Overview
Crosshairs are thin vertical and horizontal lines centered on a data point in a chart. When you, as a chart creator, enable crosshairs in your charts, your users will then be able to target a single element:
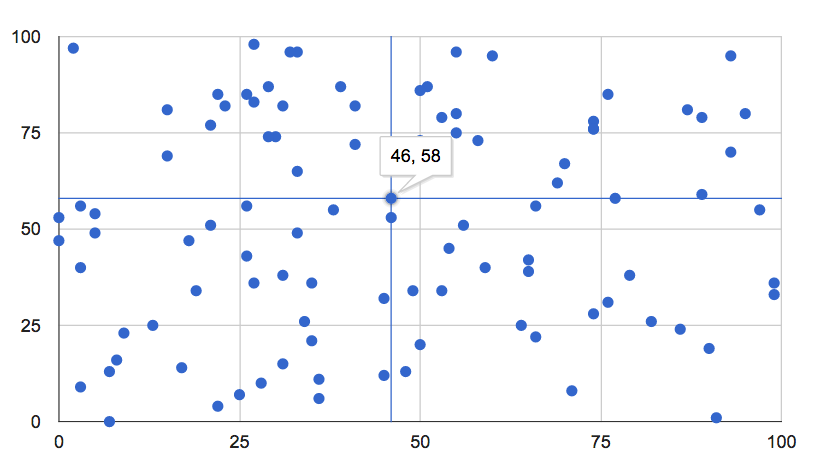
Crosshairs can appear on focus, selection, or both. They're available for scatter charts, line charts, area charts, and for the line and area portions of combo charts.
A Simple Example
Hover over the points below, or select them, to see crosshairs:
Here's a complete web page that creates the above chart, with the line for crosshairs in bold:
<html> <head> <script type="text/javascript" src="https://www.gstatic.com/charts/loader.js"></script> <script type="text/javascript"> google.charts.load("current", {packages:["corechart"]}); google.charts.setOnLoadCallback(drawChart); function drawChart() { var data = new google.visualization.DataTable(); data.addColumn('number'); data.addColumn('number'); for (var i = 0; i < 100; i++) data.addRow([Math.floor(Math.random()*100), Math.floor(Math.random()*100)]); var options = { legend: 'none', crosshair: { trigger: 'both' } // Display crosshairs on focus and selection. }; var chart = new google.visualization.ScatterChart(document.getElementById('chart_div')); chart.draw(data, options); } </script> </head> <body> <div id="chart_div" style="width: 900px; height: 500px;"></div> </body> </html>
Crosshair Options
The following crosshair options are available:
crosshair: { trigger: 'both' } | display on both focus and selection |
crosshair: { trigger: 'focus' } | display on focus only |
crosshair: { trigger: 'selection' } | display on selection only |
crosshair: { orientation: 'both' } | display both horizontal and vertical hairs |
crosshair: { orientation: 'horizontal' } | display horizontal hairs only |
crosshair: { orientation: 'vertical' } | display vertical hairs only |
crosshair: { color: color_string } | set crosshair color to color_string, e.g., 'red' or '#f00' |
crosshair: { opacity: opacity_number } | set crosshair opacity to opacity_number, with 0.0 being fully transparent and 1.0 fully opaque |
crosshair: { focused: { color: color_string } } | set crosshair color to color_string on focus |
crosshair: { focused: { opacity: opacity_number } } | set crosshair opacity to opacity_number on focus |
crosshair: { selected: { color: color_string } } | set crosshair color to color_string on selection |
crosshair: { selected: { opacity: opacity_number } } | set crosshair opacity to opacity_number on selection |