Google Fit provides a set of health and wellness data types under the com.google
namespace.
Data types define the format of the values inside data points. A data point can represent:
- An instantaneous reading or observation
- An aggregate with statistics over a time interval
Google Fit defines data types for instantaneous observations and data types for aggregate data. Data points consist of values for the fields of a data type and timestamp information. Points that represent instantaneous observations include a timestamp, and points of an aggregate data type also include the start time for the interval.
Google Fit also lets you define new data types.
Data type groups
Google Fit has these data types:
- Public data types
- Standard data types provided by the platform have the 'com.google' prefix. For example, com.google.step_count.delta. These data types capture instant readings for health and wellness data, including fitness activity, sleep and nutrition. Any app can request the relevant permissions to read and write to these data types, except for a few location data types which can only be read by the app that wrote them.
For more information, see:
- Health data types
- Data types provided by the platform that have restricted access due to potentially sensitive data. For more information, see Health data types.
- Aggregate data types
- Data types to read health and wellness information aggregated by time or activity type. For more information, see Aggregate data types.
- Private custom data types
- Custom data types defined by a specific app. Only the app that defines the data type can read and write data of this type. For more information, see Custom data types.
Using data types
Android
On Android, data types are defined as public fields of the
DataType
class. How you invoke the Fitness APIs with the data type depends on what you want to accomplish:
- To record data, use the Recording API to create a subscription for each data type you want to record.
- To read data, use the History API to submit a read request for each data type.
- To insert historical data from the past, use the History API to submit an insert request for each data type.
- To create sessions, use the Sessions API to insert or record data with session metadata.
To create data points for an instaneous
DataType
object, assign values with the correct format. The following example shows you
how to assign the food item as a string, the meal type as a constant from the
Field
class,
and nutrient contents as mapped float values.
val nutritionSource = DataSource.Builder() .setDataType(DataType.TYPE_NUTRITION) ... .build() val nutrients = mapOf( Field.NUTRIENT_TOTAL_FAT to 0.4f, Field.NUTRIENT_SODIUM to 1f, Field.NUTRIENT_POTASSIUM to 422f ) val banana = DataPoint.builder(nutritionSource) .setTimestamp(now, TimeUnit.MILLISECONDS) .setField(Field.FIELD_FOOD_ITEM, "banana") .setField(Field.FIELD_MEAL_TYPE, Field.MEAL_TYPE_SNACK) .setField(Field.FIELD_NUTRIENTS, nutrients) .build()
After setting data points in your app, you can insert, read, or delete historical data with the History API.
REST
The dataSources
resource includes the data type
(and a list of its fields) for each data source. You can specify one of these data types when
you create data sources, and you can obtain the name of the data type and a list of its fields
when you retrieve a data source from the fitness store.
For example, a data source representation specifies its data type as follows:
{ "dataStreamId": "exampleDataSourceId", ... "dataType": { "name": "com.google.step_count.delta" }, ... }
Authorization scopes
Authorization scopes cover groups of data types that a user can authorize an app to access. They help users understand what kinds of data an app wants to access. They also make it easier to give apps permission to use that data by not having to approve each individual data type. Users grant these permissions once they've downloaded your app.
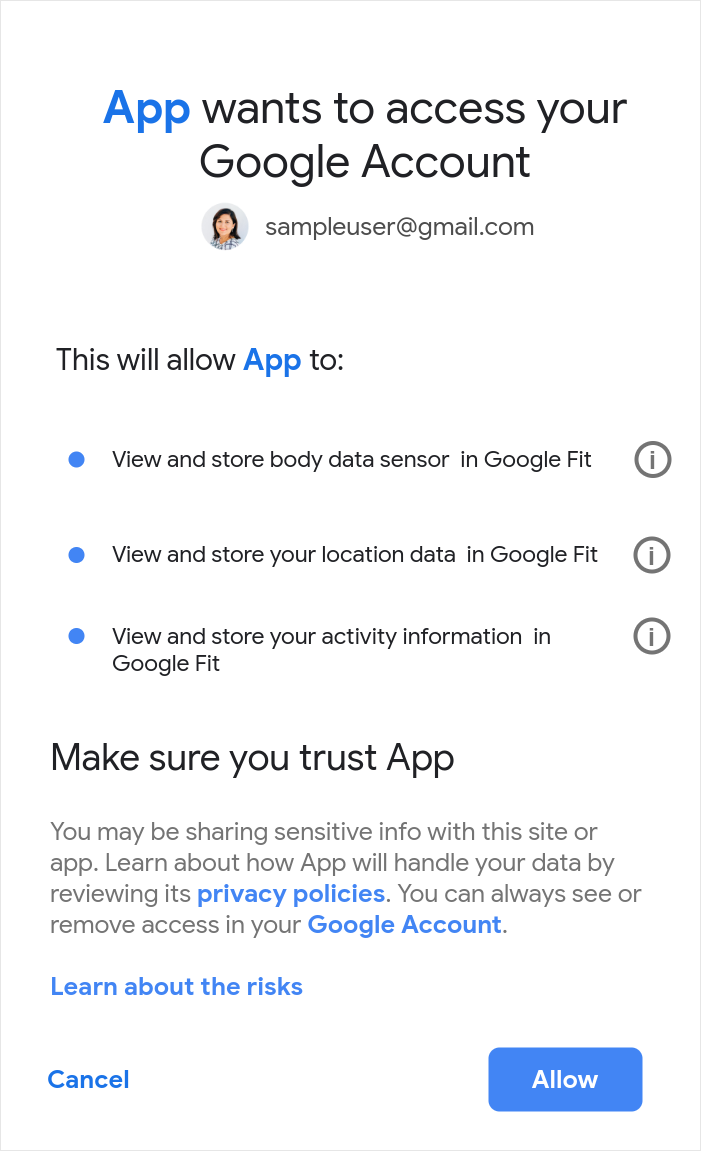
After you've tested your app with a small number of users, before launching your app you need to request verification based on the scopes relevant to those data types. Read through the data types pages linked above to understand which scopes apply to each data type.
For example, if your app needs to read and write writing blood pressure, it needs to declare it's doing both read and write and request both scopes. If it's only writing blood pressure data to the Google Fit platform, it only needs to request the write scope.
Choose data types responsibly. Don't request every data type in case your app might need it. The types specified determine which scopes the user is prompted to grant permission for. Only ask for the data types your app needs so users are more likely to grant access. Users more readily grant access to limited, clearly described scopes.
Use this table to check if the scopes your app needs access to are sensitive or restricted (which determines the verification steps you'll need to follow):
Scope | Description | Category |
---|---|---|
https://www.googleapis.com/auth/fitness.activity.read |
Read activity data from the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.activity.write |
Write activity data to the Google Fit platform | Restricted |
https://www.googleapis.com/auth/fitness.blood_glucose.read |
Read blood glucose data from the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.blood_glucose.write |
Write blood glucose data to the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.blood_pressure.read |
Read blood pressure data from the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.blood_pressure.write |
Write blood pressure data to the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.body.read |
Read body measurement data (height, weight, body fat percentage) from the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.body.write |
Write body measurement data to the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.body_temperature.read |
Read body temperature data from the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.body_temperature.write |
Write body temperature data to the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.heart_rate.read |
Read heart rate data from the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.heart_rate.write |
Write heart rate data to the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.location.read |
Read location data from the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.location.write |
Write location data to the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.nutrition.read |
Read nutrition data from the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.nutrition.write |
Write nutrition data to the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.oxygen_saturation.read |
Read oxygen saturation data from the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.oxygen_saturation.write |
Write oxygen saturation data to the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.reproductive_health.read |
Read reproductive health data from the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.reproductive_health.write |
Write reproductive health data to the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.sleep.read |
Read sleep data from the Google Fit platform. | Restricted |
https://www.googleapis.com/auth/fitness.sleep.write |
Write sleep data to the Google Fit platform. | Restricted |
Adding new scopes to an existing app
When you update your app to request a new scope (for example, if you add the new sleep or heart rate scopes, or add a read scope), users will be prompted that your app is requesting access to these scopes and they can choose to grant or reject access.
It's best practice to request authorization from users for resources at the time you need them. Follow the guidelines on requesting incremental authorization.
Users will be more likely to grant access if they understand why/how your app uses this data:
- Consider adding a screen that warns/informs users that they'll be asked for these scopes.
- Clearly explain why your app is asking for access to these scopes/data so users can make an informed decision.
Learn more about best practices around app permissions for Android.