Mediation is a common way for sell-side ad platforms to offer yield management. In a mediation workflow, the mediation SDK ('mediator') invokes multiple ad networks ('mediatee' or 'mediatees') to obtain the best ad for a given slot. In some cases, both the mediator and the ad networks it invokes need their SDKs to be on device and interact.
This document outlines key changes to the mediation workflows in the SDK Runtime. It covers the following topics:
- Differences between previous mediation flows and current SDK Runtime mediation support
- Setup actions for mediation workflows in the SDK Runtime, and the different stages of transition
- Guidance on how to handle scenarios where not all SDKs have migrated to the Runtime
Support for Mediated Ads in the SDK Runtime is available from AGP 8.5 and from the following versions of the SDK Runtime Jetpack libraries:
Androidx library | Version |
---|---|
androidx.privacysandbox.activity | 1.0.0-alpha01 |
androidx.privacysandbox.sdkruntime | 1.0.0-alpha13 |
androidx.privacysandbox.tools | 1.0.0-alpha08 |
androidx.privacysandbox.ui | 1.0.0-alpha09 |
Glossary
The following terms are key to understanding mediation in the SDK Runtime:
- Runtime-enabled SDK (RE SDK): An SDK made to run in the SDK Runtime environment and communicate with the app over inter-process communication (IPC).
- Runtime-aware SDK (RA SDK): A non-runtime-enabled SDK, linked to the app statically, which may contain your existing SDK code as well as new code to call into your runtime-enabled SDK.
- In-app SDK: An SDK that runs linked to the app statically, and has no awareness of the SDK Runtime. This may be an ad network that has not transitioned to SDK Runtime, or a publisher's custom adapter.
- Mediator: Ad mediation SDK that provides on-device a mediation service by interacting with other ad network SDKs.
- Mediatee: Ad network SDK that gets called by the Mediator to provide and render an ad.
- Mediation Adapter: SDKs utilized by the Mediator SDK to provide API interface translation to interoperate with various mediatee SDKs, usually provided by the mediator. These can be runtime aware or runtime unaware.
Typical mediation flows
If your SDK needs to support mediation use cases in the SDK Runtime, you will need to implement some changes. This section reviews the key elements of mediation flows so we can address the changes required for mediators and mediatees.
The flows we describe represent a simplified version of on-device mediation with multiple ad network SDKs, and serve as a basis for discussion on the changes required to make mediation journeys compatible with the SDK Runtime.
Given the variation in mediation flow implementations, we focus on the following two main flows:
- Initialization (including discovery of ad networks and communication)
- Ad user interface (UI) presentation
Initialization
The following represents a standard initialization, ad network discovery, and communication flow:
- The client app initiates the mediator
- The mediator discovers and initializes the relevant mediatees and adapters
- The mediator uses its adapters to communicate with each mediatee
- The client app requests the mediator to load an ad
- The client app requests the mediator to show this ad
Ad UI Presentation
When it comes to rendering the ad after the final request in the previous step, the flow depends on the type of ad:
Banner ads | Full-screen ads | Native ads |
---|---|---|
The mediator SDK creates an ad View, which wraps the winning mediatee's ad View. It may also set listeners on this View, or auto-refresh the ad (using the same or a different mediatee). |
The mediator SDK requests a full-screen ad from the mediatee, which in turn starts an Activity. | The publisher manages the view handling and inflating using components returned by the mediator SDK. |
Mediation flows in the SDK Runtime
How mediation works inside of the SDK Runtime is different depending on whether the mediatee is runtime-enabled or not. Based on this, we can have the following scenarios:
- Both mediator and mediatee are in the SDK Runtime: RE mediatee
- The mediator is in the SDK Runtime, and the mediatee is in-app: In-app mediatee
RE Mediatee
The following architecture diagram shows a high-level overview of the interaction of the mediator's runtime-enabled (RE) and runtime-aware (RA) SDKs, the RE mediation adapters, and the mediatees' RE SDKs.
Mediation adapters need to be in the same process as the mediatee they're interfacing with, so they will also have to migrate to the SDK Runtime.

Initialization
When considering the initialization, discovery, and communication of both runtime-enabled mediator and mediatee, the flow will follow these steps:
- The app (or the RA SDK) load and initialize the mediator SDK using
SdkSandboxManager#loadSdk
. - During its initialization, the mediator SDK loads and initializes any
required mediatees in the SDK Runtime using
SdkSandboxController#loadSdk
. - RE SDK can discover all loaded SDKs in the Runtime by calling
SdkSandboxController#getSandboxedSdks
.

Ad UI Presentation
The following section covers loading banners and full-screen ads from an RE mediatee.
RE Mediatee banner ads
Given a request from the app to load a banner ad, the flow to complete rendering is as follows:
- The mediator selects the winning mediatee for this ad.
- The mediator obtains a
SandboxedUiAdapter
from the mediatee. - The mediator forwards the UiAdapter to the app.

Learn more about the usage of SandboxedUiAdapter
and the SDK Runtime UI Library.
Overlays to banner ads
If mediators want to add an overlay to the ad, they would have to modify the flow as follows:
- The mediator creates a layout with its overlay and a
SandboxedSdkView
. - The mediator selects the winning mediatee for this ad.
- The mediator obtains a
SandboxedUiAdapter
from the mediatee. - The mediator sets the mediatee's
UiAdapter
to theSandboxedSdkView
. - The mediator shares the populated view with the app.
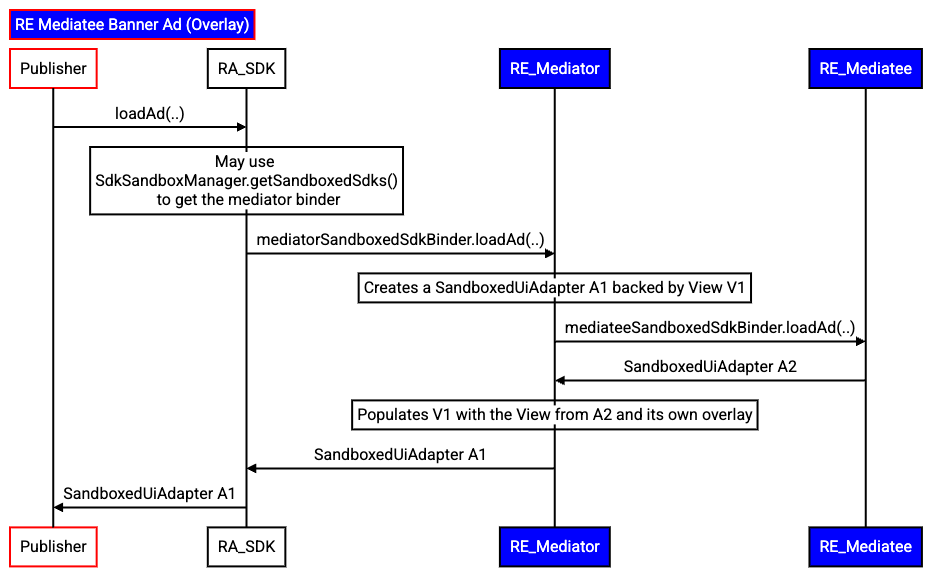
RE Mediatee full-screen ads
Given a request from the app to load a full-screen ad, the flow follows these steps:
- The app (or the RA SDK) passes an
SdkActivityLauncher
to the mediator with the request to load an ad.- The client can restrict the creation of activities using a predicate.
- The mediator selects the winning mediatee for this ad.
- The mediator requests the mediatee to load an ad, passing the
SdkActivityLauncher
from the app. - The mediatee registers an activity handler, and gets an identifier token for the registered activity.
- The mediatee uses the
SdkActivityLauncher
to request to start an activity using this token. - If the client app's predicate allows it, the SDK Runtime will start this activity in the dedicated process.

Learn more about Activity support for full-screen ads in the SDK Runtime.
In-app Mediatee
The following architecture diagram shows a high-level overview of the interaction of the mediator's RE and RA SDKs, of the mediation adapters that are unaware of the SDK Runtime, and the mediatees' SDKs statically linked to the app (also runtime-unaware).

Initialization
Since in this scenario mediatees are statically linked to the app and not yet migrated to the SDK Runtime, the mediator's runtime-enabled SDK should have a process to register them.
This registration should be accessible using the mediator's API, but the
implementation details are left to each mediator's discretion. We call this API
MediationSandboxedSdk#registerInAppMediatee
.
When considering the initialization, discovery, and communication of a RE mediator SDK and in-app mediatee SDKs, the flow will follow these steps:
- The app loads and initializes the mediator's runtime-aware SDK.
- The mediator's RA SDK:
- Initializes the mediator's RE SDK using
SdkSandboxManager#loadSdk
. - Initializes all in-app mediatee SDKs.
- Discovers and registers the in-app mediatee SDKs using the API provided
by the RE SDK,
MediationSandboxedSdk#registerInAppMediate
.
- Initializes the mediator's RE SDK using
Besides having all the in-app mediatee SDKs registered, the mediator's RE SDK
can discover all the SDKs loaded in the SDK Runtime using
SdkSandboxController#getSandboxedSdks
.
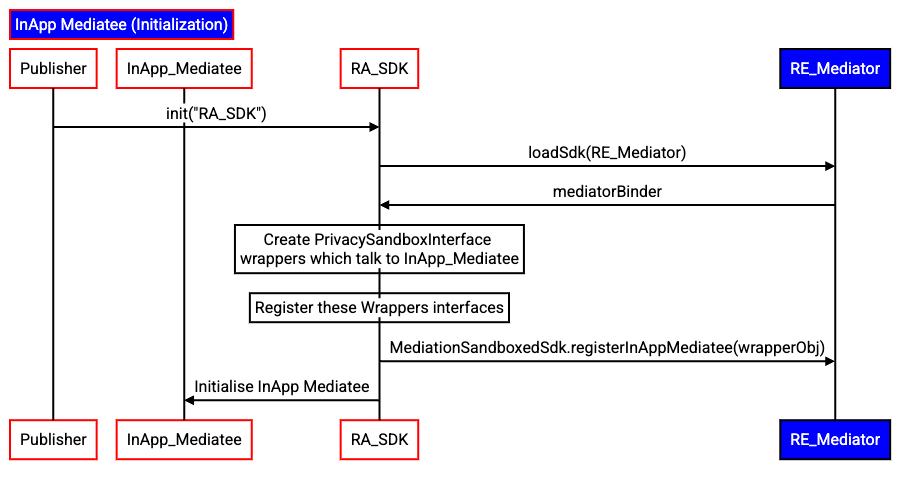
Ad UI Presentation
The following section covers loading banners and full-screen ads from an in-app mediatee.
In-app mediatee banner ads
Given a request from the app to load a banner ad, the flow to complete rendering is as follows:
- The mediator's runtime-aware SDK forwards the app's request to its runtime-enabled SDK.
- The mediator's RE SDK selects the relevant mediatee.
- The mediator's RE SDK retrieves the reference to the mediatee, and requests to load an ad through the RA SDK.
- The RA SDK obtains a View from the in-app mediatee.
- The RA SDK creates a
SandboxedUiAdapter
for the View it received. - The RA SDK forwards the
UiAdapter
to the RE SDK. - The RE SDK forwards the
UiAdapter
to the app.

In-app mediatee full-screen ads
Given a request from the app to load a full-screen ad, the flow follows these steps:
- The app passes an
SdkActivityLauncher
to the mediator's RA SDK with the request to load an ad.- The client can restrict the creation of activities using a predicate.
- The mediator's RA SDK forwards the app's request to its RE SDK.
- The mediator's RE SDK:
- Selects the relevant mediatee.
- Retrieves the reference to the in-app mediatee.
- Requests to load an ad through the RA SDK.
- The RA SDK requests the mediatee to load an ad.
- The mediatee starts the activity directly. The app's predicate won't be honored.
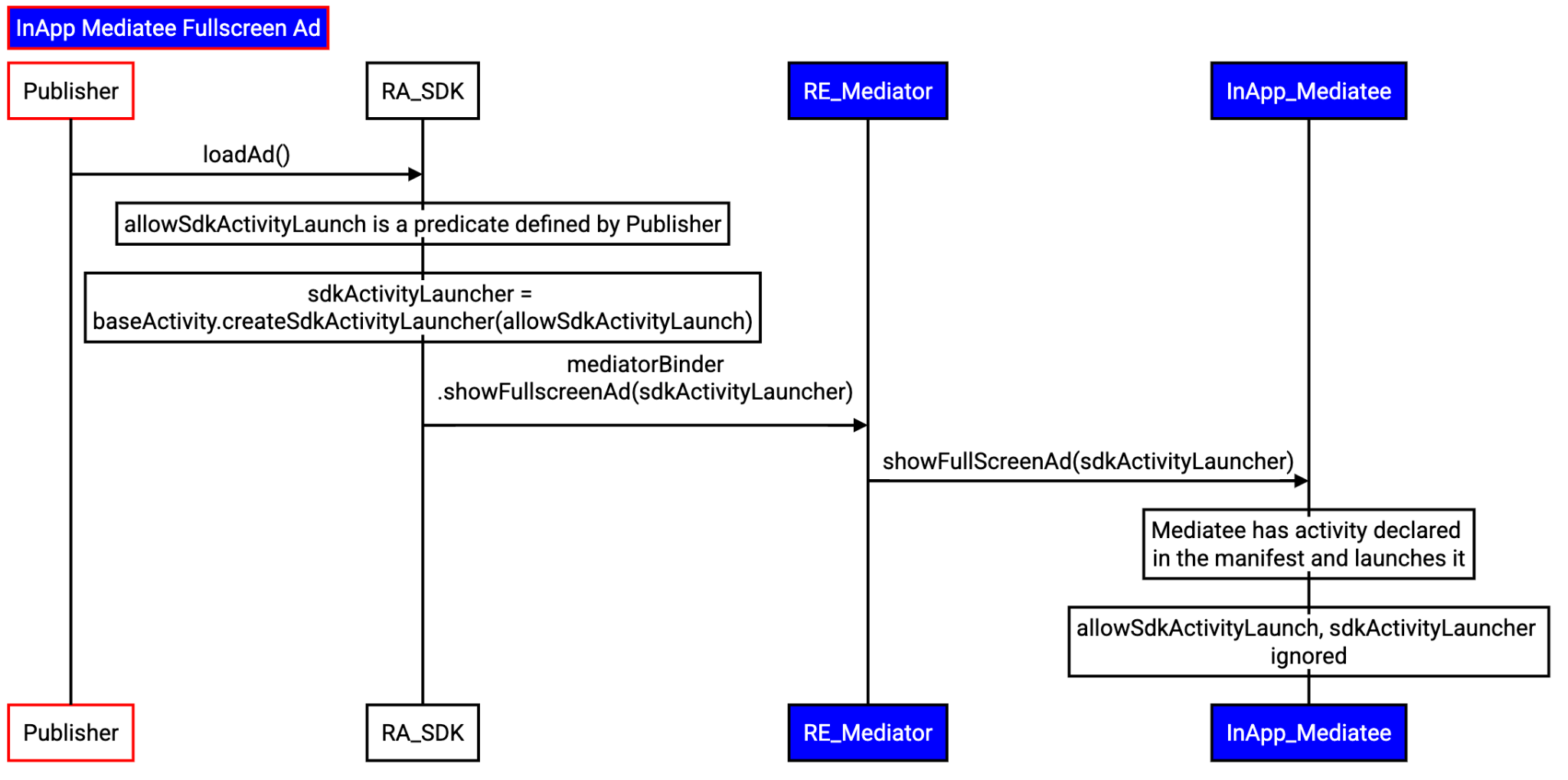
Engage and share feedback
The Privacy Sandbox on Android is an ongoing project, and this document reflects its current design. Your feedback is essential as we continue to develop and enhance its features. File a bug to provide feedback.