The OAuth-based Google Sign-in "Streamlined" linking type adds Google Sign-In on top of OAuth-based account linking. This provides seamless voice-based linking for Google users while also enabling account linking for users who registered to your service with a non-Google identity.
This linking type begins with Google Sign-In, which allows you to check if the user's Google profile information exists in your system. If the user's information isn't found in your system, a standard OAuth flow begins. The user can also choose to create a new account with their Google profile information.
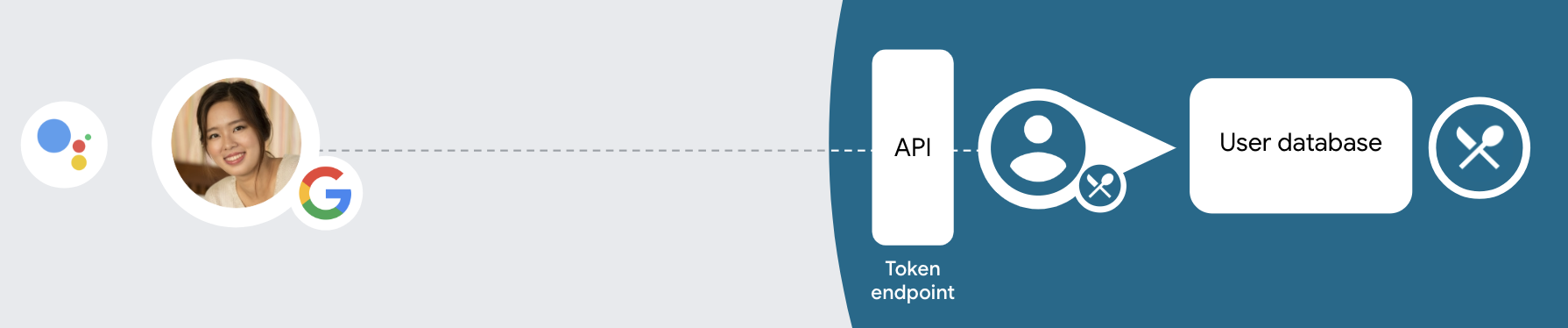
To perform account linking with the Streamlined linking type, follow these general steps:
- First, ask the user to give consent to access their Google profile.
- Use the information in their profile to identify the user.
- If you can't find a match for the Google user in your authentication system,
the flow proceeds depending on whether you configured your Actions project
in the Actions console to allow user account creation via voice or only on
your website.
- If you allow account creation via voice, validate the ID token received from Google. You can then create a user based on the profile information contained in the ID token.
- If you don't allow account creation via voice, the user is transferred to a browser where they can load your authorization page and complete the user creation flow.
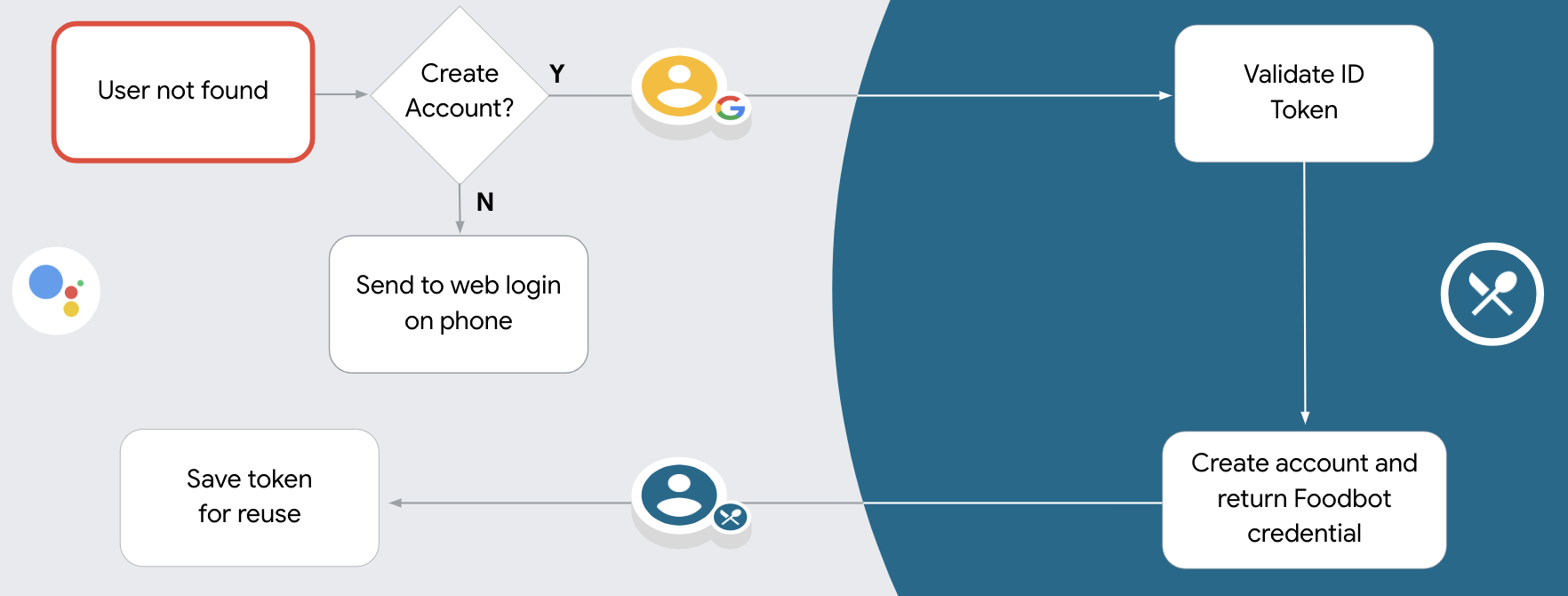
Support account creation via voice
If you allow user account creation via voice, Assistant asks the user whether they want to do the following:
- Create a new account on your system using their Google account information, or
- Sign in to your authentication system with a different account if they have an existing non-Google account.
Allowing account creation via voice is recommended if you want to minimize the friction of the account creation flow. The user only needs to leave the voice flow if they want to sign in using an existing non-Google account.
Disallow account creation via voice
If you disallowed user account creation via voice, Assistant opens the URL to the web site that you provided for user authentication. If the interaction is happening on a device that doesn't have a screen, Assistant directs the user to a phone to continue the account linking flow.
Disallowing creation is recommended if:
You do not want to allow users that have non-Google accounts to create a new user account and want them to link to their existing user accounts in your authentication system instead. For example, if you offer a loyalty program, you might want to make sure that the user doesn't lose the points accrued on their existing account.
You need to have full control of the account creation flow. For example, you could disallow creation if you need to show your terms of service to the user during account creation.
Implement OAuth-based Google Sign-in "Streamlined" linking
Accounts are linked with industry standard OAuth 2.0 flows. Actions on Google supports the implicit and authorization code flows.
In the implicit code flow, Google opens your authorization endpoint in the user's browser. After successful sign in, you return a long-lived access token to Google. This access token is now included in every request sent from the Assistant to your Action.
In the authorization code flow, you need two endpoints:
- The authorization endpoint, which is responsible for presenting the sign-in UI to your users that aren't already signed in and recording consent to the requested access in the form of a short-lived authorization code.
- The token exchange endpoint, which is responsible for two types of exchanges:
- Exchanges an authorization code for a long-lived refresh token and a short-lived access token. This exchange happens when the user goes through the account linking flow.
- Exchanges a long-lived refresh token for a short-lived access token. This exchange happens when Google needs a new access token because the one it had expired.
Although the implicit code flow is simpler to implement, Google recommends that access tokens issued using the implicit flow never expire, because using token expiration with the implicit flow forces the user to link their account again. If you need token expiration for security reasons, you should strongly consider using the auth code flow instead.
Configure the project
To configure your project to use Streamlined linking, follow these steps:
- Open the Actions console and select the project you want to use.
- Click on the Develop tab and choose Account linking.
- Enable the switch next to Account linking.
- In the Account creation section, select Yes.
In Linking type, select OAuth & Google Sign In and Implicit.
In Client Information, do the following:
- Assign a value to Client ID issued by your Actions to Google to identify requests coming from Google.
- Insert the URLs for your Authorization and Token Exchange endpoints.
Click Save.
Implement your OAuth server
To support the OAuth 2.0 implicit flow, your service makes an authorization endpoint available by HTTPS. This endpoint is responsible for authenticating and obtaining consent from users for data access. The authorization endpoint presents a sign-in UI to your users that aren't already signed in and records consent to the requested access.
When your Action needs to call one of your service's authorized APIs, Google uses this endpoint to get permission from your users to call these APIs on their behalf.
A typical OAuth 2.0 implicit flow session initiated by Google has the following flow:
- Google opens your authorization endpoint in the user's browser. The user signs in if not signed in already, and grants Google permission to access their data with your API if they haven't already granted permission.
- Your service creates an access token and returns it to Google by redirecting the user's browser back to Google with the access token attached to the request.
- Google calls your service's APIs, and attaches the access token with each request. Your service verifies that the access token grants Google authorization to access the API and then completes the API call.
Handle authorization requests
When your Action needs to perform account linking via an OAuth 2.0 implicit flow, Google sends the user to your authorization endpoint with a request that includes the following parameters:
Authorization endpoint parameters | |
---|---|
client_id |
The client ID you assigned to Google. |
redirect_uri |
The URL to which you send the response to this request. |
state |
A bookkeeping value that is passed back to Google unchanged in the redirect URI. |
response_type |
The type of value to return in the response. For the OAuth 2.0 implicit
flow, the response type is always token . |
For example, if your authorization endpoint is available at https://myservice.example.com/auth
,
a request might look like:
GET https://myservice.example.com/auth?client_id=GOOGLE_CLIENT_ID&redirect_uri=REDIRECT_URI&state=STATE_STRING&response_type=token
For your authorization endpoint to handle sign-in requests, do the following steps:
Verify the
client_id
andredirect_uri
values to prevent granting access to unintended or misconfigured client apps:- Confirm that the
client_id
matches the client ID you assigned to Google. - Confirm that the URL specified by the
redirect_uri
parameter has the following form:https://oauth-redirect.googleusercontent.com/r/YOUR_PROJECT_ID
YOUR_PROJECT_ID is the ID found on the Project settings page of the Actions Console.
- Confirm that the
Check if the user is signed in to your service. If the user isn't signed in, complete your service's sign-in or sign-up flow.
Generate an access token that Google will use to access your API. The access token can be any string value, but it must uniquely represent the user and the client the token is for and must not be guessable.
Send an HTTP response that redirects the user's browser to the URL specified by the
redirect_uri
parameter. Include all of the following parameters in the URL fragment:access_token
: the access token you just generatedtoken_type
: the stringbearer
state
: the unmodified state value from the original request The following is an example of the resulting URL:https://oauth-redirect.googleusercontent.com/r/YOUR_PROJECT_ID#access_token=ACCESS_TOKEN&token_type=bearer&state=STATE_STRING
Google's OAuth 2.0 redirect handler will receive the access token and confirm
that the state
value hasn't changed. After Google has obtained an
access token for your service, Google will attach the token to subsequent calls
to your Action as part of the AppRequest.
Handle automatic linking
After the user gives your Action consent to access their Google profile, Google sends a request that contains a signed assertion of the Google user's identity. The assertion contains information that includes the user's Google Account ID, name, and email address. The token exchange endpoint configured for your project handles that request.
If the corresponding Google account is already present in your authentication system,
your token exchange endpoint returns a token for the user. If the Google account doesn't
match an existing user, your token exchange endpoint returns a user_not_found
error.
The request has the following form:
POST /token HTTP/1.1 Host: oauth2.example.com Content-Type: application/x-www-form-urlencoded grant_type=urn:ietf:params:oauth:grant-type:jwt-bearer&intent=get&assertion=JWT&consent_code=CONSENT_CODE&scope=SCOPES
Your token exchange endpoint must be able to handle the following parameters:
Token endpoint parameters | |
---|---|
grant_type |
The type of token being exchanged. For these requests, this
parameter has the value urn:ietf:params:oauth:grant-type:jwt-bearer . |
intent |
For these requests, the value of this parameter is `get`. |
assertion |
A JSON Web Token (JWT) that provides a signed assertion of the Google user's identity. The JWT contains information that includes the user's Google Account ID, name, and email address. |
consent_code |
Optional: When present, a one-time code that indicates that the user has granted consent for your Action to access the specified scopes. |
scope |
Optional: Any scopes you configured Google to request from users. |
When your token exchange endpoint receives the linking request, it should do the following:
Validate and decode the JWT assertion
You can validate and decode the JWT assertion by using a JWT-decoding library for your language. Use Google's public keys (available in JWK or PEM format) to verify the token's signature.
When decoded, the JWT assertion looks like the following example:
{ "sub": 1234567890, // The unique ID of the user's Google Account "iss": "https://accounts.google.com", // The assertion's issuer "aud": "123-abc.apps.googleusercontent.com", // Your server's client ID "iat": 233366400, // Unix timestamp of the assertion's creation time "exp": 233370000, // Unix timestamp of the assertion's expiration time "name": "Jan Jansen", "given_name": "Jan", "family_name": "Jansen", "email": "jan@gmail.com", // If present, the user's email address "locale": "en_US" }
In addition to verifying the token's signature, verify that the assertion's issuer
(iss
field) is https://accounts.google.com
and that the audience (aud
field)
is the client ID assigned to your Action.
Check if the Google account is already present in your authentication system
Check whether either of the following conditions are true:
- The Google Account ID, found in the assertion's
sub
field, is in your user database. - The email address in the assertion matches a user in your user database.
If either condition is true, the user has already signed up and you can issue an access token.
If neither the Google Account ID nor the email address specified in the assertion
matches a user in your database, the user hasn't signed up yet. In this case, your
token exchange endpoint should reply with a HTTP 401 error, that specifies error=user_not_found
,
as in the following example:
HTTP/1.1 401 Unauthorized Content-Type: application/json;charset=UTF-8 { "error":"user_not_found", }When Google receives the 401 error response with a
user_not_found
error, Google
calls your token exchange endpoint with the value of the intent
parameter
set to create and sending an ID token that contains the user's profile information
with the request.
Handle account creation via Google Sign-In
When a user needs to create an account on your service, Google makes a
request to your token exchange endpoint that specifies
intent=create
, as in the following example:
POST /token HTTP/1.1 Host: oauth2.example.com Content-Type: application/x-www-form-urlencoded response_type=token&grant_type=urn:ietf:params:oauth:grant-type:jwt-bearer&scope=SCOPES&intent=create&consent_code=CONSENT_CODE&assertion=JWT[&NEW_ACCOUNT_INFO]
The assertion
parameter contains A JSON Web Token (JWT) that provides
a signed assertion of the Google user's identity. The JWT contains information
that includes the user's Google Account ID, name, and email address, which you can use
to create a new account on your service.
To respond to account creation requests, your token exchange endpoint must do the following:
Validate and decode the JWT assertion
You can validate and decode the JWT assertion by using a JWT-decoding library for your language. Use Google's public keys (available in JWK or PEM format) to verify the token's signature.
When decoded, the JWT assertion looks like the following example:
{ "sub": 1234567890, // The unique ID of the user's Google Account "iss": "https://accounts.google.com", // The assertion's issuer "aud": "123-abc.apps.googleusercontent.com", // Your server's client ID "iat": 233366400, // Unix timestamp of the assertion's creation time "exp": 233370000, // Unix timestamp of the assertion's expiration time "name": "Jan Jansen", "given_name": "Jan", "family_name": "Jansen", "email": "jan@gmail.com", // If present, the user's email address "locale": "en_US" }
In addition to verifying the token's signature, verify that the assertion's issuer
(iss
field) is https://accounts.google.com
and that the audience (aud
field)
is the client ID assigned to your Action.
Validate user information and create new account
Check whether either of the following conditions are true:
- The Google Account ID, found in the assertion's
sub
field, is in your user database. - The email address in the assertion matches a user in your user database.
If either condition is true, prompt the user to link their existing account with
their Google Account by responding to the request with an HTTP 401 error, specifying
error=linking_error
and the user's email address as the login_hint
, as in the
following example:
HTTP/1.1 401 Unauthorized Content-Type: application/json;charset=UTF-8 { "error":"linking_error", "login_hint":"foo@bar.com" }
If neither condition is true, create a new user account using the information provided in the JWT. New accounts do not typically have a password set. It is recommended that you add Google Sign In to other platforms to enable users to log in via Google across the surfaces of your application. Alternatively, you can email the user a link that starts your password recovery flow to allow the user to set a password for signing in on other platforms.
When the creation is completed, issue an access token and return the values in a JSON object in the body of your HTTPS response, like in the following example:
{ "token_type": "Bearer", "access_token": "ACCESS_TOKEN", "expires_in": SECONDS_TO_EXPIRATION }
Design the voice user interface for the authentication flow
Check if the user is verified and start the account linking flow
- Open your Actions Builder project in the Actions Console.
- Create a new scene to start account linking in your Action:
- Click Scenes.
- Click the add (+) icon to add a new scene.
- In the newly created scene, click the add add icon for Conditions.
- Add a condition that checks if the user associated with the conversation is a
a verified user. If the check fails, your Action can't perform account linking
during the conversation, and should fall back to providing access to
functionality that doesn't require account linking.
- In the
Enter new expression
field under Condition, enter the following logic:user.verificationStatus != "VERIFIED"
- Under Transition, select a scene that doesn't require account linking or a scene that is the entry point to guest-only functionality.
- In the
- Click the add add icon for Conditions.
- Add a condition to trigger an account linking flow if the user doesn't have
an associated identity.
- In the
Enter new expression
field under Condition, enter the following logic::user.verificationStatus == "VERIFIED"
- Under Transition, select the Account Linking system scene.
- Click Save.
- In the
After saving, a new account linking system scene called <SceneName>_AccountLinking
is added to your project.
Customize the account linking scene
- Under Scenes, select the account linking system scene.
- Click Send prompt and add a short sentence to describe to the user why the Action needs to access their identity (for example "To save your preferences").
- Click Save.
- Under Conditions, click If user successfully completes account linking.
- Configure how the flow should proceed if the user agrees to link their account. For example, call the webhook to process any custom business logic required and transition back to the originating scene.
- Click Save.
- Under Conditions, click If user cancels or dismisses account linking.
- Configure how the flow should proceed if the user doesn't agree to link their account. For example, send an acknowledging message and redirect to scenes that provide functionality that doesn't require account linking.
- Click Save.
- Under Conditions, click If system or network error occurs.
- Configure how the flow should proceed if the account linking flow can't be completed because of system or network errors. For example, send an acknowledging message and redirect to scenes that provide functionality that doesn't require account linking.
- Click Save.
Handle data access requests
If the Assistant request contains an access token, check first that the access token is valid and not expired and then retrieve from your user account database the user account associated with the token.