Learn how reporting IDs work in a Protected Audience auction
Overview
Reporting IDs are identifiers associated with an ad that are available for usage in generating a bid, scoring a bid, and reporting. The reporting IDs are provided by the buyer in the interest group config, and they become available in generateBid()
, scoreAd()
, reportResult()
and reportWin()
under various conditions which are discussed in this guide.
Reporting IDs allow you to report an identifier for an ad, and also enable use cases such as deals.
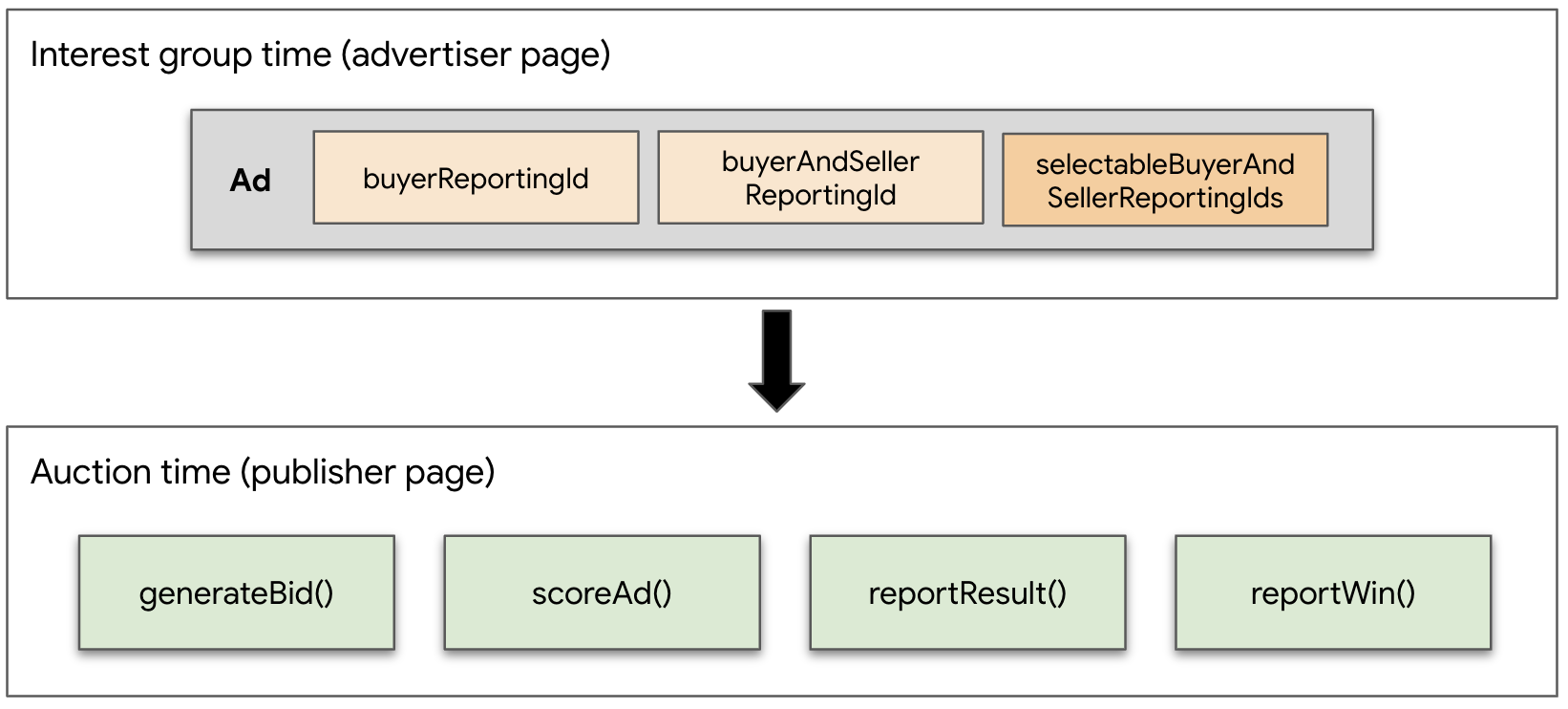
There are three reporting IDs of two types:
- Non-selectable reporting IDs
buyerReportingId
(a string)buyerAndSellerReportingId
(a string)
- Selectable reporting IDs
selectableBuyerAndSellerReportingIds
(an array of strings)
The reporting IDs behave differently depending on if selectable reporting IDs are used. When only non-selectable reporting IDs are used, those IDs become available inside the reporting functions only. When selectable reporting IDs are used, along with non-selectable reporting IDs if needed, then all IDs defined become available inside generateBid()
and scoreAd()
also.
Non-selectable reporting IDs
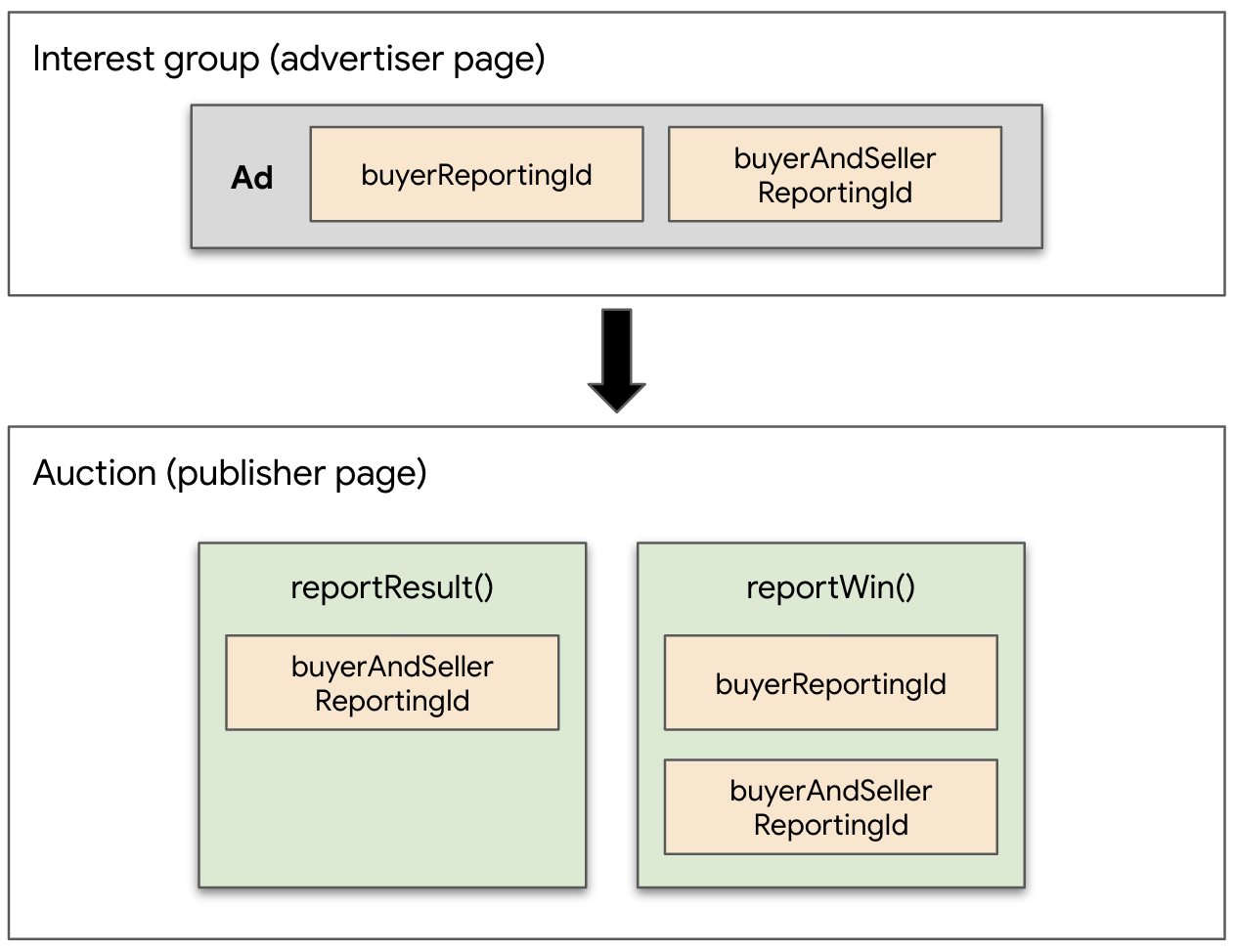
buyerReportingId
and buyerAndSellerReportingId
are non-selectable reporting IDs defined in the interest group config that become available in buyer and seller reporting functions. The buyer and seller reporting functions will only run for the winning ad, and the functions will receive the reporting IDs defined for that winning ad.
When used without selectable reporting IDs, the buyer reporting function receives buyerReportingId
or buyerAndSellerReportingId
depending on the overwriting behavior, and the seller reporting function receives buyerAndSellerReportingId
. If neither buyerReportingId
nor buyerAndSellerReportingId
are defined in the interest group config, then the reportWin()
function receives the interest group name (interestGroupName
) of the winning bid.
Non-selectable IDs are not available inside generateBid()
and scoreAd()
if they are not used in conjunction with selectable reporting IDs.
Reporting IDs in interest groups
The reporting IDs are defined by the buyer for each ad in an interest group:
navigator.joinAdInterestGroup({
owner: 'https://buyer.example',
name: 'example-interest-group',
ads: [{
renderUrl: `https://buyer.example/ad.html`,
// buyerAndSellerReportingId goes to the buyer and seller reporting functions
buyerAndSellerReportingId: 'bsrid123',
// buyerReportingId is defined here as an example, but
// is not used due to the overwrite rules described later
buyerReportingId: 'brid123',
}]
});
Seller reporting
During the seller reporting phase, the buyerAndSellerReportingId
value becomes available to reportResult()
:
function reportResult(..., browserSignals, ...) {
const {
buyerAndSellerReportingId // 'bsrid123'
} = browserSignals;
sendReportTo(`https://seller.example/report?bsrid=${buyerAndSellerReportingId}`);
}
Before the ID becomes available inside reportResult()
, it is checked for k-anonymity with the interest group owner, bidding script URL, render URL, and ad size (ad size is excluded from this check until at least Q1 2025). If it is not k-anonymous, the reportResult()
function will still run, but the reporting ID value won't be available inside the function.
Buyer reporting
During the buyer reporting phase of the auction, one reporting ID becomes available to reportWin()
. If more than one reporting ID is defined in the interest group, then an overwrite rule is applied where buyerAndSellerReportingId
overwrites buyerReportingId
:
- If
buyerAndSellerReportingId
andbuyerReportingId
are both defined, thenbuyerAndSellerReportingId
will overwritebuyerReportingId
, andbuyerAndSellerReportingId
will be available insidereportWin()
. - If only
buyerReportingId
is defined, thenbuyerReportingId
will be available. - If neither
buyerAndSellerReportingId
norbuyerReportingId
are defined, then theinterestGroupName
will be available.
function reportWin(..., browserSignals, ...) {
const {
buyerAndSellerReportingId // 'bsrid123'
} = browserSignals;
sendReportTo(`https://seller.example/report?bsrid=${buyerAndSellerReportingId}`);
}
The reporting ID that becomes available inside reportWin()
is checked for k-anonymity with the interest group owner, bidding script URL, render URL, and ad size (ad size is excluded from this check until at least Q1 2025). If it fails the k-anonymity check, then reportWin()
will still run, but the reporting ID value won't be available inside the function.
Only buyerReportingId
is defined
If only buyerReportingId
is defined in the interest group config:
navigator.joinAdInterestGroup({
owner: 'https://buyer.example',
name: 'example-interest-group',
ads: [{
renderUrl: `https://buyer.example/ad.html`,
buyerReportingId: 'brid123',
}]
});
Then buyerReportingId
is available inside reportWin()
:
function reportWin(..., browserSignals, ...) {
const {
buyerReportingId, // 'brid123'
} = browserSignals;
}
Before becoming available to reportWin()
, buyerReportingId
is checked for k-anonymity with the interest group owner, bidding script URL, render URL, and ad size (ad size is excluded from this check until at least Q1 2025).
Only buyerAndSellerReportingId is defined
If only buyerAndSellerReportingId
is defined in the interest group config:
navigator.joinAdInterestGroup({
owner: 'https://buyer.example',
name: 'example-interest-group',
ads: [{
renderUrl: `https://buyer.example/ad.html`,
buyerAndSellerReportingId: 'bsrid123',
}]
});
Then buyerAndSellerReportingId
is available inside reportWin()
:
function reportWin(..., browserSignals, ...) {
const {
buyerAndSellerReportingId, // 'bsrid123'
} = browserSignals;
}
Before becoming available to reportWin()
, buyerAndSellerReportingId
is checked for k-anonymity with the interest group owner, bidding script URL, render URL, and ad size (ad size is excluded from this check until at least Q1 2025).
Both buyerAndSellerReportingId
and buyerReportingId
are defined
If both buyerAndSellerReportingId
and buyerReportingId
are defined in the interest group config:
navigator.joinAdInterestGroup({
owner: 'https://buyer.example',
name: 'example-interest-group',
ads: [{
renderUrl: `https://buyer.example/ad.html`,
buyerReportingId: 'brid123',
buyerAndSellerReportingId: 'bsrid123',
}]
});
Then only buyerAndSellerReportingId
is available inside reportWin()
due to the overwrite behavior:
function reportWin(..., browserSignals, ...) {
const {
buyerAndSellerReportingId, // 'bsrid123'
} = browserSignals;
}
Before becoming available to reportWin()
, buyerAndSellerReportingId
is checked for k-anonymity with the interest group owner, bidding script URL, render URL, and ad size (ad size is excluded from this check until at least Q1 2025).
Neither buyerAndSellerReportingId
nor buyerReportingId
are defined
If neither reporting IDs are defined in the interest group config:
navigator.joinAdInterestGroup({
owner: 'https://buyer.example',
name: 'example-interest-group',
ads: [{
renderUrl: `https://buyer.example/ad.html`,
}]
});
Then the interest group name
is available inside reportWin()
:
function reportWin(..., browserSignals, ...) {
const {
interestGroupName, // 'example-interest-group'
} = browserSignals;
}
Before becoming available to reportWin()
, the interest group name (interestGroupName
) is checked for k-anonymity with the interest group owner, bidding script URL, render URL, and ad size (ad size is excluded from this check until at least Q1 2025).
Selectable reporting IDs
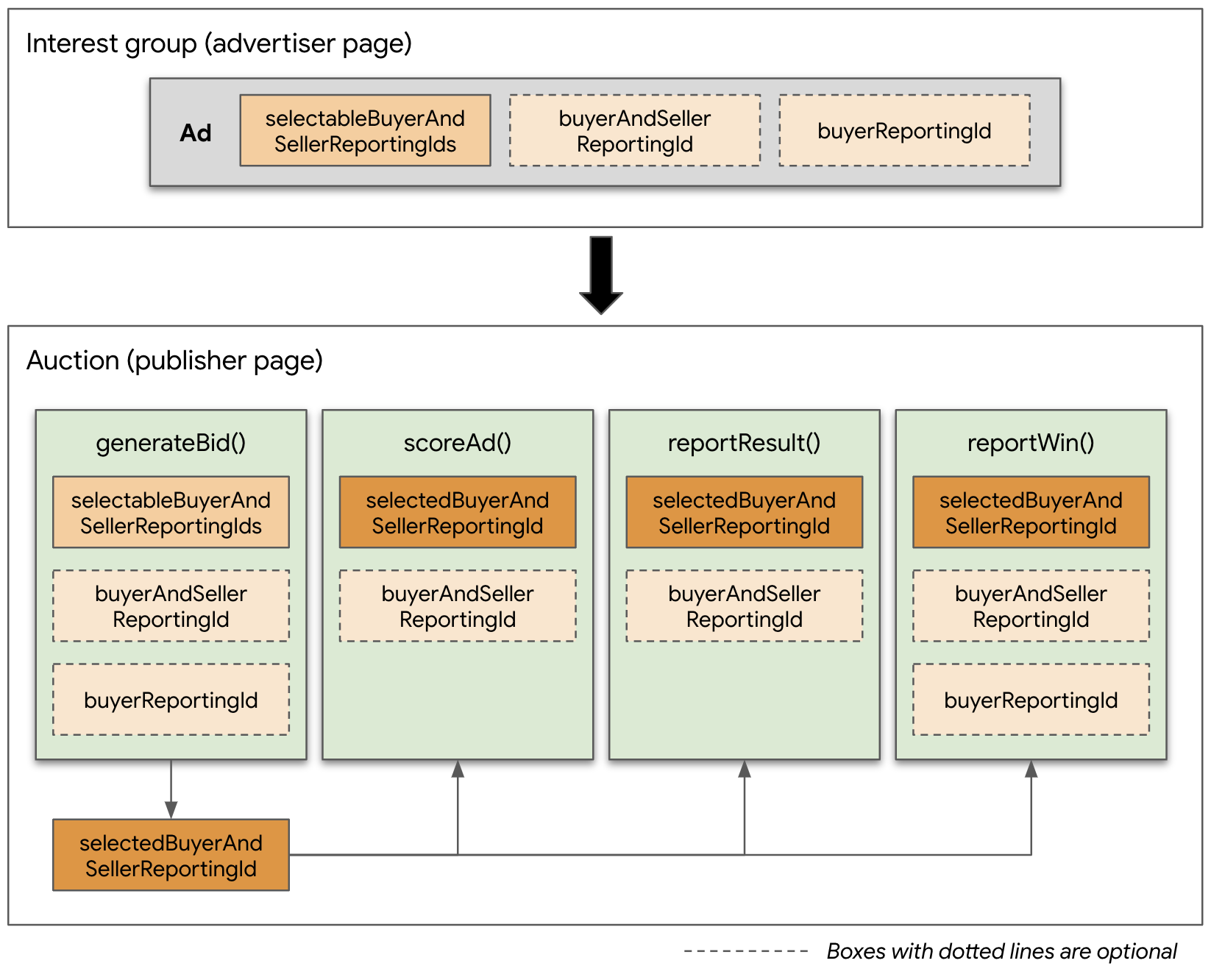
Selectable reporting IDs allow a buyer to select an ID during bid generation, and the browser makes the selected value available to scoreAd()
and reporting functions. The selectableBuyerAndSellerReportingIds
value, which is an array of strings, is provided to generateBid()
, and the buyer is able to return one selected ID as selectedBuyerAndSellerReportingId
.
The generateBid()
and scoreAd()
functions will run for each ad defined in the interest group config and receive the reporting IDs for each ad. The buyer and seller reporting functions will only run for the winning ad, and the functions will receive the reporting IDs defined for that winning ad.
When non-selectable reporting IDs are used in conjunction with selectable reporting IDs, their behavior changes from the workflow described in the previous section. Unlike the initial behavior of non-selectable reporting IDs being only available inside reporting functions, selectable reporting IDs enable non-selectable reporting IDs to become available inside generateBid()
and scoreAd()
also.
Interest group
The selectable reporting IDs field - selectableBuyerAndSellerReportingIds
- is an array of strings defined by the buyer in the interest group for an ad. Non-selectable reporting IDs can also be defined alongside selectable reporting IDs:
navigator.joinAdInterestGroup({
owner: 'https://buyer.example',
name: 'example-interest-group',
ads: [{
renderUrl: `https://buyer.example/ad.html`,
buyerReportingId: 'brid123',
buyerAndSellerReportingId: 'bsrid123',
selectableBuyerAndSellerReportingIds: ['sbsrid1', 'sbsrid2', 'sbsrid3']
}]
});
Buyer bid generation
If selectableBuyerAndSellerReportingIds
was defined in the interest group config, then it becomes available inside generateBid()
along with other reporting IDs that were defined.
function generateBid(interestGroup, ...) {
const [{
buyerReportingId, // 'brid123'
buyerAndSellerReportingId, // 'bsrid123'
selectableBuyerAndSellerReportingIds // ['sbsrid1', 'sbsrid2', 'sbsrid3']
}] = interestGroup.ads;
return {
bid: 1,
render: 'https://buyer.example/ad.html',
selectedBuyerAndSellerReportingId: 'sbsrid2' // Buyer returns the selected ID
};
}
The buyer can choose one of the IDs from the selectableBuyerAndSellerReportingIds
array in generateBid()
and return the selected ID as selectedBuyerAndSellerReportingId
. The bid is rejected if the selected value is not in the selectableBuyerAndSellerReportingIds
array. If selectableBuyerAndSellerReportingIds
is defined in the interest group config, and the buyer does not return selectedBuyerAndSellerReportingId
from generateBid()
, then the reporting IDs will revert to the behavior described for non-selectable reporting IDs.
A bid with a returned value for selectedbuyerAndSellerReportingId
may only win the auction if the value of selectedbuyerAndSellerReportingId
is jointly k-anonymous along with buyerAndSellerReportingId
(if present), buyerReportingId
(if present), the interest group owner, bidding script URL, render URL, and ad size (ad size is excluded from this check until at least Q1 2025).
Seller ad scoring
For the seller, the selectedBuyerAndSellerReportingId
that was returned by the buyer from generateBid()
becomes available in scoreAd()
, along with buyerAndSellerReportingId
if it was defined in the interest group config.
function scoreAd(..., browserSignals, ...) {
const {
buyerAndSellerReportingId, // 'bsrid123'
selectedBuyerAndSellerReportingId, // 'sbsrid2'
} = browserSignals;
// ...
}
Seller reporting
For seller reporting, the selectedBuyerAndSellerReportingId
that was returned by the buyer from generateBid()
becomes available in reportResult()
, along with buyerAndSellerReportingId
, if it was defined in the interest group.
function reportResult(..., browserSignals, ...) {
const {
buyerAndSellerReportingId, // 'bsrid123'
selectedBuyerAndSellerReportingId // 'sbsrid2'
} = browserSignals;
// ...
}
If selectableBuyerAndSellerReportingIds
was defined in the interest group config, and selectedBuyerAndSellerReportingId
was returned from generateBid()
, then it cannot win the auction unless selectedBuyerAndSellerReportingId
and buyerAndSellerReportingId
(if present) are k-anonymous with the interest group owner, bidding script URL, render URL, and ad size (ad size is excluded from this check until at least Q1 2025), and reportResult()
won't be executed for that bid. Therefore, if reportResult()
is called with a value for selectedBuyerAndSellerReportingId
, it means the reporting IDs passed the k-anonymity check, and all of the reporting IDs defined will be available inside reportResult()
.
Buyer reporting
If selectableBuyerAndSellerReportingIds
was defined in the interest group config, and selectedBuyerAndSellerReportingId
was returned from generateBid()
, then all reporting IDs that were defined in the interest group config become available. Note that similar to seller reporting, if the reporting IDs are not k-anonymous, then they cannot win the auction and reportWin()
won't run for that bid.
function reportWin(..., browserSignals, ...) {
const {
buyerReportingId, // 'brid123'
buyerAndSellerReportingId, // 'bsrid123'
selectedBuyerAndSellerReportingId // 'sbsrid2'
} = browserSignals;
// ...
}
Overwrite rules
Here we summarize the overwrite rules for both non-selectable reporting IDs and selectable reporting IDs. Which of selectableBuyerAndSellerReportingIds
, buyerAndSellerReportingId
, buyerReportingId
, and the interest group name gets passed to reportWin()
is determined by the browser with the following logic:
- If
selectedBuyerAndSellerReportingId
is returned from a bid, thenselectedBuyerAndSellerReportingId
,buyerAndSellerReportingId
(if defined in interest group), andbuyerReportingId
(if defined in the interest group) are all available for reporting. - Otherwise, if
buyerAndSellerReportingId
is defined in the interest group, then onlybuyerAndSellerReportingId
is available for reporting. - Otherwise, if
buyerReportingId
is defined in the interest group, then onlybuyerReportingId
is available for reporting. - Otherwise, only the interest group
name
is available for reporting.
The following table describes the overwrite behavior:
Are the reporting IDs defined in the interest group config? | Reporting IDs available | |||
selectableBuyerAnd
|
buyerAndSeller
|
buyerReportingId
|
reportWin()
|
reportResult()
|
Yes, and selected in generateBid()
|
Optional | Optional |
1) selectableBuyerAnd 2) buyerAndSeller (if defined)3) buyerReportingId (if defined)
|
1) selectableBuyerAnd 2) buyerAndSeller (if defined) |
No, or not selected in generateBid() |
Yes | Ignored | buyerAndSeller |
buyerAndSeller |
No, or not selected in generateBid() |
No | Yes | buyerReportingId |
None |
No, or not selected in generateBid() |
No | No | interestGroupName |
None |
Engage and share feedback
- To learn more about reporting IDs, see the Reporting ID section of the Protected Audience explainer.
- GitHub: Raise questions and follow discussion in issues on the API repository.
- W3C: Discuss industry use cases in the WICG call.
- Announcements: Join or view the mailing list.
- Privacy Sandbox developer support: Ask questions and join discussions on the Privacy Sandbox Developer Support repository.
- Chromium: File a Chromium bug to ask questions about the implementation available to test in Chrome.