Java에서 Google API에 쉽게 액세스
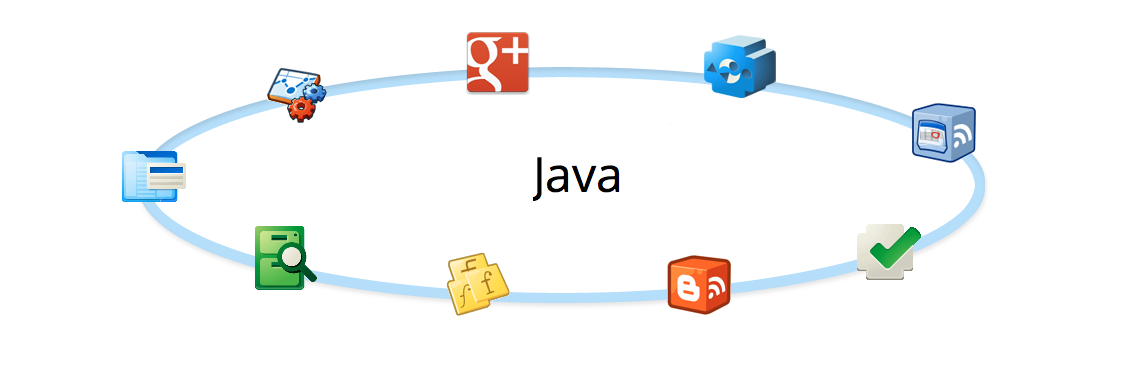
자바용 Google API 클라이언트 라이브러리는 모든 Google API에 공통적인 기능(예: HTTP 전송, 오류 처리, 인증, JSON 파싱, 미디어 다운로드/업로드, 일괄 처리)을 제공합니다. 라이브러리에는 일관된 인터페이스를 갖춘 강력한 OAuth 2.0 라이브러리, 모든 데이터 스키마를 지원하는 가볍고 효율적인 XML 및 JSON 데이터 모델, 프로토콜 버퍼가 지원됩니다.
자바용 Google 클라이언트 클라이언트를 사용하여 Google API를 호출하려면 액세스하는 Google API에 생성된 자바 라이브러리가 필요합니다. 생성된 라이브러리에는 핵심 google-api-java-client 라이브러리와 함께 루트 URL과 같은 API 관련 정보가 포함됩니다. 또한 API 컨텍스트에서 항목을 나타내고 JSON 객체와 자바 객체 간에 변환하는 데 유용한 클래스도 포함됩니다.
자바용 Google 클라이언트 클라이언트를 사용하여 Google API를 호출하려면 액세스하는 Google API에 생성된 자바 라이브러리가 필요합니다. 생성된 라이브러리에는 핵심 google-api-java-client 라이브러리와 함께 루트 URL과 같은 API 관련 정보가 포함됩니다. 또한 API 컨텍스트에서 항목을 나타내고 JSON 객체와 자바 객체 간에 변환하는 데 유용한 클래스도 포함됩니다.
Java용 Google API 클라이언트 라이브러리의 주요 사항
Google API를 호출하는 것은 간단합니다.
Java용 Google API 클라이언트 라이브러리와 함께 Google 서비스별로 생성된 라이브러리를 사용하여 Google API를 호출할 수 있습니다. Google API용으로 생성된 클라이언트 라이브러리를 찾으려면 지원되는 Google API 목록을 참고하세요. 다음은 Java용 Calendar API 클라이언트 라이브러리를 사용하여 Google Calendar API를 호출하는 예입니다.
// Show events on user's calendar. View.header("Show Calendars"); CalendarList feed = client.calendarList().list().execute(); View.display(feed);
라이브러리를 사용하면 더 쉽게 일괄 처리 및 미디어 업로드/다운로드를 할 수 있습니다.
라이브러리는 일괄 처리, 미디어 업로드, 미디어 다운로드를 위한 도우미 클래스를 제공합니다.라이브러리를 사용하면 인증이 더 쉬워집니다.
이 라이브러리에는 OAuth 2.0을 처리하는 데 필요한 코드의 양을 줄일 수 있는 강력한 인증 라이브러리가 포함되어 있습니다. 몇 줄만으로도 충분한 경우도 있습니다. 예:
/** Authorizes the installed application to access user's protected data. */ private static Credential authorize() throws Exception { // load client secrets GoogleClientSecrets clientSecrets = GoogleClientSecrets.load(JSON_FACTORY, new InputStreamReader(CalendarSample.class.getResourceAsStream("/client_secrets.json"))); // set up authorization code flow GoogleAuthorizationCodeFlow flow = new GoogleAuthorizationCodeFlow.Builder( httpTransport, JSON_FACTORY, clientSecrets, Collections.singleton(CalendarScopes.CALENDAR)).setDataStoreFactory(dataStoreFactory) .build(); // authorize return new AuthorizationCodeInstalledApp(flow, new LocalServerReceiver()).authorize("user"); }
라이브러리는 Google App Engine에서 실행됩니다.
App Engine용 도우미를 사용하면 인증된 API 호출을 빠르게 처리할 수 있으며 코드를 토큰으로 교환하는 데 대해 걱정할 필요가 없습니다.
예:
예:
@Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws IOException { AppIdentityCredential credential = new AppIdentityCredential(Arrays.asList(UrlshortenerScopes.URLSHORTENER)); Urlshortener shortener = new Urlshortener.Builder(new UrlFetchTransport(), new JacksonFactory(), credential) .build(); UrlHistory history = shortener.URL().list().execute(); ... }
라이브러리는 Android 4.4 이상에서 실행됩니다.
Java용 Google 클라이언트 라이브러리의 Android용 도우미 클래스는 Android AccountManager와 잘 통합되어 있습니다. 예를 들면 다음과 같습니다.
@Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // Google Accounts credential = GoogleAccountCredential.usingOAuth2(this, Collections.singleton(TasksScopes.TASKS)); SharedPreferences settings = getPreferences(Context.MODE_PRIVATE); credential.setSelectedAccountName(settings.getString(PREF_ACCOUNT_NAME, null)); // Tasks client service = new com.google.api.services.tasks.Tasks.Builder(httpTransport, jsonFactory, credential) .setApplicationName("Google-TasksAndroidSample/1.0").build(); }
간단한 설치
생성된 라이브러리를 사용하지 않는 경우 다운로드 페이지에서 Java용 Google API 클라이언트 라이브러리의 바이너리를 직접 다운로드하거나 Maven 또는 Gradle을 사용할 수 있습니다. Maven을 사용하려면 pom.xml 파일에 다음 줄을 추가합니다.
Gradle을 사용하려면 build.gradle 파일에 다음 줄을 추가합니다.
<project> <dependencies> <dependency> <groupId>com.google.api-client</groupId> <artifactId>google-api-client</artifactId> <version>1.32.1</version> </dependency> </dependencies> </project>
Gradle을 사용하려면 build.gradle 파일에 다음 줄을 추가합니다.
repositories { mavenCentral() } dependencies { compile 'com.google.api-client:google-api-client:1.32.1' }Java용 Google API 클라이언트 라이브러리 설치 및 설정에 관한 자세한 내용은 다운로드 및 설정 안내를 참고하세요.
지원되는 환경
Java용 Google API 클라이언트 라이브러리는 다음과 같은 Java 환경을 지원합니다.
- 자바 7 이상, 표준 (SE), 엔터프라이즈 (EE)
- Google App Engine
- Android 4.4 이상 — 필요한 Google 서비스에서 Google Play 서비스 라이브러리를 사용할 수 있는 경우 이 라이브러리 대신 해당 라이브러리를 사용하세요. Google Play 라이브러리는 최상의 성능과 환경을 제공합니다.