Easily access Google APIs from Java
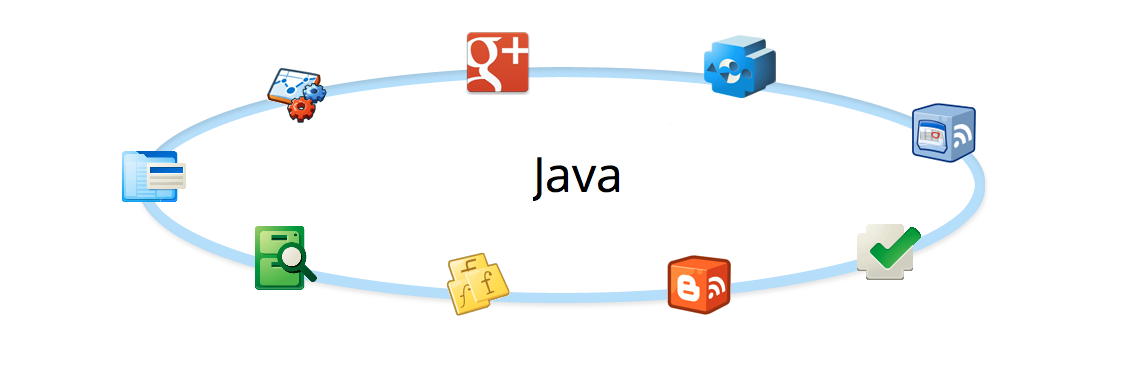
The Google API Client Library for Java provides functionality common to all Google APIs, for example HTTP transport, error handling, authentication, JSON parsing, media download/upload, and batching. The library includes a powerful OAuth 2.0 library with a consistent interface; lightweight, efficient XML and JSON data models that support any data schema; and support for protocol buffers.
To call a Google API using Google's client libraries for Java, you need the generated Java library for the Google API you are accessing. These generated libraries include the core google-api-java-client library along with API-specific information such as the root URL. They also include classes that represent entities in the context of the API, and that are useful for making conversions between JSON objects and Java objects.
To call a Google API using Google's client libraries for Java, you need the generated Java library for the Google API you are accessing. These generated libraries include the core google-api-java-client library along with API-specific information such as the root URL. They also include classes that represent entities in the context of the API, and that are useful for making conversions between JSON objects and Java objects.
Deprecated features
Deprecated non-beta features will be removed eighteen months after the release in which they are first deprecated. You must fix your usages before this time. If you don't, any type of breakage might result, and you are not guaranteed a compilation error.
Highlights of the Google API Client Library for Java
It's simple to call Google APIs
You can call Google APIs using Google service-specific generated libraries with the Google API Client Library for Java. (To find the generated client library for a Google API, visit the list of supported Google APIs.) Here's an example that uses the Calendar API Client Library for Java to make a call to the Google Calendar API:
// Show events on user's calendar. View.header("Show Calendars"); CalendarList feed = client.calendarList().list().execute(); View.display(feed);
The library makes batching and media upload/download easier
The library offers helper classes for batching, media upload, and media download.The library makes auth easier
The library includes a powerful authentication library that can reduce the amount of code you need to handle OAuth 2.0. Sometimes a few lines is all you need. For example:
/** Authorizes the installed application to access user's protected data. */ private static Credential authorize() throws Exception { // load client secrets GoogleClientSecrets clientSecrets = GoogleClientSecrets.load(JSON_FACTORY, new InputStreamReader(CalendarSample.class.getResourceAsStream("/client_secrets.json"))); // set up authorization code flow GoogleAuthorizationCodeFlow flow = new GoogleAuthorizationCodeFlow.Builder( httpTransport, JSON_FACTORY, clientSecrets, Collections.singleton(CalendarScopes.CALENDAR)).setDataStoreFactory(dataStoreFactory) .build(); // authorize return new AuthorizationCodeInstalledApp(flow, new LocalServerReceiver()).authorize("user"); }
The library runs on Google App Engine
App Engine-specific helpers make quick work of authenticated calls to APIs, and you do not need to worry about exchanging code for tokens.
For example:
For example:
@Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws IOException { AppIdentityCredential credential = new AppIdentityCredential(Arrays.asList(UrlshortenerScopes.URLSHORTENER)); Urlshortener shortener = new Urlshortener.Builder(new UrlFetchTransport(), new JacksonFactory(), credential) .build(); UrlHistory history = shortener.URL().list().execute(); ... }
The library runs on Android 4.4 or higher.
The Google Client Library for Java's Android-specific helper classes are well-integrated with Android AccountManager. For example:
@Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // Google Accounts credential = GoogleAccountCredential.usingOAuth2(this, Collections.singleton(TasksScopes.TASKS)); SharedPreferences settings = getPreferences(Context.MODE_PRIVATE); credential.setSelectedAccountName(settings.getString(PREF_ACCOUNT_NAME, null)); // Tasks client service = new com.google.api.services.tasks.Tasks.Builder(httpTransport, jsonFactory, credential) .setApplicationName("Google-TasksAndroidSample/1.0").build(); }
Installation is easy
If you are not using a generated library, you can download the binary for the Google API Client Library for Java directly from the downloads page, or you can use Maven or Gradle. To use Maven, add the following lines to your pom.xml file:
To use Gradle, add the following lines to your build.gradle file:
<project> <dependencies> <dependency> <groupId>com.google.api-client</groupId> <artifactId>google-api-client</artifactId> <version>1.32.1</version> </dependency> </dependencies> </project>
To use Gradle, add the following lines to your build.gradle file:
repositories { mavenCentral() } dependencies { compile 'com.google.api-client:google-api-client:1.32.1' }For more details about installing and setting up the Google API Client Library for Java, see the download and setup instructions.
Supported environments
The Google API Client Library for Java supports these Java environments:
- Java 7 or higher, standard (SE) and enterprise (EE).
- Google App Engine.
- Android 4.4 or higher — but if a Google Play Services library is available for the Google service you need, use that library instead of this one. The Google Play library will give you the best possible performance and experience.