This document provides an overview of how to measure in-app ecommerce related actions and impressions with the Google Analytics SDK for iOS v3.
Overview
Enhanced ecommerce enables the measurement of user interactions with products across the user's shopping experience, including: product impressions, product clicks, viewing product details, adding a product to a shopping cart, initiating the checkout process, transactions, and refunds.
Implementation
Before implementing enhanced ecommerce tracking in your app, you must add the enhanced ecommerce library to your app.
After configuring your application to use enhanced ecommerce, you can:
- Measure Ecommerce Activities
- Measure Transactions
- Measure Refunds
- Measure the Checkout Process
- Measure Internal Promotions
Measuring Ecommerce Activities
A typical enhanced ecommerce implementation will measure product impressions, and any of the following actions:
- Selecting a product.
- Viewing product details.
- Impressions and selection of internal promotions.
- Adding / removing a product from a shopping cart.
- Initiating the checkout process for a product.
- Purchases and refunds.
Measuring Impressions
To measure a product impression, set the product and impression values and send it with a hit:
idtracker = [[GAI sharedInstance] trackerWithTrackingId:@"MyTrackingId"]; GAIEcommerceProduct *product = [[GAIEcommerceProduct alloc] init]; [product setId:@"P12345"]; [product setName:@"Android Warhol T-Shirt"]; [product setCategory:@"Apparel/T-Shirts"]; [product setBrand:@"Google"]; [product setVariant:@"Black"]; [product setCustomDimension:1 value:@"Member"]; GAIDictionaryBuilder *builder = [GAIDictionaryBuilder createScreenView]; // Sets the product impression for the next available slot, starting with 1. [builder addProductImpression:product impressionList:@"Search Results" impressionSource:@"From Search"]; [tracker set:kGAIScreenName value:@"My Impression Screen"]; [tracker send:[builder build]];
A product must have a name or id value. All other values are optional and don't need to be set.
Measuring Actions
Actions are measured by setting product values and then setting product action values to specify the action being performed.
For example, the following code measures the selection of a product displayed in a list of search results:
id<GAITracker> tracker = [[GAI sharedInstance] trackerWithTrackingId:@"MyTrackingId"]; GAIEcommerceProduct *product = [[GAIEcommerceProduct alloc] init]; [product setId:@"P12345"]; [product setName:@"Android Warhol T-Shirt"]; [product setCategory:@"Apparel/T-Shirts"]; [product setBrand:@"Google"]; [product setVariant:@"Black"]; [product setCustomDimension:1 value:@"Member"]; GAIEcommerceProductAction *action = [[GAIEcommerceProductAction alloc] init]; [action setAction:kGAIPAClick]; [action setProductActionList:@"Search Results"]; GAIDictionaryBuilder *builder = [GAIDictionaryBuilder createScreenView]; [builder setProductAction:action]; // Sets the product for the next available slot, starting with 1 [builder addProduct:product]; [tracker set:kGAIScreenName value:@"My Impression Screen"]; [tracker send:[builder build]];
A product must have a name or id value. All other values are optional and don't need to be set.
Combining Impressions and Actions
In cases where you have both product impressions and an action, it is possible to combine and measure this in a single hit.
The example below shows how to measure a product detail view with a related products section:
// The product from the related products section. idtracker = [[GAI sharedInstance] trackerWithTrackingId:@"MyTrackingId"]; GAIEcommerceProduct *product = [[GAIEcommerceProduct alloc] init]; [product setId:@"P12346"]; [product setName:@"Android Warhol T-Shirt"]; [product setCategory:@"Apparel/T-Shirts"]; [product setBrand:@"Google"]; [product setVariant:@"White"]; GAIDictionaryBuilder *builder = [GAIDictionaryBuilder createScreenView]; // Sets the product impression for the next available slot, starting with 1. [builder addProductImpression:product impressionList:@"Related Products" impressionSource:@"From Related"]; // The product being viewed. product = [[GAIEcommerceProduct alloc] init]; [product setId:@"P12345"]; [product setName:@"Android Warhol T-Shirt"]; [product setCategory:@"Apparel/T-Shirts"]; [product setBrand:@"Google"]; [product setVariant:@"Black"]; GAIEcommerceProductAction *action = [[GAIEcommerceProductAction alloc] init]; [action setAction:kGAIPADetail]; [builder setProductAction:action]; // Sets the product for the next available slot, starting with 1. [builder addProduct:product]; [tracker set:kGAIScreenName value:@"Related Products Screen"]; [tracker send:[builder build]];
Measuring Transactions
Measure a transaction by setting product values and then setting product action values to specify a purchase action. Transaction level details like total revenue, tax, and shipping are set with the product action values.
id<GAITracker> tracker = [[GAI sharedInstance] trackerWithTrackingId:@"MyTrackingId"]; GAIEcommerceProduct *product = [[GAIEcommerceProduct alloc] init]; [product setId:@"P12345"]; [product setName:@"Android Warhol T-Shirt"]; [product setCategory:@"Apparel/T-Shirts"]; [product setBrand:@"Google"]; [product setVariant:@"Black"]; [product setPrice:@29.20]; [product setCouponCode:@"APPARELSALE"]; [product setQuantity:@1]; GAIDictionaryBuilder *builder = [GAIDictionaryBuilder createEventWithCategory:@"Ecommerce" action:@"Purchase" label:nil value:nil]; GAIEcommerceProductAction *action = [[GAIEcommerceProductAction alloc] init]; [action setAction:kGAIPAPurchase]; [action setTransactionId:@"T12345"]; [action setAffiliation:@"Google Store - Online"]; [action setRevenue:@37.39]; [action setTax:@2.85]; [action setShipping:@5.34]; [action setCouponCode:@"SUMMER2013"]; [builder setProductAction:action]; // Sets the product for the next available slot, starting with 1 [builder addProduct:product]; [tracker send:[builder build]];
Specifying Currency
By default, you can configure a common, global, currency for all transactions and items through the Google Analytics management web interface.
The local currency must be specified in the ISO 4217
standard.
Read the Currency Codes
Reference document for a complete list of supported conversion
currencies.
Local currencies are specified by setting the currency code value on the tracker. For example, this tracker will send currency values as Euros:
id<GAITracker> tracker = [[GAI sharedInstance] trackerWithTrackingId:@"MyTracker"]; [tracker set:kGAIScreenName value:@"transaction"]; [tracker set:kGAICurrencyCode value:@"EUR"]; // Set tracker currency to Euros. GAIDictionaryBuilder *builder = [GAIDictionaryBuilder createScreenView]; [tracker send:[builder build]];
Measuring Refunds
To refund an entire transaction, set product action values to specify the transaction ID and a refund action type:
// Refund an entire transaction. id<GAITracker> tracker = [[GAI sharedInstance] trackerWithTrackingId:@"MyTrackingId"]; GAIDictionaryBuilder *builder = [GAIDictionaryBuilder createEventWithCategory:@"Ecommerce" action:@"Refund" label:nil value:nil]; GAIEcommerceProductAction *action = [[GAIEcommerceProductAction alloc] init]; [action setAction:kGAIPARefund]; [action setTransactionId:@"T12345"]; [builder setProductAction:action]; [tracker send:[builder build]];
If a matching transaction is not found, the refund will not be processed.
To measure a partial refund, set product action values to specify the transaction ID, product ID(s), and product quantities to be refunded:
// Refund a single product. id<GAITracker> tracker = [[GAI sharedInstance] trackerWithTrackingId:@"MyTrackingId"]; GAIDictionaryBuilder *builder = [GAIDictionaryBuilder createEventWithCategory:@"Ecommerce" action:@"Refund" label:nil value:nil]; GAIEcommerceProduct *product = [[GAIEcommerceProduct alloc] init]; [product setId:@"P12345"]; // Product ID is required for partial refund. [product setQuantity:@1]; // Quanity is required for partial refund. [builder addProduct:product]; GAIEcommerceProductAction *action = [[GAIEcommerceProductAction alloc] init]; [action setAction:kGAIPARefund]; [action setTransactionId:@"T12345"]; // Transaction ID is required for partial refund. [builder setProductAction:action]; [tracker send:[builder build]];
Using Non-Interaction Events for Refunds
If you need to send refund data using an event and the event is not part of normally measured user behavior (i.e. not user initiated), then it’s recommended that you send a non-interaction event. This will prevent certain metrics from being affected by the event. For example:
// Refund an entire transaction. id<GAITracker> tracker = [[GAI sharedInstance] trackerWithTrackingId:@"MyTrackingId"]; GAIDictionaryBuilder *builder = [GAIDictionaryBuilder createEventWithCategory:@"Ecommerce" action:@"Refund" label:nil value:nil]; [builder set:@"1" forKey:kGAINonInteraction]; GAIEcommerceProductAction *action = [[GAIEcommerceProductAction alloc] init]; [action setAction:kGAIPARefund]; [action setTransactionId:@"T12345"]; [builder setProductAction:action]; [tracker send:[builder build]];
Measuring the Checkout Process
To measure each step in a checkout process:
- Add tracking code to measure each step of the checkout process.
- If applicable, add tracking code to measure checkout options.
- Optionally set user-friendly step names for the checkout funnel report by configuring Ecommerce Settings in the admin section of the web interface.
1. Measuring Checkout Steps
For each step in your checkout process, you’ll need to implement the corresponding tracking code to send data to Google Analytics.
Step
Field
For each checkout step that you measure you should include a
step
value. This value is used to map your checkout actions to the
labels you configured for each step in Ecommerce Settings.
Option
Field
If you have additional information about the given checkout step at the time the step is measured, you can set the checkout option field with a checkout action to capture this information. For example, the default payment type for the user (e.g. 'Visa').
Measuring a Checkout Step
To measure a checkout step, set product values and then set product action values to indicate a checkout action. If applicable you can also set a checkout step and checkout option value with the checkout.
The following example shows how to measure the first step of a checkout process, with a single product, and some additional information about the payment type:
id<GAITracker> tracker = [[GAI sharedInstance] trackerWithTrackingId:@"MyTrackingId"]; GAIEcommerceProduct *product = [[GAIEcommerceProduct alloc] init]; [product setId:@"P12345"]; [product setName:@"Android Warhol T-Shirt"]; [product setCategory:@"Apparel/T-Shirts"]; [product setBrand:@"Google"]; [product setVariant:@"Black"]; [product setPrice:@29.20]; [product setCouponCode:@"APPARELSALE"]; [product setQuantity:@1]; GAIDictionaryBuilder *builder = [GAIDictionaryBuilder createEventWithCategory:@"Ecommerce" action:@"Checkout" label:nil value:nil]; // Add the step number and additional info about the checkout to the action. GAIEcommerceProductAction *action = [[GAIEcommerceProductAction alloc] init]; [action setAction:kGAIPACheckout]; [action setCheckoutStep:@1]; [action setCheckoutOption:@"Visa"]; [builder addProduct:product]; [builder setProductAction:action]; [tracker send:[builder build]];
2. Measuring Checkout Options
Checkout options allow you to measure additional information about the state of the checkout. This is useful in cases where you've measured a checkout step but additional information about the same checkout step is available after a user selected option has been set. For example, the user selects a shipping method.
To measure a checkout option, set product action values to indicate a checkout option and include the step number, and the option description.
You'll likely want to measure this action once the user has performed some action to move on to the next step in the checkout process. For example:
// (On "Next" button click.) id<GAITracker> tracker = [[GAI sharedInstance] trackerWithTrackingId:@"MyTrackingId"]; GAIDictionaryBuilder *builder = [GAIDictionaryBuilder createEventWithCategory:@"Ecommerce" action:@"CheckoutOption" label:nil value:nil]; GAIEcommerceProductAction *action = [[GAIEcommerceProductAction alloc] init]; [action setAction:kGAIPACheckoutOption]; [action setCheckoutStep:@1]; [action setCheckoutOption:@"Fedex"]; [builder setProductAction:action]; [tracker send:[builder build]]; // Advance to next page.
3. Checkout Funnel Configuration
Each step in your checkout process can be given a descriptive name that will be used in reports. To configure these names, visit the Admin section of the Google Analytics Web Interface, select the view (profile) and click on Ecommerce Settings. Follow the Ecommerce set-up instructions to label each checkout step you intend to track.
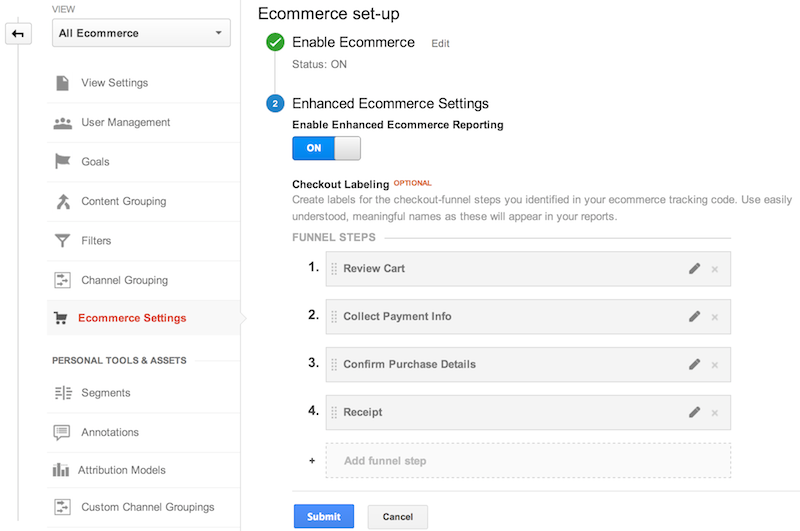
Measuring Internal Promotions
Enhanced ecommerce includes support for measuring impressions and selections of internal promotions, such as banners displayed to promote a sale.
Promotion Impressions
Internal promotion impressions are generally measured with the initial screen view or an event by setting promotion values. For example:
id<GAITracker> tracker = [[GAI sharedInstance] trackerWithTrackingId:@"MyTrackingId"]; GAIEcommercePromotion *promotion = [[GAIEcommercePromotion alloc] init]; [promotion setId:@"PROMO_1234"]; [promotion setName:@"Summer Sale"]; [promotion setCreative:@"summer_banner2"]; [promotion setPosition:@"banner_slot1"]; GAIDictionaryBuilder *builder = [GAIDictionaryBuilder createEventWithCategory:@"Ecommerce" action:@"Promotion" label:nil value:nil]; [builder addPromotion:promotion]; [tracker send:[builder build]];
Promotion Clicks
Clicks on internal promotions can be measured by using promotion values and then setting product action values to indicate a promotion click. For example:
id<GAITracker> tracker = [[GAI sharedInstance] trackerWithTrackingId:@"MyTrackingId"]; GAIEcommercePromotion *promotion = [[GAIEcommercePromotion alloc] init]; [promotion setId:@"PROMO_1234"]; [promotion setName:@"Summer Sale"]; [promotion setCreative:@"summer_banner2"]; [promotion setPosition:@"banner_slot1"]; GAIDictionaryBuilder *builder = [GAIDictionaryBuilder createEventWithCategory:@"Internal Promotions" action:@"click" label:@"Summer Sale" value:nil]; [builder set:kGAIPromotionClick forKey:kGAIPromotionAction]; [builder addPromotion:promotion]; [tracker send:[builder build]];