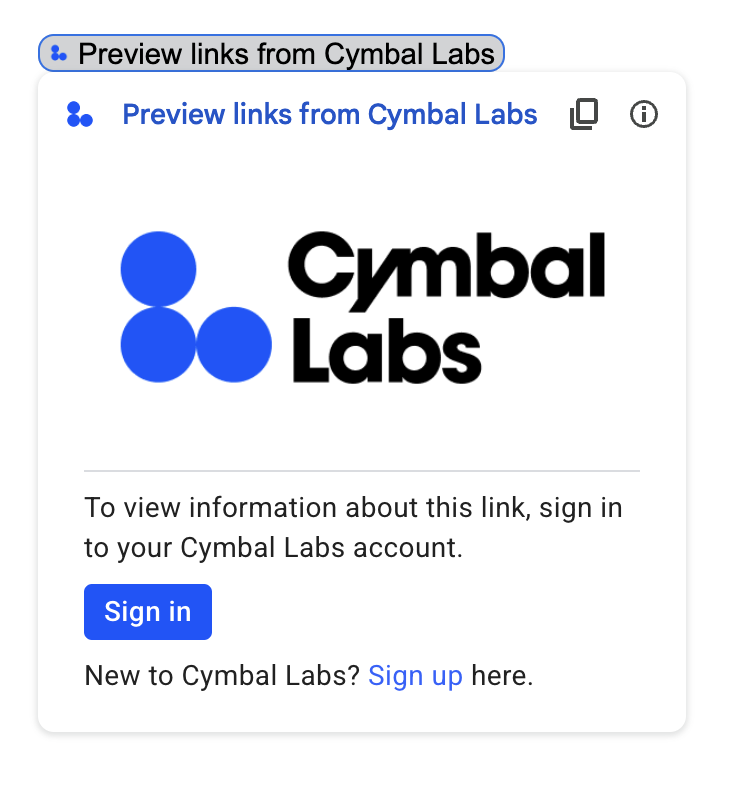
Jika Add-on Google Workspace Anda terhubung ke layanan atau API pihak ketiga yang memerlukan otorisasi, add-on dapat meminta pengguna untuk login dan mengizinkan akses.
Halaman ini menjelaskan cara mengautentikasi pengguna menggunakan alur otorisasi (seperti OAuth), yang mencakup langkah-langkah berikut:
- Mendeteksi kapan otorisasi diperlukan.
- Menampilkan antarmuka kartu yang meminta pengguna untuk login ke layanan.
- Muat ulang add-on agar pengguna dapat mengakses layanan atau resource yang dilindungi.
Jika add-on hanya memerlukan identitas pengguna, Anda dapat langsung mengautentikasi pengguna menggunakan ID Google Workspace atau alamat email mereka. Agar dapat menggunakan alamat email untuk autentikasi, lihat memvalidasi permintaan JSON. Jika Anda membuat add-on menggunakan Google Apps Script, buka dokumentasi Apps Script untuk mempelajari cara menghubungkan add-on ke layanan pihak ketiga.
Mendeteksi bahwa otorisasi diperlukan
Saat menggunakan add-on, pengguna mungkin tidak diberi otorisasi untuk mengakses resource yang dilindungi karena berbagai alasan, seperti berikut:
- Token akses untuk terhubung ke layanan pihak ketiga belum dibuat atau habis masa berlakunya.
- Token akses tidak mencakup resource yang diminta.
- Token akses tidak mencakup cakupan yang diperlukan dalam permintaan.
Add-on Anda harus mendeteksi kasus ini sehingga pengguna dapat login dan mengakses layanan Anda.
Minta pengguna untuk login ke layanan Anda
Saat add-on mendeteksi bahwa otorisasi diperlukan, add-on harus menampilkan antarmuka kartu untuk meminta pengguna login ke layanan. Kartu login harus mengalihkan pengguna untuk menyelesaikan proses autentikasi dan otorisasi pihak ketiga di infrastruktur Anda.
Sebaiknya jaga aplikasi tujuan dengan Login dengan Google, dan dapatkan ID pengguna menggunakan token identitas yang dikeluarkan selama login. Sub-klaim berisi ID unik pengguna dan dapat dihubungkan dengan ID dari add-on Anda.
Membuat dan mengembalikan kartu login
Untuk kartu login layanan, Anda dapat menggunakan kartu otorisasi dasar Google, atau Anda dapat menyesuaikan kartu untuk menampilkan informasi tambahan, seperti logo organisasi. Jika memublikasikan add-on secara publik, Anda harus menggunakan kartu kustom.
Kartu otorisasi dasar
Gambar berikut menampilkan contoh kartu otorisasi dasar Google:
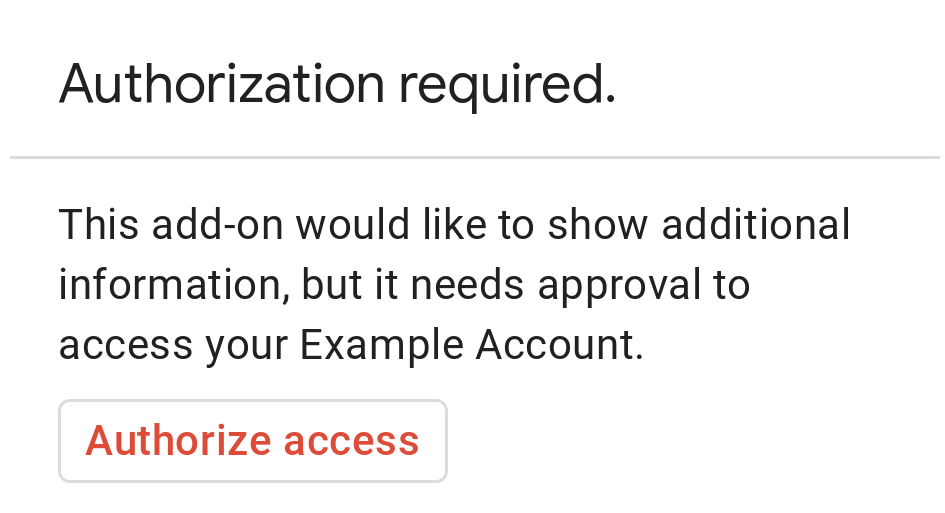
Untuk menggunakan kartu otorisasi dasar, tampilkan respons JSON berikut:
{ "basic_authorization_prompt": { "authorization_url": "AUTHORIZATION_REDIRECT", "resource": "RESOURCE_DISPLAY_NAME" } }
Ganti kode berikut:
AUTHORIZATION_REDIRECT
: URL untuk aplikasi web yang menangani otorisasi.RESOURCE_DISPLAY_NAME
: Nama tampilan untuk resource atau layanan yang dilindungi. Nama ini akan ditampilkan kepada pengguna di perintah otorisasi. Misalnya, jikaRESOURCE_DISPLAY_NAME
Anda adalahExample Account
, dialog akan muncul, "Add-on ini ingin menampilkan informasi tambahan, tetapi memerlukan persetujuan untuk mengakses Akun Contoh Anda".
Setelah menyelesaikan otorisasi, pengguna diminta untuk memuat ulang add-on agar dapat mengakses resource yang dilindungi.
Kartu otorisasi khusus
Untuk mengubah permintaan otorisasi, Anda dapat membuat kartu kustom untuk pengalaman login layanan Anda.
Jika Anda memublikasikan add-on secara publik, Anda harus menggunakan kartu otorisasi khusus. Untuk mempelajari lebih lanjut persyaratan publikasi untuk Google Workspace Marketplace, lihat Tentang peninjauan aplikasi.
Gambar berikut menampilkan contoh kartu otorisasi kustom untuk halaman beranda add-on. Kartu ini menyertakan logo, deskripsi, dan tombol login:
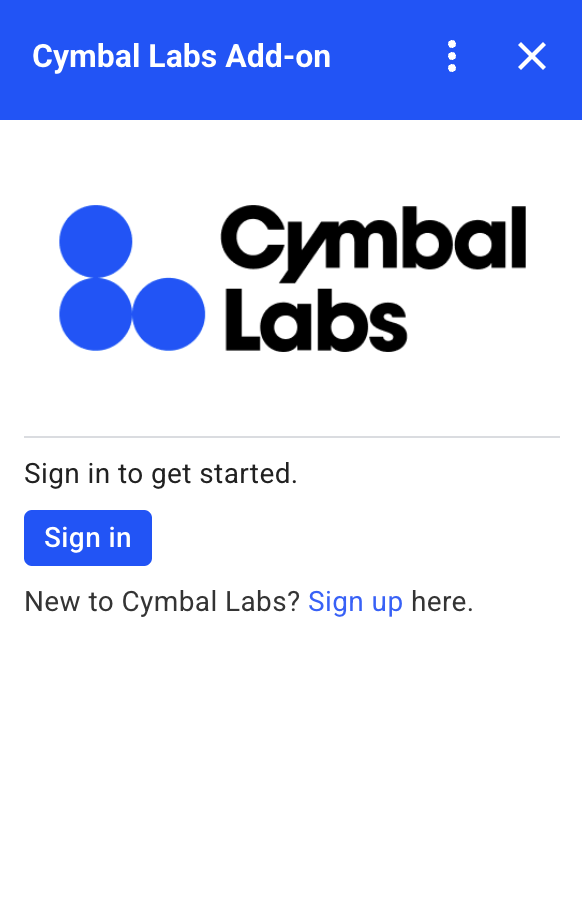
Untuk menggunakan contoh kartu kustom ini, tampilkan respons JSON berikut:
{ "custom_authorization_prompt": { "action": { "navigations": [ { "pushCard": { "sections": [ { "widgets": [ { "image": { "imageUrl": "LOGO_URL", "altText": "LOGO_ALT_TEXT" } }, { "divider": {} }, { "textParagraph": { "text": "DESCRIPTION" } }, { "buttonList": { "buttons": [ { "text": "Sign in", "onClick": { "openLink": { "url": "AUTHORIZATION_REDIRECT", "onClose": "RELOAD", "openAs": "OVERLAY" } }, "color": { "red": 0, "green": 0, "blue": 1, "alpha": 1, } } ] } }, { "textParagraph": { "text": "TEXT_SIGN_UP" } } ] } ] } } ] } } }
Ganti kode berikut:
LOGO_URL
: URL untuk logo atau gambar. Harus berupa URL publik.LOGO_ALT_TEXT
: Teks alternatif untuk logo atau gambar, sepertiCymbal Labs Logo
.DESCRIPTION
: Pesan ajakan (CTA) bagi pengguna untuk login, sepertiSign in to get started
.- Untuk memperbarui tombol login:
AUTHORIZATION_REDIRECT
: URL untuk aplikasi web yang menangani otorisasi.- Opsional: Untuk mengubah warna tombol, perbarui nilai float RGBA
kolom
color
.
TEXT_SIGN_UP
: Teks yang meminta pengguna membuat akun jika mereka belum memilikinya. Misalnya,New to Cymbal Labs? <a href=\"https://www.example.com/signup\">Sign up</a> here
.