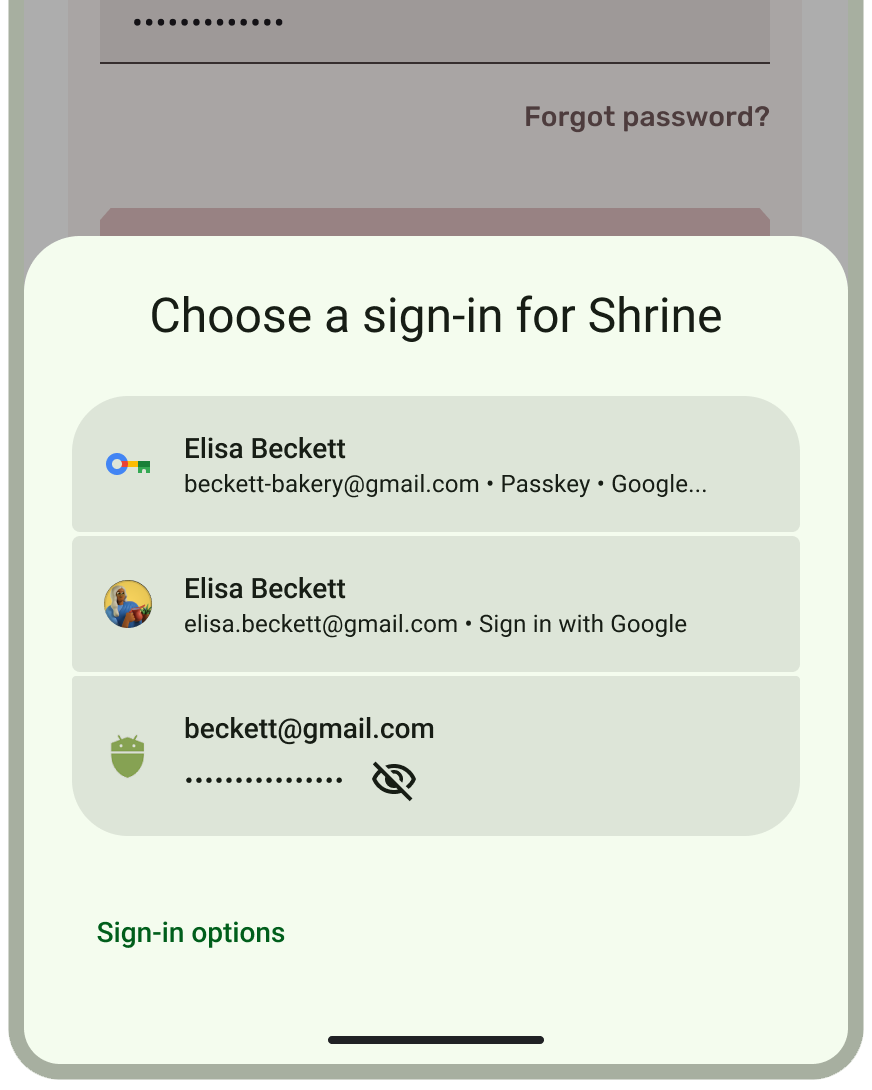
Boost your app's user experience by seamlessly integrating passwordless authentication with passkeys using Credential Manager. Credential Manager is an Android Jetpack library that unifies API support for most major authentication methods, including passkeys, passwords, and federated sign-in solutions (such as Sign-in with Google).
Credential Manager automatically displays a unified bottom-sheet for modern authentication methods, and is the modern replacement for existing authentication implementations, including Smart Lock for Passwords on Android, and One Tap. Credential Manager's unified sign-in interface gives your users a clear, familiar, and consistent experience, reduces churn and improves registration and sign-in speeds.
Learn more about how to integrate Credential Manager with your Android app:
- Sign in your user with Credential Manager developer's guide
- User authentication with passkeys UX guide
- Integrate Credential Manager with Sign in with Google
- Upgrade from legacy Sign in with Google button flows
- Integrate Credential Manager with WebView
- Migrate from Smart Lock for Passwords to Credential Manager
- Migrate from FIDO2 to Credential Manager
- Migrate from legacy Google Sign-In to Credential Manager
- androidx.credentials API documentation
- Passkeys on Android learning pathway
- Codelab: Learn how to simplify auth journeys using Credential Manager API in your Android app
Learn more about how to streamline your existing identity and authentication APIs to support passkeys and improved usability with the Credential Manager API: