Read It is a Google Assistant feature available on Android devices that offers another way for users to read long-form web content like news articles and blog posts. Users can say something like "Hey Google, read it" to have an app read web-based content out loud, highlight the words being read, and auto-scroll the page. To learn more about this feature, you can also read the Read It product update post.
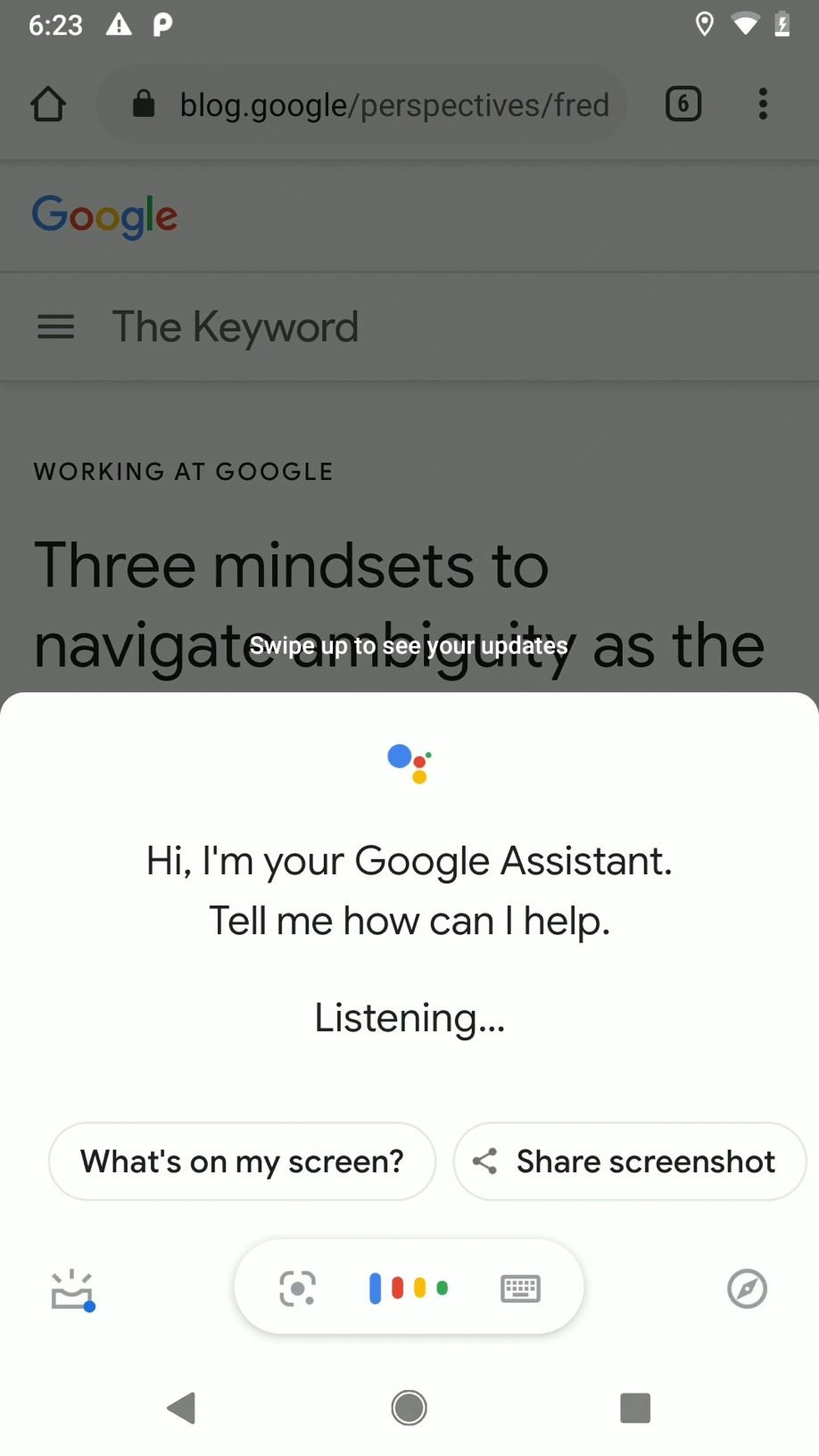
Android apps with web-based content can support Read It by providing information
to Assistant using the onProvideAssistContent()
method.
This process helps maintain the structure of data as it's shared with Assistant. Users who receive shared app content can then be deep linked or receive content directly, instead of as text or a screenshot.
Implement onProvideAssistContent()
for any web-based content
and any sharable entity
in your app.
Provide content to Assistant
For Read It to access your content, your app must provide Assistant with information about the content, like its web URI and some basic context. Assistant can then retrieve your content to be read out loud to the user.
For Android apps that already implement web-based content using WebViews or Chrome Custom Tabs, use the same web URIs for Read It as a starting point.
When combining Read It functionality with built-in intents, you only need to
implement onProvideAssistContent()
for the final app activity in the user's
task flow after invoking the App Action.
For example, if your app displays
news articles, implement onProvideAssistContent()
in the final screen
showing the article; you don't need to implement it for any in-progress or
preview screens.
Provide a web URI for your content in the uri
field of AssistContent
.
Provide contextual information as a JSON-LD object
using schema.org vocabulary in the
structuredData
field.
The following code snippet shows an example of providing content to Assistant:
Kotlin
override fun onProvideAssistContent(outContent: AssistContent) { super.onProvideAssistContent(outContent) // Set the web URI for content to be read from a // WebView, Chrome Custom Tab, or other source val urlString = url.toString() outContent.setWebUri(Uri.parse(urlString)) // Create JSON-LD object based on schema.org structured data val structuredData = JSONObject() .put("@type", "Article") .put("name", "ExampleName of blog post") .put("url", outContent.getWebUri()) .toString() outContent.setStructuredData(structuredData) }
Java
@Override public void onProvideAssistContent(AssistContent outContent) { // Set the web URI for content to be read from a // WebView, Chrome Custom Tab, or other source String urlString = url.toString(); outContent.setWebUri(Uri.parse(urlString)); try { // Create JSON-LD object based on schema.org structured data String structuredData = new JSONObject() .put("@type", "Article") .put("name", "ExampleName of blog post") .put("url", outContent.getWebUri()) .toString(); outContent.setStructuredData(structuredData); } catch (JSONException ex) { // Handle exception Log.e(TAG, ex.getMessage()); } super.onProvideAssistContent(outContent); }
When implementing onProvideAssistContent()
, provide as much
data as possible about each entity
. The following
fields are required:
@type
.name
.url
(only required if the content is URL-addressable)
To learn more about using onProvideAssistContent()
, see the
Optimizing Contextual Content for the Assistant guide in
the Android developer documentation.