本頁說明如何建立首頁,以便與 Google Chat 應用程式。在應用程式的首頁中稱為「應用程式首頁」 Google Chat API 是「首頁」分頁中的可自訂資訊卡介面 / 即時訊息聊天室 使用者與 Chat 擴充應用程式之間的連線

您可以使用應用程式首頁,分享與 Chat 應用程式互動或讓使用者透過 Chat 存取及使用外部服務或工具的訣竅。
使用 Card Builder 設計及預覽即時通訊應用程式的訊息和使用者介面:
開啟資訊卡建立工具必要條件
Node.js
已啟用互動功能的 Google Chat 應用程式。如要使用 HTTP 服務建立互動式 Chat 應用程式,請完成這個快速入門導覽課程。
Python
已啟用互動功能的 Google Chat 應用程式。如要建立 請使用 HTTP 服務互動式即時通訊應用程式,請完成快速入門導覽課程。
Java
已啟用互動功能的 Google Chat 應用程式。如要使用 HTTP 服務建立互動式 Chat 應用程式,請完成這個快速入門導覽課程。
Apps Script
已啟用互動功能的 Google Chat 應用程式。如要在 Apps Script 中建立互動式 Chat 應用程式,請完成這個快速入門。
設定 Chat 應用程式首頁
如要支援應用程式首頁,您必須設定 Chat 應用程式,以便接收 APP_HOME
互動事件。每當使用者透過 Chat 應用程式傳送即時訊息,點選「首頁」分頁時,Chat 應用程式就會收到這項事件。
如要在 Google Cloud 控制台中更新配置設定,請按照下列步驟操作:
前往 Google Cloud 控制台中的「選單」。 >「更多產品」 > Google Workspace > 產品庫 > Google Chat API。
依序按一下「管理」和「設定」分頁標籤。
在「互動功能」下方,前往「功能」專區設定應用程式首頁:
- 勾選「接收 1:1 訊息」核取方塊。
- 勾選「支援應用程式首頁」核取方塊。
如果 Chat 應用程式使用 HTTP 服務,請前往「連線設定」,然後在「應用程式首頁網址」欄位中指定端點。您可以使用在「HTTP 端點網址」欄位中指定的網址。
按一下 [儲存]。
建構應用程式主畫面資訊卡
當使用者開啟應用程式主畫面時,Chat 應用程式必須透過傳回 pushCard
導覽和 Card
的 RenderActions
例項,處理 APP_HOME
互動事件。如要建立
就可以加入互動式小工具,例如按鈕
Chat 應用程式可處理的輸入內容或文字輸入內容
以其他資訊卡或對話方塊做出回應。
在下列範例中,Chat 應用程式會顯示初始應用程式首頁資訊卡,其中顯示資訊卡建立時間和按鈕。使用者點選按鈕後,Chat 應用程式 會傳回更新過的資訊卡,以顯示更新卡片的時間。
Node.js
Python
Java
Apps Script
實作在所有 APP_HOME
之後呼叫的 onAppHome
函式
互動事件:
這個範例會傳回 卡片 JSON,藉此傳送資訊卡訊息。你也可以使用 Apps Script 資訊卡服務。
回應應用程式主畫面互動
如果您的初始應用程式首頁資訊卡包含互動式小工具 (例如按鈕或選取輸入內容),Chat 應用程式必須透過 updateCard
導覽,傳回 RenderActions
的例項,以處理相關互動事件。進一步瞭解如何處理互動式廣告
小工具,請參閱
處理使用者輸入的資訊。
在上例中,初始的應用程式首頁資訊卡包含按鈕。只要
使用者點選按鈕、CARD_CLICKED
互動事件
會觸發 updateAppHome
函式來重新整理應用程式首頁資訊卡,如所示
下列程式碼:
開啟對話方塊
Chat 應用程式也可以開啟對話框,回應應用程式首頁中的互動內容。
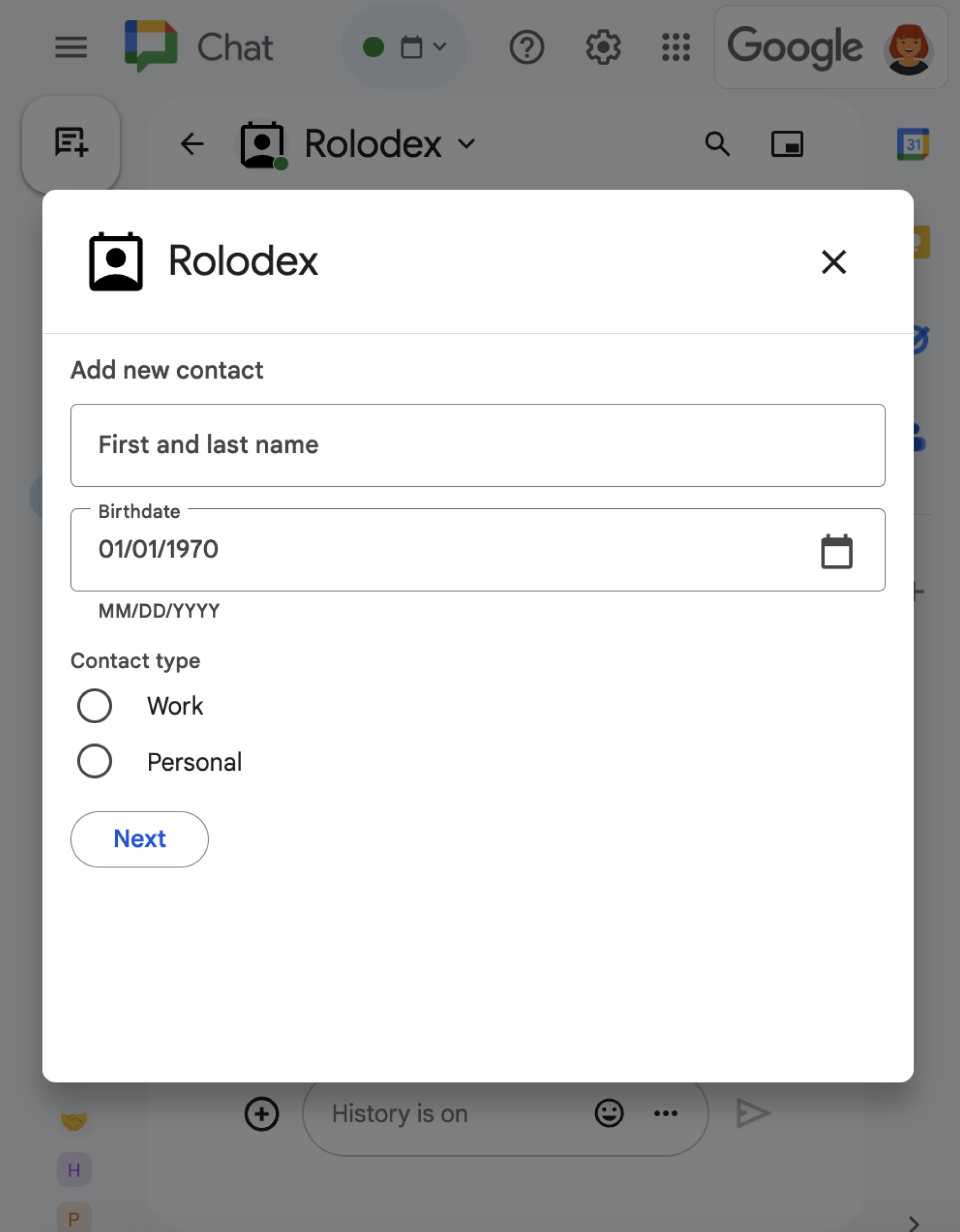
如要從應用程式首頁開啟對話方塊,請依下列方式處理相關互動事件:
使用包含 Card
的 updateCard
導覽傳回 renderActions
物件。在以下範例中,Chat 應用程式會處理 CARD_CLICKED
互動事件並開啟對話方塊,以回應應用程式首頁資訊卡的按鈕點選動作:
{ renderActions: { action: { navigations: [{ updateCard: { sections: [{
header: "Add new contact",
widgets: [{ "textInput": {
label: "Name",
type: "SINGLE_LINE",
name: "contactName"
}}, { textInput: {
label: "Address",
type: "MULTIPLE_LINE",
name: "address"
}}, { decoratedText: {
text: "Add to favorites",
switchControl: {
controlType: "SWITCH",
name: "saveFavorite"
}
}}, { decoratedText: {
text: "Merge with existing contacts",
switchControl: {
controlType: "SWITCH",
name: "mergeContact",
selected: true
}
}}, { buttonList: { buttons: [{
text: "Next",
onClick: { action: { function: "openSequentialDialog" }}
}]}}]
}]}}]}}}
如要關閉對話方塊,請處理下列互動事件:
CLOSE_DIALOG
:關閉對話方塊並返回 Chat 應用程式的初始應用程式首頁資訊卡。CLOSE_DIALOG_AND_EXECUTE
:關閉對話方塊並重新整理應用程式首頁 資訊卡
以下程式碼範例會使用 CLOSE_DIALOG
關閉對話方塊,並返回應用程式主畫面資訊卡:
{ renderActions: { action: {
navigations: [{ endNavigation: { action: "CLOSE_DIALOG" }}]
}}}
如要向使用者收集資訊,您也可以建構連續對話方塊。如要瞭解如何建構連續對話方塊,請參閱「開啟對話方塊並回應」。