The Phone Number Hint API, a library powered by Google Play services, provides a frictionless way to show a user’s (SIM-based) phone numbers as a hint.
The benefits to using Phone Number Hint include the following:
- No additional permission requests are needed
- Eliminates the need for the user to manually type in the phone number
- No Google account is needed
- Not directly tied to sign in/up workflows
- Wider support for Android versions compared to Autofill
Before you begin
To prepare your app, complete the steps in the following sections.
Configure your app
Add the Google Play services
dependency for the Phone Number Hint API to your
module's Gradle build file,
which is commonly app/build.gradle
:
apply plugin: 'com.android.application'
...
dependencies {
implementation 'com.google.android.gms:play-services-auth:21.3.0'
}
How it works
The Phone Number Hint API utilizes a PendingIntent
to initiate the flow. Once the PendingIntent has been launched the user will be
presented with a UI, listing out all (SIM-based) phone numbers. The user can
then choose to select a phone number they would like to use or cancel the flow.
The selected phone number will then be made available to the developer to
retrieve from the Intent
.
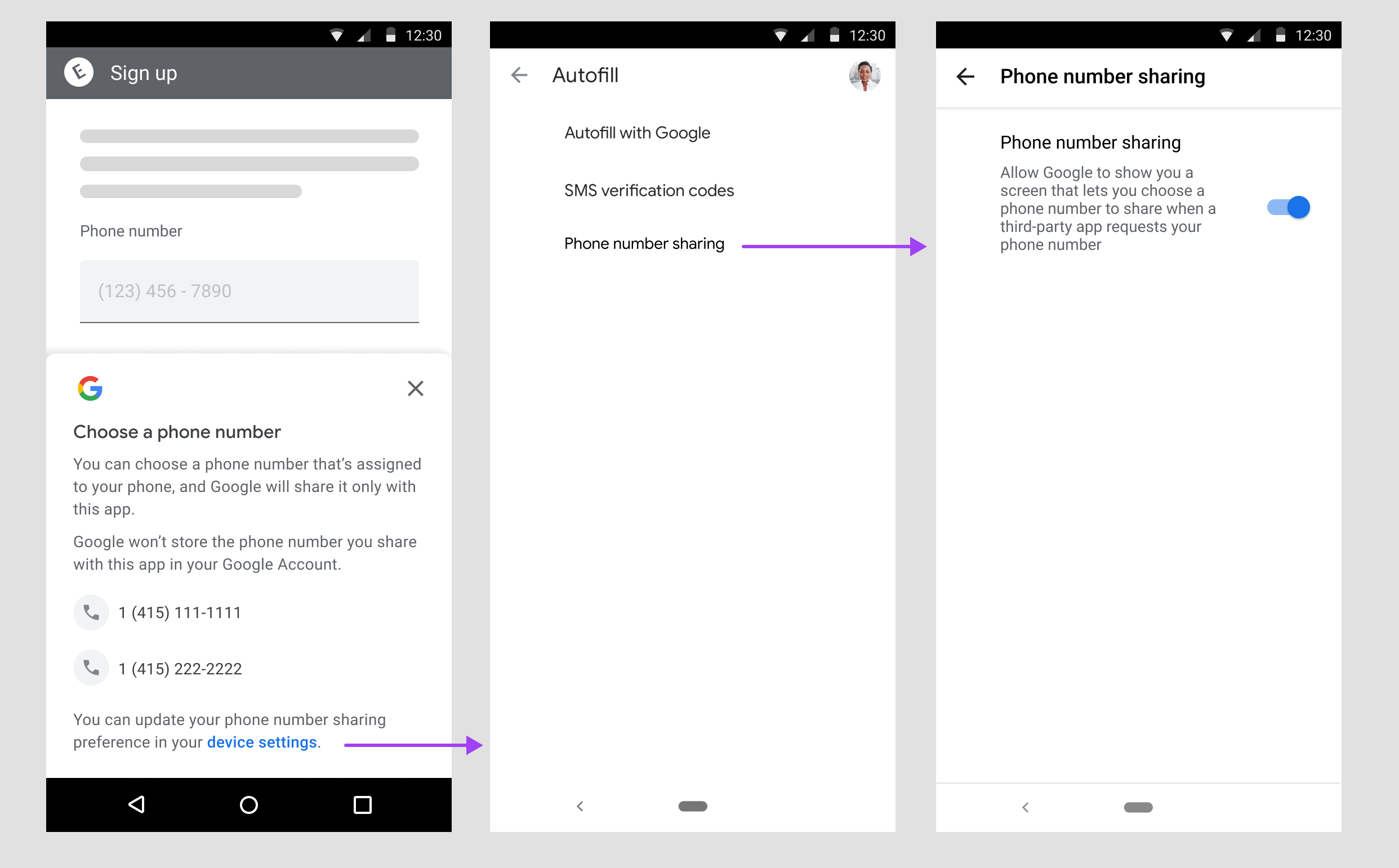
Create a GetPhoneNumbeHintIntentRequest object
Start by creating a GetPhoneNumberHintIntentRequest
object using the
provided GetPhoneNumberHintIntentRequest.Builder()
method. This request object can then be used to get an Intent
to start the
Phone Number Hint flow.
Kotlin
val request: GetPhoneNumberHintIntentRequest = GetPhoneNumberHintIntentRequest.builder().build()
Java
GetPhoneNumberHintIntentRequest request = GetPhoneNumberHintIntentRequest.builder().build();
Requesting Phone Number Hint
Call SignInClient.getPhoneNumberHintIntent()
,
passing in the previous GetPhoneNumberHintIntentRequest
object,
to retrieve the PendingIntent
to initiate the Phone Number Hint flow.
Kotlin
val phoneNumberHintIntentResultLauncher = ... Identity.getSignInClient(activity) .getPhoneNumberHintIntent(request) .addOnSuccessListener { result: PendingIntent -> try { phoneNumberHintIntentResultLauncher.launch( IntentSenderRequest.Builder(result).build() ) } catch (e: Exception) { Log.e(TAG, "Launching the PendingIntent failed") } } .addOnFailureListener { Log.e(TAG, "Phone Number Hint failed") }
Java
ActivityResultLauncherphoneNumberHintIntentResultLauncher = ... Identity.getSignInClient(activity) .getPhoneNumberHintIntent(request) .addOnSuccessListener( result -> { try { phoneNumberHintIntentResultLauncher.launch(result.getIntentSender()); } catch(Exception e) { Log.e(TAG, "Launching the PendingIntent failed", e); } }) .addOnFailureListener(e -> { Log.e(TAG, "Phone Number Hint failed", e); });
Retrieving the Phone Number
Pass in the Intent
to SignInClient.getPhoneNumberFromIntent
to retrieve the phone number.
Kotlin
val phoneNumberHintIntentResultLauncher = registerForActivityResult(ActivityResultContracts.StartIntentSenderForResult()) { result -> try { val phoneNumber = Identity.getSignInClient(activity).getPhoneNumberFromIntent(result.data) } catch(e: Exception) { Log.e(TAG, "Phone Number Hint failed") } }
Java
ActivityResultLauncherphoneNumberHintIntentResultLauncher = registerForActivityResult( new ActivityResultContracts.StartActivityForResult(), new ActivityResultCallback () { @Override public void onActivityResult(ActivityResult result) { try { String phoneNumber = Identity.getSignInClient(activity).getPhoneNumberFromIntent(result.getData()); } catch { Log.e(TAG, "Phone Number Hint failed", e); } } });