die Chat-App auf die Anfrage ein actionResponse
-Objekt zurückgeben, bei dem type
auf DIALOG
und das Objekt Message
gesetzt ist. Um den Inhalt des Dialogfelds anzugeben, fügen Sie die folgenden Objekte ein:
- Ein
actionResponse
-Objekt, dessentype
aufDIALOG
gesetzt ist. - Ein
dialogAction
-Objekt. Das Feldbody
enthält die UI-Elemente, die auf der Karte angezeigt werden sollen, einschließlich eines oder mehrerersections
-Widgets. Wenn Sie Informationen von Nutzern erfassen möchten, können Sie Formulareingabe-Widgets und ein Schaltflächen-Widget angeben. Weitere Informationen zum Entwerfen von Formularinputs finden Sie unter Informationen von Nutzern erheben und verarbeiten.
Das folgende Codebeispiel zeigt, wie eine Chat-App eine Antwort zurückgibt, die ein Dialogfeld öffnet:
Node.js
Python
Java
Apps Script
In diesem Beispiel wird eine Kartennachricht gesendet, indem Karten-JSON zurückgegeben wird. Sie können auch den Kartendienst von Apps Script verwenden.
Dialogfeld einreichen
Wenn Nutzer auf eine Schaltfläche klicken, über die ein Dialogfeld gesendet wird, erhält Ihre Chat-App ein Interaktionsereignis vom Typ CARD_CLICKED
, bei dem dialogEventType
SUBMIT_DIALOG
ist.
Ihre Chat-App muss das Interaktionsereignis auf eine der folgenden Arten verarbeiten:
- Ein weiteres Dialogfeld zurückgeben, um eine weitere Karte oder ein weiteres Formular auszufüllen.
- Schließen Sie das Dialogfeld, nachdem Sie die von den Nutzern eingereichten Daten überprüft haben, und senden Sie optional eine Bestätigungsnachricht.
Optional: Ein anderes Dialogfeld zurückgeben
Nachdem Nutzer den ersten Dialog gesendet haben, können Chat-Apps einen oder mehrere zusätzliche Dialoge zurückgeben, damit Nutzer Informationen vor dem Senden prüfen, mehrstufige Formulare ausfüllen oder Formularinhalte dynamisch ausfüllen können.
Zur Verarbeitung der von Nutzern eingegebenen Daten verwendet die Chat-App das event.common.formInputs
-Objekt. Weitere Informationen zum Abrufen von Werten aus Eingabe-Widgets finden Sie unter Informationen von Nutzern erheben und verarbeiten.
Wenn Sie alle Daten im Blick behalten möchten, die Nutzer im ersten Dialogfeld eingeben, müssen Sie der Schaltfläche, über die das nächste Dialogfeld geöffnet wird, Parameter hinzufügen. Weitere Informationen findest du unter Daten auf eine andere Karte übertragen.
In diesem Beispiel öffnet eine Chat-App ein erstes Dialogfeld, das zu einem zweiten Dialogfeld zur Bestätigung führt, bevor die Eingabe gesendet wird:
Node.js
Python
Java
Apps Script
In diesem Beispiel wird eine Kartennachricht gesendet, indem Karten-JSON zurückgegeben wird. Sie können auch den Apps Script-Kartendienst verwenden.
Dialogfeld schließen
Wenn Nutzer in einem Dialogfeld auf eine Schaltfläche klicken, führt Ihre Chat-App die zugehörige Aktion aus und stellt dem Ereignisobjekt die folgenden Informationen zur Verfügung:
eventType
istCARD_CLICKED
.dialogEventType
istSUBMIT_DIALOG
.
Die Chat-App sollte ein ActionResponse
-Objekt zurückgeben, dessen type
auf DIALOG
und dialogAction
festgelegt ist.
Optional: Benachrichtigung anzeigen
Wenn Sie das Dialogfeld schließen, können Sie auch eine Textbenachrichtigung anzeigen lassen.
Die Chat-App kann mit einer Erfolgs- oder Fehlerbenachrichtigung antworten. Dazu wird ein ActionResponse
zurückgegeben, für das actionStatus
festgelegt ist.
Im folgenden Beispiel wird geprüft, ob die Parameter gültig sind, und das Dialogfeld wird je nach Ergebnis mit einer Textbenachrichtigung geschlossen:
Node.js
Python
Java
Apps Script
In diesem Beispiel wird eine Kartennachricht gesendet, indem Karten-JSON zurückgegeben wird. Sie können auch den Kartendienst von Apps Script verwenden.
Weitere Informationen zum Übergeben von Parametern zwischen Dialogfeldern finden Sie unter Daten auf eine andere Karte übertragen.
Optional: Bestätigungsnachricht senden
Wenn Sie das Dialogfeld schließen, können Sie auch eine neue Nachricht senden oder eine vorhandene aktualisieren.
Wenn du eine neue Nachricht senden möchtest, gib ein ActionResponse
-Objekt zurück, bei dem type
auf NEW_MESSAGE
gesetzt ist. Im folgenden Beispiel wird der Dialog mit einer Textbenachrichtigung und einer Bestätigungs-SMS geschlossen:
Node.js
Python
Java
Apps Script
In diesem Beispiel wird eine Kartennachricht gesendet, indem Karten-JSON zurückgegeben wird. Sie können auch den Kartendienst von Apps Script verwenden.
Wenn du eine Nachricht aktualisieren möchtest, gib ein actionResponse
-Objekt zurück, das die aktualisierte Nachricht enthält und für das type
einer der folgenden Werte festgelegt ist:
UPDATE_MESSAGE
: Aktualisiert die Nachricht, die die Dialoganfrage ausgelöst hat.UPDATE_USER_MESSAGE_CARDS
: Aktualisiert die Karte anhand einer Linkvorschau.
Fehlerbehebung
Wenn eine Google Chat-App oder Karte einen Fehler zurückgibt, wird in der Chat-Benutzeroberfläche die Meldung „Ein Fehler ist aufgetreten“ angezeigt. oder „Ihre Anfrage konnte nicht verarbeitet werden“ Manchmal wird in der Chat-Benutzeroberfläche keine Fehlermeldung angezeigt, aber die Chat-App oder -Karte führt zu einem unerwarteten Ergebnis. Beispielsweise wird eine Kartennachricht möglicherweise nicht angezeigt.
Auch wenn in der Chat-Benutzeroberfläche keine Fehlermeldung angezeigt wird, sind beschreibende Fehlermeldungen und Protokolldaten verfügbar, die Ihnen bei der Fehlerbehebung helfen, wenn die Fehlerprotokollierung für Chat-Apps aktiviert ist. Informationen zum Ansehen, Entfernen und Beheben von Fehlern finden Sie unter Google Chat-Fehler beheben.
Weitere Informationen
- Sehen Sie sich das Beispiel „Contact Manager“ an, eine Chat-App, in der über Dialogfelder Kontaktdaten erfasst werden.
- Dialogfelder über die Startseite der Google Chat App öffnen
- Slash-Befehle einrichten und darauf reagieren
- Von Nutzern eingegebene Informationen verarbeiten