This guide shows you how to use the Google Mobile Ads SDK to load and display ads from DT Exchange using AdMob Mediation, covering waterfall integrations. It covers how to add DT Exchange to an ad unit's mediation configuration and how to integrate the DT Exchange SDK and adapter into an Android app.
Supported integrations and ad formats
The mediation adapter for DT Exchange has the following capabilities:
Integration | |
---|---|
Bidding | |
Waterfall | |
Formats | |
Banner | |
Interstitial | |
Rewarded | |
Native |
Requirements
- Android API level 21 or higher
Latest Google Mobile Ads SDK
Complete the mediation Get started guide
Step 1: Set up configurations in DT Exchange UI
Add new app and ad placement
Sign up or log in to DT Exchange Console.
Click on the Add App button to add your app.
Select your Platform, fill out the rest of the form, and click Add Placements.
Enter a name for the new placement in the field labeled Name your Placement and select your desired Placement Type from the dropdown list. Finally, click Save Placement.
App ID and Placement ID
Open the left navigation bar by hovering your mouse over it. Then click Apps from the left menu.
On the App Management page, take note of the App ID next to your app.
Select your app, navigate to the Placements tab, and click on the copy icon next to Placement Name. Take note of the Placement ID.
Publisher ID, Consumer Key and Consumer Secret
Click on your username from the left menu and select User Profile.
Take note of the Publisher ID, Consumer Key, and Consumer Secret as these are needed in the next step.
Step 2: Set up DT Exchange demand in AdMob UI
Configure mediation settings for your ad unit
You need to add DT Exchange to the mediation configuration for your ad unit.
First, sign in to your AdMob account. Next, navigate to the Mediation tab. If you have an existing mediation group you'd like to modify, click the name of that mediation group to edit it, and skip ahead to Add DT Exchange as an ad source.
To create a new mediation group, select Create Mediation Group.
Enter your ad format and platform, then click Continue.
Give your mediation group a name, and select locations to target. Next, set the mediation group status to Enabled, and then click Add Ad Units.
Associate this mediation group with one or more of your existing AdMob ad units. Then click Done.
You should now see the ad units card populated with the ad units you selected:
Add DT Exchange as an ad source
Under the Waterfall card in the Ad Sources section, select Add Ad Source. Then select DT Exchange.
Select DT Exchange and enable the Optimize switch. Enter the Publisher ID, Consumer Secret and Consumer Key obtained in the previous section to set up ad source optimization for DT Exchange. Then enter an eCPM value for DT Exchange and click Continue.
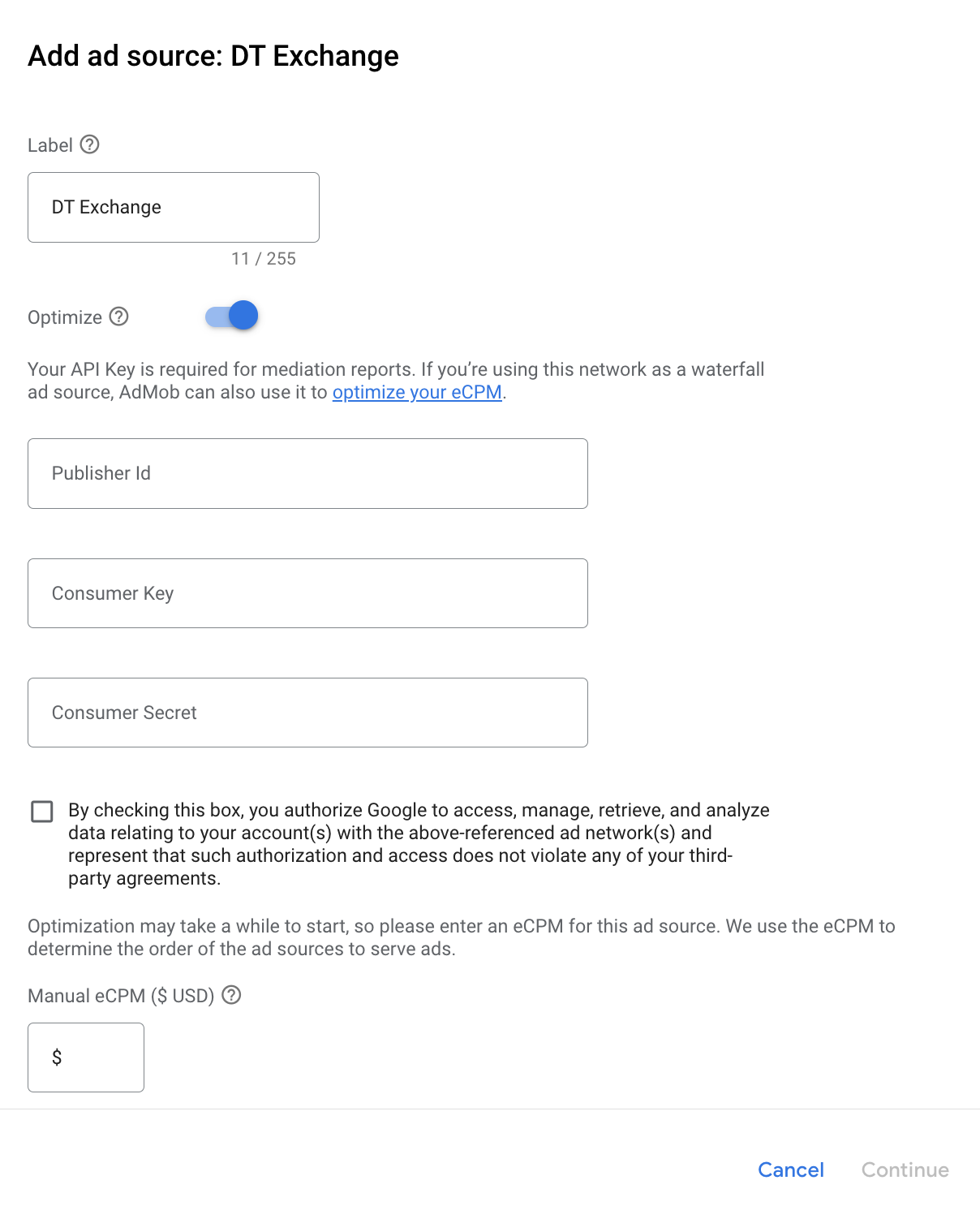
If you already have a mapping for DT Exchange, you can select it. Otherwise, click Add mapping.
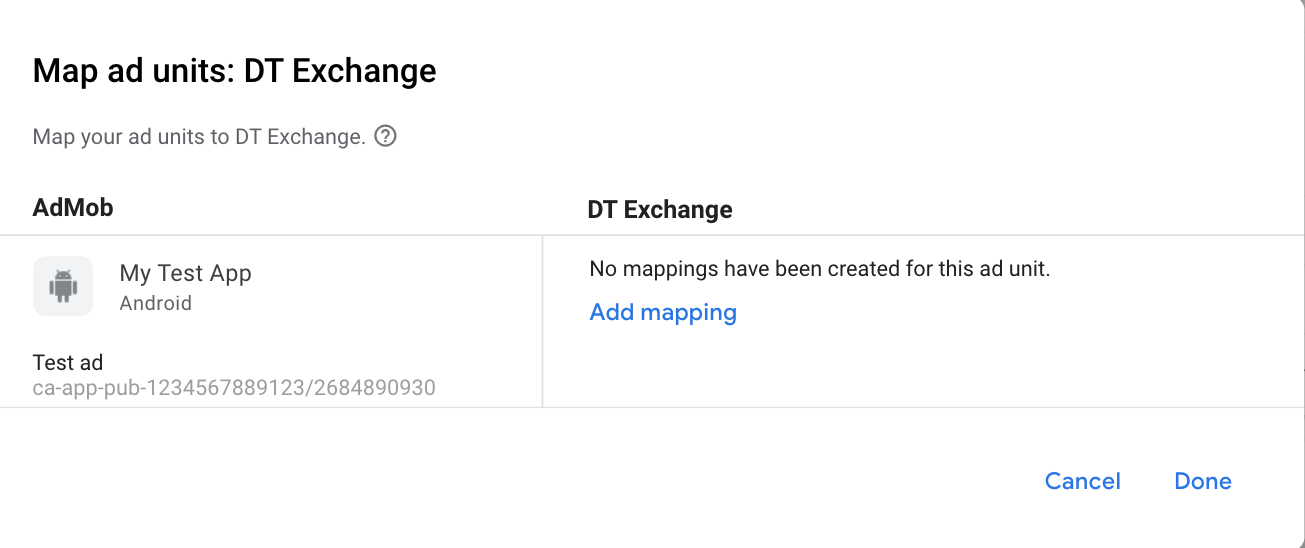
Next, enter the Application ID and Placement ID obtained in the previous section. Then click Done.
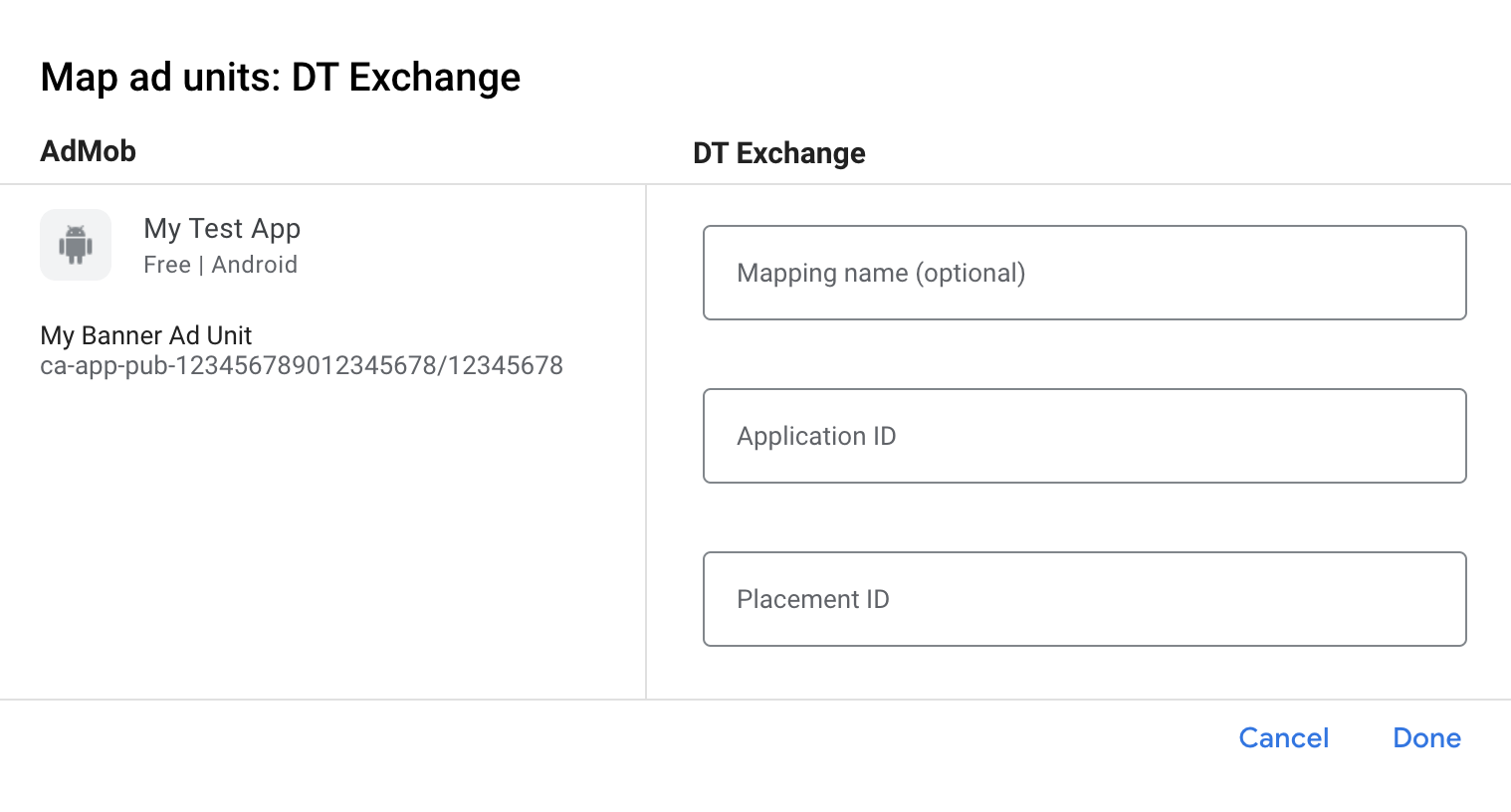
Add DT Exchange (Fyber Monetization) to GDPR and US state regulations ad partners list
Follow the steps in GDPR settings and US state regulations settings to add DT Exchange (Fyber Monetization) to the GDPR and US state regulations ad partners list in the AdMob UI.
Step 3: Import the DT Exchange SDK and adapter
Android Studio integration (recommended)
In your app-level build.gradle.kts
file, add the following implementation
dependencies and configurations. Use the latest versions of the DT Exchange SDK
and adapter:
dependencies {
implementation("com.google.android.gms:play-services-ads:23.5.0")
implementation("com.google.ads.mediation:fyber:8.3.3.0")
}
Manual integration
- Download the latest version of the
DT Exchange SDK for Android and add
the following files to your project:
ia-mraid-kit-release-x.y.z.aar
ia-sdk-core-release-x.y.z.aar
ia-video-kit-release-x.y.z.aar
- Navigate to the
DT Exchange adapter artifacts
on Google's Maven Repository. Select the latest version, download the DT
Exchange adapter's
.aar
file, and add it to your project.
Step 4: Implement privacy settings on DT Exchange SDK
EU consent and GDPR
Under the Google EU User Consent Policy, you must ensure that certain disclosures are given to, and consents obtained from, users in the European Economic Area (EEA) regarding the use of device identifiers and personal data. This policy reflects the requirements of the EU ePrivacy Directive and the General Data Protection Regulation (GDPR). When seeking consent, you must identify each ad network in your mediation chain that may collect, receive, or use personal data and provide information about each network's use. Google currently is unable to pass the user's consent choice to such networks automatically.
DT Exchange contains an API that lets you forward user consent to their SDK. The following sample code shows how to pass consent information to the DT Exchange SDK manually. Should you choose to pass consent information to the DT Exchange SDK manually, it is recommended that this code is called prior to requesting ads through the Google Mobile Ads SDK.
Java
import com.fyber.inneractive.sdk.external.InneractiveAdManager;
// ...
InneractiveAdManager.setGdprConsent(true);
InneractiveAdManager.setGdprConsentString("myGdprConsentString");
Kotlin
import com.fyber.inneractive.sdk.external.InneractiveAdManager
// ...
InneractiveAdManager.setGdprConsent(true)
InneractiveAdManager.setGdprConsentString("myGdprConsentString")
Visit DT Exchange's GDPR Resource Page and their GDPR implementation guide for more details and the values that may be provided in the method.
US states privacy laws
U.S. states privacy laws require giving users the right to opt out of the "sale" of their "personal information" (as the law defines those terms), with the opt-out offered via a prominent "Do Not Sell My Personal Information" link on the "selling" party's homepage. The U.S. states privacy laws compliance guide offers the ability to enable restricted data processing for Google ad serving, but Google is unable to apply this setting to each ad network in your mediation chain. Therefore, you must identify each ad network in your mediation chain that may participate in the sale of personal information and follow guidance from each of those networks to ensure compliance.
DT Exchange contains an API that lets you forward user consent to their SDK. The following sample code shows how to pass consent information to the DT Exchange SDK manually. Should you choose to pass consent information to the DT Exchange SDK manually, it is recommended that this code is called prior to requesting ads through the Google Mobile Ads SDK.
Java
import com.fyber.inneractive.sdk.external.InneractiveAdManager;
// ...
InneractiveAdManager.setUSPrivacyString("myUSPrivacyString");
Kotlin
import com.fyber.inneractive.sdk.external.InneractiveAdManager
// ...
InneractiveAdManager.setUSPrivacyString("myUSPrivacyString")
Visit DT Exchange's CCPA Resource Page and their CCPA implementation guide for more details and the values that may be provided in the method.
Step 5: Add required code
No additional code is required for DT Exchange integration.
Step 6: Test your implementation
Enable test ads
Make sure you register your test device for AdMob.
Verify test ads
To verify that you are receiving test ads from DT Exchange, enable single ad source testing in ad inspector using the DT Exchange (Waterfall) ad source(s).
Optional steps
Network-specific parameters
The DT Exchange adapter supports additional request parameters that can be passed to the adapter as an Android Bundle. The adapter looks for the following keys in the bundle:
Request parameters and values | |
---|---|
InneractiveMediationDefs.KEY_AGE
|
Integer. The age of the user |
FyberMediationAdapter.KEY_MUTE_VIDEO
|
Boolean. Mute or unmute video |
Here's a code example of how to set these ad request parameters:
Java
Bundle extras = new Bundle();
extras.putInt(InneractiveMediationDefs.KEY_AGE, 10);
extras.putBoolean(FyberMediationAdapter.KEY_MUTE_VIDEO, false);
AdRequest request = new AdRequest.Builder()
.addNetworkExtrasBundle(FyberMediationAdapter.class, extras)
.build();
Kotlin
var extras = Bundle()
extras.putInt(InneractiveMediationDefs.KEY_AGE, 10)
extras.putBoolean(FyberMediationAdapter.KEY_MUTE_VIDEO, false)
val request = AdRequest.Builder()
.addNetworkExtrasBundle(FyberMediationAdapter::class.java, extras)
.build()
Error codes
If the adapter fails to receive an ad from DT Exchange, you can check the
underlying error from the ad response using
ResponseInfo.getAdapterResponses()
under the following class:
com.google.ads.mediation.fyber.FyberMediationAdapter
Here are the codes and accompanying messages thrown by the DT Exchange adapter when an ad fails to load:
Error code | Reason |
---|---|
101 | DT Exchange server parameters configured in the AdMob UI are missing/invalid. |
103 | The requested ad size does not match a DT Exchange supported banner size. |
105 | DT Exchange SDK loaded an ad but returned an unexpected controller. |
106 | Ad is not ready to display. |
200-399 | DT Exchange SDK returned an error. See code for more details. |
DT Exchange Android Mediation Adapter Changelog
Version 8.3.3.0
- Verified compatibility with DT Exchange SDK 8.3.3.
Built and tested with:
- Google Mobile Ads SDK version 23.5.0.
- DT Exchange SDK version 8.3.3.
Version 8.3.2.0
- Verified compatibility with DT Exchange SDK 8.3.2.
Built and tested with:
- Google Mobile Ads SDK version 23.4.0.
- DT Exchange SDK version 8.3.2.
Version 8.3.1.0
- Verified compatibility with DT Exchange SDK 8.3.1.
Built and tested with:
- Google Mobile Ads SDK version 23.3.0.
- DT Exchange SDK version 8.3.1.
Version 8.3.0.0
- Added support for passing the Google Mobile Ads SDK version to DT Exchange SDK.
- Verified compatibility with DT Exchange SDK 8.3.0.
Built and tested with:
- Google Mobile Ads SDK version 23.2.0.
- DT Exchange SDK version 8.3.0.
Version 8.2.7.0
- Verified compatibility with DT Exchange SDK 8.2.7.
Built and tested with:
- Google Mobile Ads SDK version 23.0.0.
- DT Exchange SDK version 8.2.7.
Version 8.2.6.1
- Updated the minimum required Google Mobile Ads SDK version to 23.0.0.
- Verified compatibility with DT Exchange SDK 8.2.6.
Built and tested with:
- Google Mobile Ads SDK version 23.0.0.
- DT Exchange SDK version 8.2.6.
Version 8.2.6.0
- Verified compatibility with DT Exchange SDK 8.2.6.
Built and tested with:
- Google Mobile Ads SDK version 22.6.0.
- DT Exchange SDK version 8.2.6.
Version 8.2.5.0
- Verified compatibility with DT Exchange SDK 8.2.5.
Built and tested with:
- Google Mobile Ads SDK version 22.5.0.
- DT Exchange SDK version 8.2.5.
Version 8.2.4.0
- Verified compatibility with DT Exchange SDK 8.2.4.
Built and tested with:
- Google Mobile Ads SDK version 22.3.0.
- DT Exchange SDK version 8.2.4.
Version 8.2.3.0
- Updated adapter to use new
VersionInfo
class. - Updated the minimum required Google Mobile Ads SDK version to 22.0.0.
Built and tested with:
- Google Mobile Ads SDK version 22.0.0.
- DT Exchange SDK version 8.2.3.
Version 8.2.2.1
- Added support for passing
muteVideo
key in mediation extra to mute interstitial ads.
Built and tested with:
- Google Mobile Ads SDK version 21.5.0.
- DT Exchange SDK version 8.2.2.
Version 8.2.2.0
- Verified compatibility with DT Exchange SDK 8.2.2.
- Updated the minimum required Google Mobile Ads SDK version to 21.5.0.
Built and tested with:
- Google Mobile Ads SDK version 21.5.0.
- DT Exchange SDK version 8.2.2.
Version 8.2.1.0
- Rebranded adapter name to "DT Exchange".
- Verified compatibility with DT Exchange SDK 8.2.1.
- Updated the minimum required Google Mobile Ads SDK version to 21.3.0.
Built and tested with:
- Google Mobile Ads SDK version 21.3.0.
- DT Exchange SDK version 8.2.1.
Version 8.2.0.0
- Verified compatibility with Fyber SDK 8.2.0.
- Updated the minimum required Google Mobile Ads SDK version to 21.1.0.
Built and tested with:
- Google Mobile Ads SDK version 21.1.0.
- Fyber SDK version 8.2.0.
Version 8.1.5.0
- Verified compatibility with Fyber SDK 8.1.5.
Built and tested with:
- Google Mobile Ads SDK version 21.0.0.
- Fyber SDK version 8.1.5.
Version 8.1.4.0
- Verified compatibility with Fyber SDK 8.1.4.
Built and tested with:
- Google Mobile Ads SDK version 21.0.0.
- Fyber SDK version 8.1.4.
Version 8.1.3.1
- Updated
compileSdkVersion
andtargetSdkVersion
to API 31. - Updated the minimum required Google Mobile Ads SDK version to 21.0.0.
- Updated the minimum required Android API level to 19.
Built and tested with:
- Google Mobile Ads SDK version 21.0.0.
- Fyber SDK version 8.1.3.
Version 8.1.3.0
- Verified compatibility with Fyber SDK 8.1.3.
- Updated the minimum required Google Mobile Ads SDK version to 20.6.0.
Built and tested with:
- Google Mobile Ads SDK version 20.6.0.
- Fyber SDK version 8.1.3.
Version 8.1.2.0
- Verified compatibility with Fyber SDK 8.1.2.
- Updated the minimum required Google Mobile Ads SDK version to 20.5.0.
Built and tested with:
- Google Mobile Ads SDK version 20.5.0.
- Fyber SDK version 8.1.2.
Version 8.1.0.0
- Verified compatibility with Fyber SDK 8.1.0.
Built and tested with:
- Google Mobile Ads SDK version 20.4.0.
- Fyber SDK version 8.1.0.
Version 7.8.4.1
- Fixed an issue where the adapter would not initialize if there is a duplicate or more than one unique app ID from the mediation configurations.
- Updated the minimum required Google Mobile Ads SDK version to 20.4.0.
Built and tested with:
- Google Mobile Ads SDK version 20.4.0.
- Fyber SDK version 7.8.4.
Version 7.8.4.0
- Verified compatibility with Fyber SDK 7.8.4.
- Updated the minimum required Google Mobile Ads SDK version to 20.3.0.
- Fixed an issue with rewarded display ads, where
onVideoComplete()
was not called. - Fyber now requires an Activity context to show ads.
Built and tested with:
- Google Mobile Ads SDK version 20.3.0.
- Fyber SDK version 7.8.4.
Version 7.8.3.0
- Verified compatibility with Fyber SDK 7.8.3.
- Added standardized adapter error codes and messages.
- Updated the minimum required Google Mobile Ads SDK version to 20.1.0.
Built and tested with:
- Google Mobile Ads SDK version 20.1.0.
- Fyber SDK version 7.8.3.
Version 7.8.2.0
- Verified compatibility with Fyber SDK 7.8.2.
- Updated the minimum required Google Mobile Ads SDK version to 20.0.0.
Built and tested with:
- Google Mobile Ads SDK version 20.0.0.
- Fyber SDK version 7.8.2.
Version 7.8.1.0
- Verified compatibility with Fyber SDK 7.8.1.
Built and tested with:
- Google Mobile Ads SDK version 19.7.0.
- Fyber SDK version 7.8.1.
Version 7.8.0.0
- Verified compatibility with Fyber SDK 7.8.0.
- Updated the minimum required Google Mobile Ads SDK version to 19.7.0.
Built and tested with:
- Google Mobile Ads SDK version 19.7.0.
- Fyber SDK version 7.8.0.
Version 7.7.4.0
- Verified compatibility with Fyber SDK 7.7.4.
- Fixed an issue where
onUserEarnedReward()
is forwarded twice for rewarded ads.
Built and tested with:
- Google Mobile Ads SDK version 19.5.0.
- Fyber SDK version 7.7.4.
Version 7.7.3.0
- Verified compatibility with Fyber SDK 7.7.3.
Built and tested with:
- Google Mobile Ads SDK version 19.5.0.
- Fyber SDK version 7.7.3.
Version 7.7.2.0
- Verified compatibility with Fyber SDK 7.7.2.
- Updated the minimum required Google Mobile Ads SDK version to 19.5.0.
Built and tested with:
- Google Mobile Ads SDK version 19.5.0.
- Fyber SDK version 7.7.2.
Version 7.7.1.0
- Verified compatibility with Fyber SDK 7.7.1.
- Updated the minimum required Google Mobile Ads SDK version to 19.4.0.
Built and tested with:
- Google Mobile Ads SDK version 19.4.0.
- Fyber SDK version 7.7.1.
Version 7.7.0.0
- Verified compatibility with Fyber SDK 7.7.0.
Built and tested with:
- Google Mobile Ads SDK version 19.3.0.
- Fyber SDK version 7.7.0.
Version 7.6.1.0
- Verified compatibility with Fyber SDK 7.6.1.
Built and tested with:
- Google Mobile Ads SDK version 19.3.0.
- Fyber SDK version 7.6.1.
Version 7.6.0.0
- Verified compatibility with Fyber SDK 7.6.0.
- Updated the adapter to support inline adaptive banner requests.
- Updated the minimum required Google Mobile Ads SDK version to 19.3.0.
- Fixed a bug where onAdOpened()/onAdClosed() methods were not being called for banner ads.
Built and tested with:
- Google Mobile Ads SDK version 19.3.0.
- Fyber SDK version 7.6.0.
Version 7.5.4.0
- Verified compatibility with Fyber SDK 7.5.4.
- Updated the minimum required Google Mobile Ads SDK version to 19.1.0.
Built and tested with:
- Google Mobile Ads SDK version 19.1.0.
- Fyber SDK version 7.5.4.
Version 7.5.3.0
- Verified compatibility with Fyber SDK 7.5.3.
Built and tested with:
- Google Mobile Ads SDK version 19.0.1.
- Fyber SDK version 7.5.3.
Version 7.5.2.0
- Verified compatibility with Fyber SDK 7.5.2.
- Updated the minimum required Google Mobile Ads SDK version to 19.0.1.
Built and tested with:
- Google Mobile Ads SDK version 19.0.1.
- Fyber SDK version 7.5.2.
Version 7.5.0.0
- Verified compatibility with Fyber SDK 7.5.0.
- Fixed NullPointerException crash during Fyber ad loading.
Built and tested with:
- Google Mobile Ads SDK version 18.3.0.
- Fyber SDK version 7.5.0.
Version 7.4.1.0
- Verified compatibility with Fyber SDK 7.4.1.
- Updated the minimum required Google Mobile Ads SDK version to 18.3.0.
Built and tested with:
- Google Mobile Ads SDK version 18.3.0.
- Fyber SDK version 7.4.1.
Version 7.3.4.0
- Initial release!
- Supports Banner, Interstitial and Rewarded ads.
Built and tested with:
- Google Mobile Ads SDK version 18.2.0.
- Fyber SDK version 7.3.4.