Places UI Kit: Place Details (Experimental)
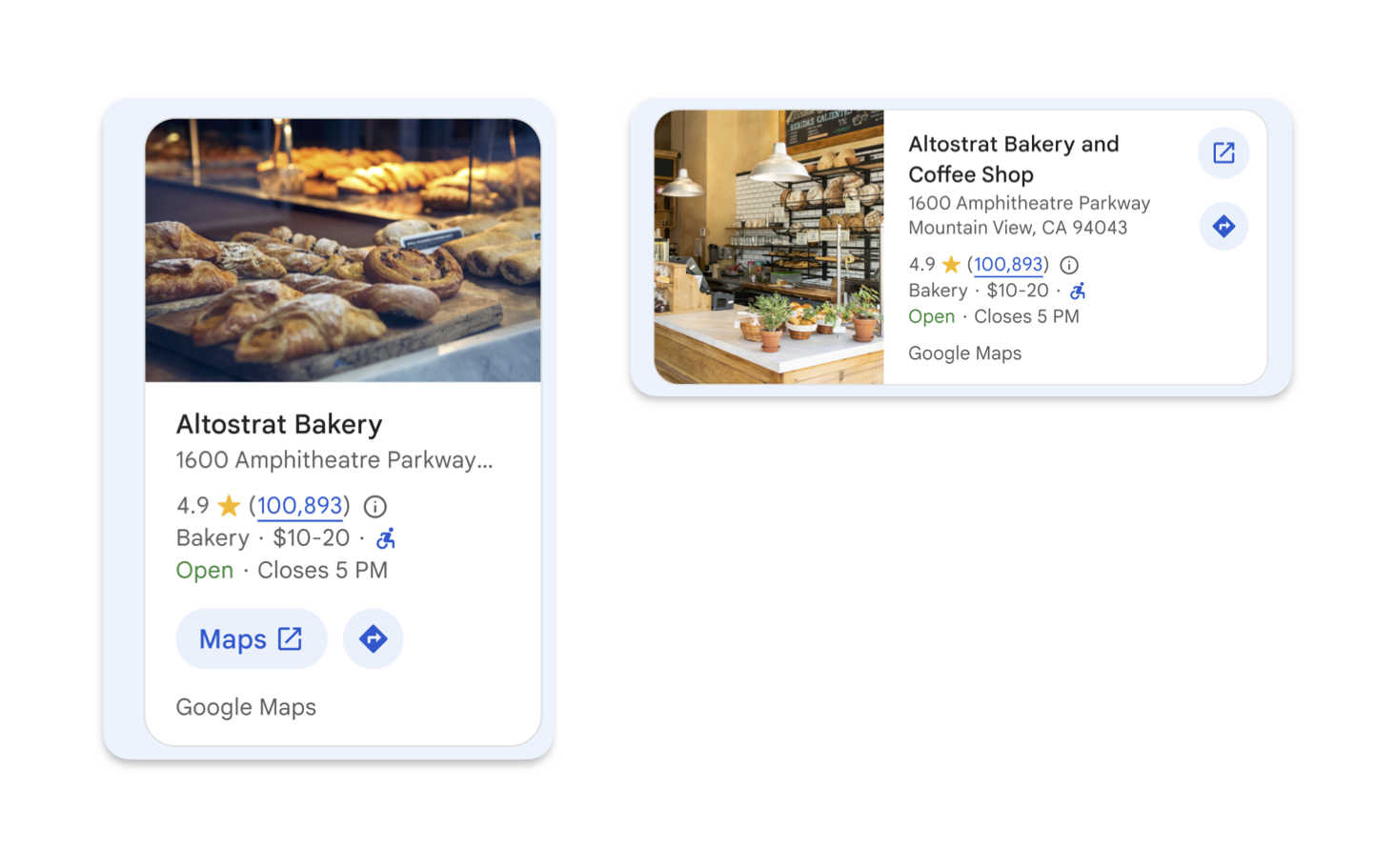
The Place Details UI Kit for Place Details lets you add an individual UI component that displays place details in your app. The UI kit can be used independently or in conjunction with other Google Maps Platform APIs and services. The UI kit takes either a Place ID or latitude/longitude coordinates and returns rendered Place Details information.
The UI kit offers a compact view, which can be displayed horizontally or vertically. You can customize the appearance of the place details by overriding any of the attributes of the default theme. You can also customize which place details fields are included by specifying a list of Content
entries, each of which corresponds to a piece of information shown about the place.
Billing
When using the Place Details UI Kit for Place Details, you are billed for each place that is loaded using the widget. If you load the same place multiple times, you are billed for each request.
Enable the Places UI Kit
Before using the Places UI Kit, you need to:
- Create a Cloud project with a billing account.
- Enable the Places API for your project.
- Get an API key.
Place Details UI Kit examples
You can add place details to your app by adding a fragment to a layout. When you instantiate the fragment, you can customize the look and feel of the place details information to suit your needs and match your app's appearance.
You can specify orientation (horizontal or vertical), theme overrides, and content. The content options are media, address, rating, price, type, accessible entrance, maps link, and directions link. [See a customization example]().
Kotlin
val fragment = PlaceDetailsCompactFragment.newInstance( orientation, listOf(Content.ADDRESS, Content.TYPE, Content.RATING, Content.ACCESSIBLE_ENTRANCE), R.style.CustomizedPlaceDetailsTheme, ) fragment.setPlaceLoadListener(object : PlaceLoadListener { override fun onSuccess() { ... } override fun onFailure(e: Exception) { ... } }) supportFragmentManager .beginTransaction() .add(R.id.fragment_container, fragment) .commitNow() fragment.loadPlaceDetails(placeId)
Java
PlaceDetailsCompactFragment fragment = PlaceDetailsCompactFragment.newInstance( Orientation.HORIZONTAL, Arrays.asList(Content.ADDRESS, Content.TYPE, Content.RATING, Content.ACCESSIBLE_ENTRANCE), R.style.CustomizedPlaceDetailsTheme); fragment.setPlaceLoadListener( new PlaceLoadListener() { @Override public void onSuccess() { ... } @Override public void onFailure(Exception e) { ... } }); getSupportFragmentManager() .beginTransaction() .add(R.id.fragment_container, fragment) .commitNow(); fragment.loadPlaceDetails(placeId);
Customization example
When instantiating a fragment, you can specify a theme that overrides any of the default style attributes. Any theme attributes that are not overridden use the default styles. If you'd like to support a dark theme, you can add an entry for the color in values-night/colors.xml
.
<style name="CustomizedPlaceDetailsTheme" parent="PlacesTheme"> <item name="placesColorPrimary">@color/app_primary_color</item> <item name="placesColorOnSurface">@color/app_color_on_surface</item> <item name="placesColorOnSurfaceVariant">@color/app_color_on_surface</item> <item name="placesTextAppearanceBodySmall">@style/app_text_appearence_small</item> <item name="placesCornerRadius">20dp</item> </style>
You can customize the following styles:
placesColorSurfaceContainerLowest
placesColorOutlineDecorative
placesColorPrimary
placesColorOnSurface
placesColorOnSurfaceVariant
placesColorSecondaryContainer
placesColorOnSecondaryContainer
placesCornerRadius
placesTextAppearanceBodySmall
placesTextAppearanceBodyMedium
placesTextAppearanceBodyLarge
placesTextAppearanceLabelLarge
placesTextAppearanceHeadlineMedium
placesColorAttributionLight
(enums for white, gray, and black)placesColorAttributionDark
(enums for white, gray, and black)
This sample customizes the standard content.
val fragmentStandardContent = PlaceDetailsCompactFragment.newInstance( orientation, PlaceDetailsCompactFragment.STANDARD_CONTENT, R.style.BrandedPlaceDetailsTheme, )
This sample customizes all content.
val fragmentAllContent = PlaceDetailsCompactFragment.newInstance( orientation, PlaceDetailsCompactFragment.ALL_CONTENT, R.style.BrandedPlaceDetailsTheme, )