如需在 Google Cloud Search 中实现访问权限控制,您需要用到用户的 Google 账号。将内容编入索引时,必须将各项内容中的所有 ACL 解析为有效的 Google 用户或群组 ID(电子邮件地址)。
在许多情况下,代码库中不会直接存储 Google 账号的信息。相反,用户可以由本地账号表示,或使用联合登录与身份提供商和 ID(用户的电子邮件地址除外)来识别每个账号。此 ID 称为外部 ID。
如果使用管理控制台创建身份源,可通过以下方式弥合多个身份系统之间的上述差异:
- 定义一个自定义用户字段以存储外部 ID。该字段的作用是解析 Google 账号的外部 ID。
- 为代码库或身份提供商托管的安全群组定义一个命名空间。
出现以下情况时,请使用身份源:
- 代码库未存储 Google Workspace 或 Google Cloud Directory 中用户的主电子邮件地址。
- 代码库定义的访问控制群组与 Google Workspace 中基于电子邮件创建的群组不对应。
身份源的优点是可通过将索引与身份映射分离来提高索引效率。利用这种分离,您就可以在创建 ACL 和将各项内容编入索引时推迟查询用户。
部署示例
下图 1 的示例说明了如何部署由企业使用的本地代码库和云端代码库。这两种代码库都使用不同类型的外部 ID 来引用用户。
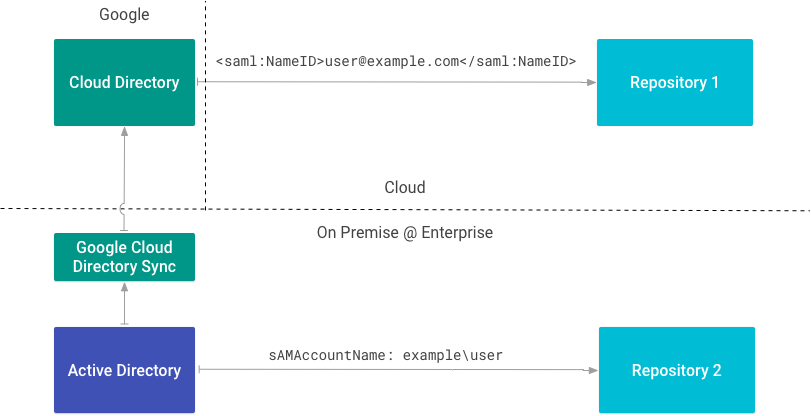
代码库 1 使用由 SAML 声明的电子邮件地址来标识用户。由于该代码库存储了 Google Workspace 或 Cloud Directory 中用户的主电子邮件地址,因此不需要身份源。
代码库 2 直接与本地目录集成,并通过用户的 sAMAccountName
属性标识用户。由于代码库 2 使用 sAMAccountName
属性作为外部 ID,因此需要身份源。
创建身份源
如果您需要用到身份源,请参阅在 Cloud Search 中映射用户身份。
在创建内容连接器之前,您必须先创建身份源,因为您需要身份源 ID 来创建 ACL 和将数据编入索引。如前所述,创建身份源时还会在 Cloud Directory 中创建自定义用户属性。利用此属性即可记录代码库中每个用户的外部 ID。该属性的命名方式遵循以下规范 IDENTITY_SOURCE_ID_identity
。
下表显示了两个身份源,一个用于将 SAM 账号名称 (sAMAccountName) 保存为外部 ID,另一个用于将用户 ID (uid) 保存为外部 ID。
身份源 | 用户属性 | 外部 ID |
---|---|---|
id1 | id1_identity | sAMAccountName |
id2 | id2_identity | uid |
请为用于引用企业中的用户的每个潜在外部 ID 创建一个身份源。
下表显示了具有 Google 账号和两个外部 ID(id1_identity 和 id2_identity)的用户及其值在 Cloud Directory 中的显示方式:
用户 | 电子邮件地址 | id1_identity | id2_identity |
---|---|---|---|
Ann | ann@example.com | example\ann | 1001 |
在形成用于索引的 ACL 时,您可以使用三个不同的 ID(Google 电子邮件、sAMAccountName 和 uid)引用同一用户。
编写用户 ACL
使用 getUserPrincpal() 方法或 getGroupPrincipal() 方法和预配的外部 ID 来创建主账号。
以下示例演示了如何检索文件权限。这些权限包括有权访问该文件的每个用户的名称。
以下代码段显示了如何使用存储在属性中的外部 ID (externalUserName
) 创建所有者的主账号。
最后,以下代码段显示了如何创建有权读取该文件的用户的主账号。
掌握所有读取者和所有者的信息后,您便可以创建 ACL:
创建主账号时,底层 REST API 的 ID 使用以下格式:identitysources/IDENTITY_SOURCE_ID/users/EXTERNAL_ID
再次参考前面的表格,如果使用 Ann 的 id1_identity
(SAMAccountName) 创建 ACL,则 ID 将被解析为:
identitysources/id1_identity/users/example/ann
整个 ID 被称为用户的中间 ID,因为它搭建了一个桥梁来联接外部 ID 和存储在 Cloud Directory 中的 Google ID。
如需了解对用于代码库的 ACL 进行建模的更多信息,请参阅 ACL。
映射群组
除上述作用外,身份源还可用作 ACL 中使用的群组的命名空间。您可以使用此命名空间功能来创建和映射仅用于安全目的或存储在代码库的群组。
首先使用 Cloud Identity Groups API 创建群组并加入成员。要将群组与身份源关联,请使用身份源的资源名称作为群组的命名空间。
以下代码段显示了如何使用 Cloud Identity Groups API 创建群组:
创建群组 ACL
如需创建群组 ACL,请使用 getGroupPrincipal() 方法和提供的外部 ID 创建群组的主账号。然后,使用 Acl.Builder 类构建 ACL,具体如下所示:
身份连接器
虽然您可以使用外部非 Google ID 来创建 ACL 和将各项内容编入索引,但在其外部 ID 解析为 Cloud Directory 中的 Google ID 之前,用户无法查看搜索内容。为此,有三种方法可以确保 Cloud Directory 掌握用户的 Google ID 和外部 ID 信息:
- 通过管理控制台手动更新每个用户的配置文件。仅在使用一些用户配置文件来进行测试和原型设计时才建议使用此流程。
- 使用 Directory API 将外部 ID 映射到 Google ID。对于无法使用身份连接器 SDK 的用户,建议使用此流程。
- 使用身份连接器 SDK 创建身份连接器。此 SDK 简化了使用 Directory API 映射 ID 的流程。
身份连接器是用于将外部 ID 从企业身份(用户和群组)映射到 Google Cloud Search 使用的内部 Google 身份的程序。如果必须创建身份源,则必须先创建身份连接器。
举例来说,Google Cloud Directory Sync (GCDS) 就是一个身份连接器。此身份连接器将用户和群组信息从 Microsoft 的活动目录映射到 Cloud Directory 以及可能表示用户在其他系统中的身份的用户属性。
使用 REST API 同步身份
使用 update
方法和 REST API 同步身份。
重新映射身份
将项的 ID 重新映射到其他 ID 后,您必须为项重新编制索引,以便新 ID 生效。例如,
- 如果您尝试移除用户的映射或将其重新映射到其他用户,系统仍会保留原始映射,直到您重新编制索引。
- 如果您删除了项 ACL 中存在的映射群组,然后创建了具有相同
groupKey
的新群组,那么在重新编制索引之前,新群组不会提供对相应项的访问权限。