Na tej stronie dowiesz się, jak Twoja aplikacja Google Chat może otwierać okna odpowiadania użytkownikom.
Okna to oparte na kartach okna interfejsy, które otwierają się z poziomu pokoju czatu lub wiadomości. Okno i jego zawartość są widoczne tylko dla użytkownika, który je otworzy.
Aplikacje do obsługi czatu mogą używać okien, aby prosić użytkowników Google Chat o informacje, w tym formularze wieloetapowe, i zbierać od nich informacje. Więcej informacji o przesyłaniu danych wejściowych do formularza znajdziesz w artykule Zbieranie i przetwarzanie informacji od użytkowników.
Wymagania wstępne
Node.js
Aplikacja Google Chat, w której można korzystać z funkcji interaktywnych. Aby utworzyć interaktywną aplikację Google Chat przy użyciu usługi HTTP, wykonaj to krótkie wprowadzenie.
Python
Aplikacja Google Chat, w której włączono funkcje interaktywne. Aby utworzyć interaktywną aplikację Google Chat przy użyciu usługi HTTP, wykonaj to krótkie wprowadzenie.
Java
Aplikacja Google Chat, w której włączono funkcje interaktywne. Aby utworzyć interaktywną aplikację do obsługi czatu przy użyciu usługi HTTP, wykonaj to krótkie wprowadzenie.
Google Apps Script
Aplikacja Google Chat, w której można korzystać z funkcji interaktywnych. Aby utworzyć interaktywną aplikację do obsługi czatu w Apps Script, zapoznaj się z tym krótkim wprowadzeniem.
Otwórz okno
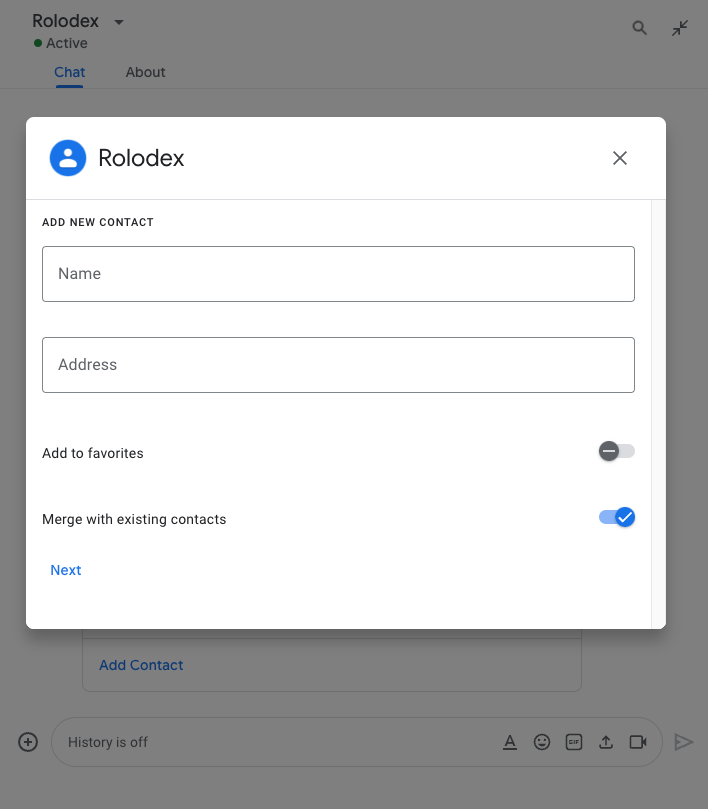
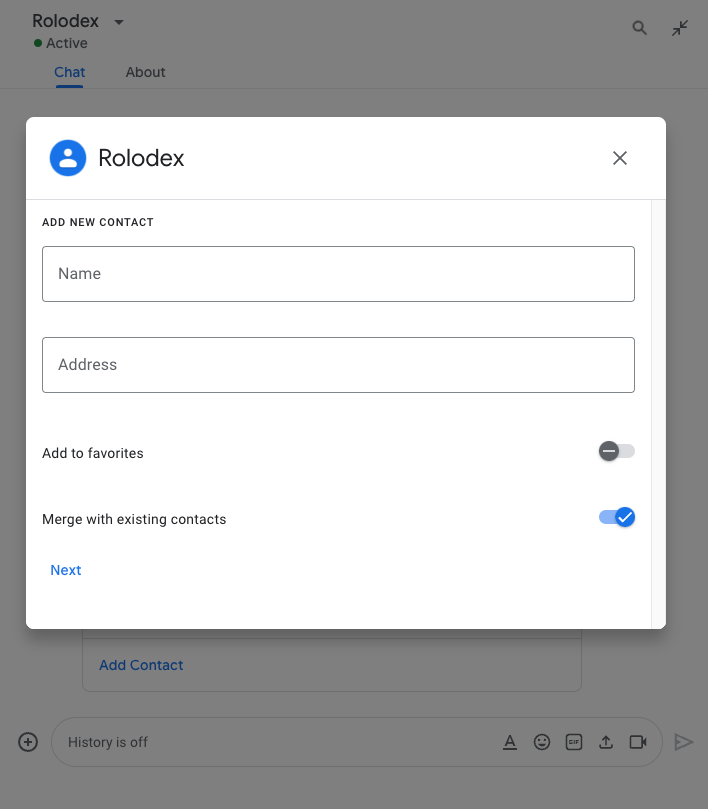
W tej sekcji dowiesz się, jak odpowiadać i konfigurować dialogy:
- Wywołanie żądania dialogu w reakcji na interakcję użytkownika.
- Zaakceptuj prośbę, wracając do okna i otwierając okno dialogowe.
- Gdy użytkownicy prześlą informacje, przetwórz przesłane dane, zamykając okno lub wyświetlając inne okno.
Wywołanie prośby o wyświetlenie okna
Aplikacja Google Chat może otwierać okna dialogowe tylko w odpowiedzi na interakcję użytkownika, taką jak polecenie po ukośniku lub kliknięcie przycisku w wiadomości na karcie.
Aby odpowiedzieć użytkownikom za pomocą dialogu, aplikacja do czatu musi stworzyć interakcję, która uruchamia prośbę o dialog, np.:
- Odpowiadanie na polecenie po ukośniku Aby aktywować żądanie z polecenia po ukośniku, podczas konfigurowania polecenia zaznacz pole wyboru Otwiera okno.
- Reakcja na kliknięcie przycisku w wiadomości,
czy to w ramach karty, czy na dole wiadomości. Aby wywołać prośbę za pomocą przycisku w wiadomości, skonfiguruj działanie
onClick
przycisku, ustawiając wartość parametruinteraction
naOPEN_DIALOG
. - Odpowiadanie na kliknięcie przycisku na stronie głównej aplikacji Google Chat Informacje o otwieraniu dialogów z poziomu strony głównej znajdziesz w artykule Tworzenie strony głównej aplikacji Google Chat.
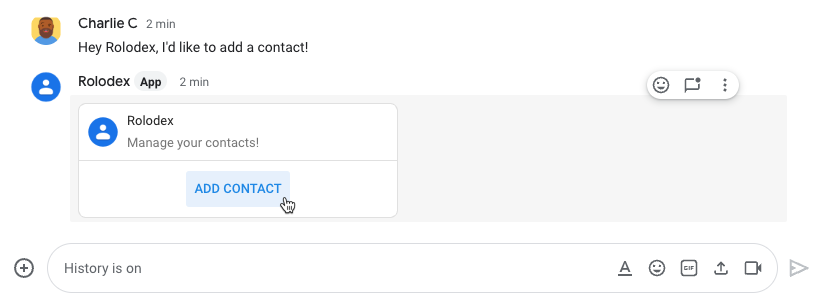
/addContact
. Wiadomość zawiera również przycisk, który użytkownicy mogą kliknąć, aby wywołać polecenie.
Poniższy przykładowy kod pokazuje, jak wywołać żądanie okna za pomocą przycisku w komunikacie karty. Aby można było otworzyć to okno, pole button.interaction
jest ustawione na OPEN_DIALOG
:
Node.js
Python
Java
Google Apps Script
W tym przykładzie wysłanie wiadomości dotyczącej karty wymaga zwrócenia pliku JSON karty. Możesz też użyć usługi kart Apps Script.
Otwórz pierwsze okno
Gdy użytkownik wywoła żądanie w oknie, Twoja aplikacja do obsługi czatu otrzyma zdarzenie interakcji reprezentowane w interfejsie Chat API jako typ event
. Jeśli interakcja powoduje wysłanie żądania dialogu, pole dialogEventType
zdarzenia ma wartość REQUEST_DIALOG
.
Aby otworzyć okno, aplikacja Google Chat może odpowiedzieć na to żądanie, zwracając obiekt actionResponse
z obiektem type
ustawionym na DIALOG
i Message
. Aby określić zawartość okna, użyj tych obiektów:
- Obiekt
actionResponse
z wartościątype
ustawioną naDIALOG
. - Obiekt
dialogAction
. Polebody
zawiera elementy interfejsu użytkownika, które mają być wyświetlane na karcie, w tym co najmniej 1 widżetsections
. Aby zbierać informacje od użytkowników, możesz użyć widżetów formularza i widżetu przycisku. Więcej informacji o projektowaniu pól formularza znajdziesz w artykule Zbieranie i przetwarzanie informacji od użytkowników.
Ten przykładowy kod pokazuje, jak aplikacja do obsługi czatu zwraca odpowiedź otwierającą okno:
Node.js
Python
Java
Google Apps Script
W tym przykładzie wysyłamy wiadomość z karty, zwracając obiekt JSON karty. Możesz też użyć usługi kart Apps Script.
Obsługa przesyłania okna
Gdy użytkownicy klikają przycisk, który przesyła okno dialogowe, Twoja aplikacja do czatu otrzymuje zdarzenie interakcji CARD_CLICKED
, w którym parametr dialogEventType
ma wartość SUBMIT_DIALOG
.
Aplikacja Google Chat musi obsłużyć zdarzenie interakcji, wykonując jedną z tych czynności:
- Zwróć kolejne okno, aby wypełnić inną kartę lub formularz.
- Zamknij okno po zweryfikowaniu danych przesłanych przez użytkownika i opcjonalnie wyślij wiadomość z potwierdzeniem.
Opcjonalnie: zwróć kolejne okno
Gdy użytkownicy prześlą pierwsze okno, aplikacje Google Chat mogą zwrócić jedno lub kilka dodatkowych okien, aby ułatwić użytkownikom przeglądanie informacji przed przesłaniem, wypełnienie wieloetapowych formularzy lub dynamiczne wypełnianie treści formularza.
Aby przetwarzać dane wprowadzane przez użytkowników, aplikacja Chat używa obiektu event.common.formInputs
. Więcej informacji o pobieraniu wartości z widżetów z danymi wejściowymi znajdziesz w artykule Zbieranie i przetwarzanie informacji o użytkownikach.
Aby śledzić dane wpisywane przez użytkowników w początkowym oknie, musisz dodać parametry do przycisku otwierającego następne okno. Szczegółowe informacje znajdziesz w artykule Przenoszenie danych na inną kartę.
W tym przykładzie aplikacja Google Chat otwiera pierwsze okno, które prowadzi do drugiego okna z prośbą o potwierdzenie przed przesłaniem:
Node.js
Python
Java
Google Apps Script
W tym przykładzie wysłanie wiadomości dotyczącej karty wymaga zwrócenia pliku JSON karty. Możesz też użyć usługi karty w Apps Script.
Zamknij okno
Gdy użytkownik kliknie przycisk w oknie, aplikacja Google Chat wykona powiązane działanie i dostarczy obiektowi zdarzenia te informacje:
eventType
toCARD_CLICKED
.dialogEventType
toSUBMIT_DIALOG
.
Aplikacja do obsługi czatu powinna zwrócić obiekt ActionResponse
z type
ustawionym na DIALOG
i dialogAction
.
Opcjonalnie: wyświetl powiadomienie
Po zamknięciu okna możesz też wyświetlić powiadomienie tekstowe.
Aplikacja Chat może odpowiedzieć pomyślnie lub wyświetlić powiadomienie o błędzie, zwracając obiekt ActionResponse
z ustawioną wartością actionStatus
.
W tym przykładzie sprawdzamy, czy parametry są prawidłowe, i zamykamy okno z powiadomieniem tekstowym w zależności od wyniku:
Node.js
Python
Java
Google Apps Script
W tym przykładzie wysyłamy wiadomość z karty, zwracając obiekt JSON karty. Możesz też użyć usługi kart Apps Script.
Szczegółowe informacje o przekazywaniu parametrów między dialogami znajdziesz w artykule Przenoszenie danych na inną kartę.
Opcjonalnie: wyślij wiadomość z potwierdzeniem
Po zamknięciu okna możesz też wysłać nową wiadomość lub zaktualizować istniejącą.
Aby wysłać nową wiadomość, zwróć obiekt ActionResponse
z wartością type
ustawioną na NEW_MESSAGE
. W tym przykładzie dialog kończy się powiadomieniem tekstowym i tekstową wiadomością potwierdzającą:
Node.js
Python
Java
Google Apps Script
W tym przykładzie wysyłamy wiadomość z karty, zwracając obiekt JSON karty. Możesz też użyć usługi kart Apps Script.
Aby zaktualizować wiadomość, zwróć obiekt actionResponse
zawierający zaktualizowaną wiadomość i ustaw wartość type
na jedną z tych opcji:
UPDATE_MESSAGE
: aktualizuje komunikat, który wywołał żądanie okna.UPDATE_USER_MESSAGE_CARDS
: aktualizuje kartę z podglądu linku.
Rozwiązywanie problemów
Gdy aplikacja Google Chat lub karta zwraca błąd, interfejs Google Chat wyświetla komunikat „Coś poszło nie tak”. lub „Nie udało się przetworzyć Twojej prośby”. Czasami w UI Google Chat nie wyświetla się żaden komunikat o błędzie, ale aplikacja lub karta Google Chat zwraca nieoczekiwany wynik, na przykład wiadomość na karcie.
Mimo że komunikat o błędzie może nie być wyświetlany w interfejsie Google Chat, są dostępne opisowe komunikaty o błędach i dane logów, które pomogą Ci w naprawianiu błędów, gdy logowanie błędów w aplikacjach Google Chat jest włączone. Informacje o wyświetlaniu, debugowaniu i naprawianiu błędów znajdziesz w artykule Rozwiązywanie problemów z błędami w Google Chat.
Powiązane artykuły
- Zobacz przykładową aplikację Contact Manager, która jest aplikacją do obsługi czatu, która używa okien do zbierania informacji kontaktowych.
- Otwieranie dialogów na stronie głównej aplikacji Google Chat
- Konfigurowanie poleceń po ukośniku i reagowanie na nie
- Informacje o procesie podawane przez użytkowników